


How to Execute Background Tasks in WPF Applications Without Freezing the UI?
Execute Background Tasks in WPF Applications
In WPF applications, it's often necessary to perform tasks in the background to avoid freezing the user interface (UI). To do this, developers need a mechanism that meets the following criteria:
- Non-blocking UI thread
- Progress reporting
- Cancellation
- Multithreading support
Recommended Approach: Task-based API and Async/Await
With the release of .NET 4.5 (or .NET 4.0 with the Microsoft.Bcl.Async library), the recommended approach for background tasks is to utilize the Task-based API and async/await. This technique offers the following advantages:
- Pseudo-sequential code workflow: Developers can write code that reads like a sequential workflow, making it easier to understand and maintain.
- Structured exception handling: Async/await provides a structured way to handle asynchronous exceptions.
Example Implementation
The following code demonstrates how to execute a background task using the Task-based API and async/await:
private async void Start(object sender, RoutedEventArgs e) { try { await Task.Run(() => { int progress = 0; for (; ; ) { System.Threading.Thread.Sleep(1); progress++; Logger.Info(progress); } }); } catch (Exception ex) { MessageBox.Show(ex.Message); } }
This code spawns a new task in parallel to the UI thread, allowing the UI to remain responsive while the background task progresses. It also includes exception handling to display an error message if an exception occurs during task execution.
Additional Resources
For more information on executing background tasks in WPF with progress reporting and cancellation support, consider the following references:
- [How to execute task in the WPF background while able to provide report and allow cancellation?](https://stackoverflow.com/questions/12342842/how-to-execute-task-in-the-wpf-background-while-able-to-provide-report-a)
- [Async in 4.5: Enabling Progress and Cancellation in Async APIs](https://blogs.msdn.microsoft.com/charlie/2012/03/14/async-in-4-5-enabling-progress-and-cancellation-in-async-apis/)
- [Async and Await](https://docs.microsoft.com/en-us/dotnet/csharp/programming-guide/concepts/async/)
- [Async/Await FAQ](https://docs.microsoft.com/en-us/dotnet/csharp/programming-guide/concepts/async/async-faq)
The above is the detailed content of How to Execute Background Tasks in WPF Applications Without Freezing the UI?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










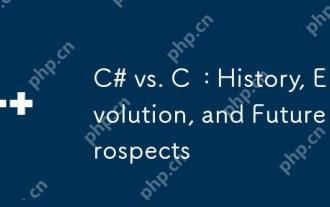
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
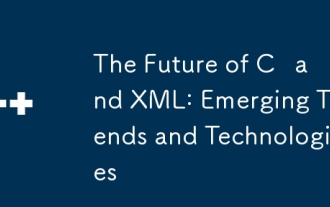
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
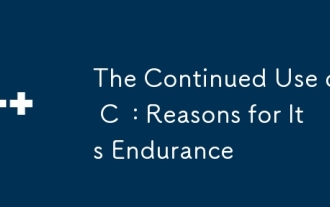
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
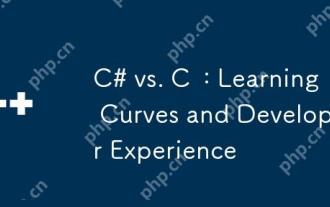
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
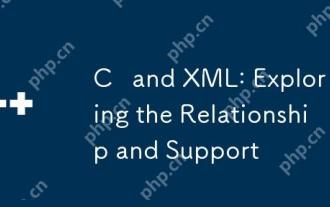
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
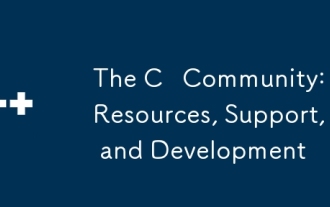
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
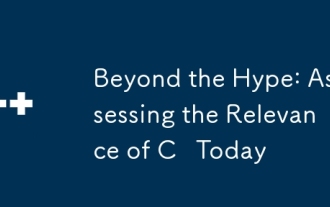
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
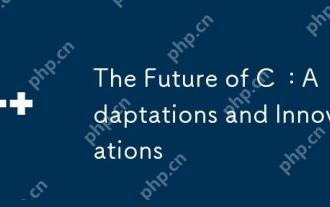
The future of C will focus on parallel computing, security, modularization and AI/machine learning: 1) Parallel computing will be enhanced through features such as coroutines; 2) Security will be improved through stricter type checking and memory management mechanisms; 3) Modulation will simplify code organization and compilation; 4) AI and machine learning will prompt C to adapt to new needs, such as numerical computing and GPU programming support.
