Flask Routes vs Flask-RESTful Routes
This article compares Flask and Flask-RESTful routes from a syntactic perspective. Both handle client-server communication using URLs, server resources, and HTTP methods, but their implementation differs significantly.
What are Routes?
Routes define communication channels between client and server, consisting of a URL path, a server resource, and HTTP methods.
URL Path:
Both route types use URL paths to specify the server address (e.g., '/home'). The key difference lies in how they're defined.
Flask Routes:
Flask uses decorators to bind functions to URL paths:
@app.route('/home')
The path is an argument to the route
method.
Flask-RESTful Routes:
Flask-RESTful uses add_resource
:
api.add_resource(Home, '/home')
The path is the second argument; the first is the server resource.
Server Resource:
This is where request processing happens. The core difference is in how Flask and Flask-RESTful structure this resource.
Flask Routes:
The server resource is a function:
def home(): return "Welcome to the homepage!"
Flask-RESTful Routes:
Flask-RESTful uses a class-based approach, inheriting from Resource
:
class Home(Resource): def get(self): return "Welcome to the homepage!"
HTTP Methods:
Both support HTTP methods (GET, POST, etc.) to specify request types. Again, the syntax differs.
Flask Routes:
HTTP methods are specified within the route
decorator:
@app.route('/home', methods=['GET'])
Flask-RESTful Routes:
Methods are defined as methods within the server resource class:
def get(self):
Putting it Together:
Flask Route Example:
@app.route('/home', methods=['GET']) def home(): return "Welcome to the homepage!"
Flask-RESTful Route Example:
class Home(Resource): def get(self): return "Welcome to the homepage!" api.add_resource(Home, '/home')
Conclusion:
Both Flask and Flask-RESTful are valid choices for client-server communication. However, Flask routes don't inherently follow REST principles, while Flask-RESTful does. Other key differences, including configuration and overall architecture, are not covered here. Further research into REST principles and the specific frameworks is recommended.
For deeper understanding, refer to resources on APIs, REST principles, Flask setup, Flask-RESTful setup, and HTTP methods.
The above is the detailed content of Flask Routes vs Flask-RESTful Routes. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










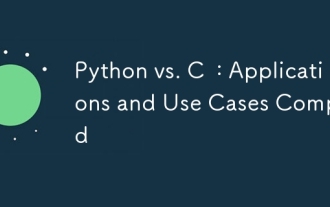
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
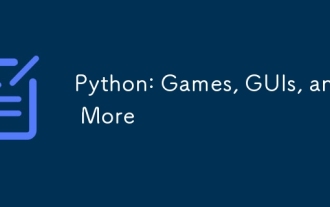
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
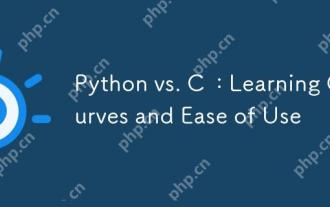
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
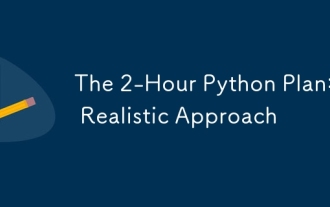
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
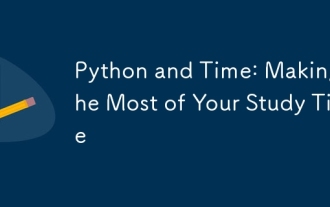
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
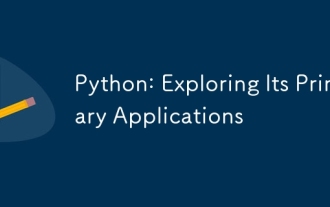
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
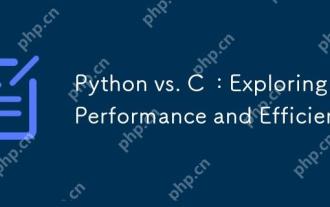
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
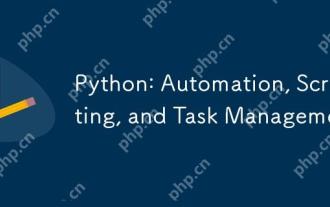
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
