Using CSRF Protection with Django and AJAX Requests
Securing Django AJAX Requests with CSRF Protection
Django's built-in CSRF (Cross-Site Request Forgery) protection, enabled by default when creating a project with django-admin startproject
, utilizes a CSRF token to safeguard against malicious requests. This middleware is added to your settings.py
.
Every POST request to your Django application requires a valid CSRF token. In Django templates, this is achieved by including {% csrf_token %}
within any form using the POST method. However, handling CSRF protection with separate front-end AJAX requests requires a different approach.
This tutorial demonstrates securing a simple Django application with AJAX requests from a separate front-end.
Application Setup
Our example application features two endpoints:
-
GET
/get-picture
: Retrieves the URL of an image stored on the server. -
POST
/set-picture
: Updates the URL of the image stored on the server.
For simplicity, error handling is omitted. The initial backend code (in urls.py
) is as follows:
from django.urls import path from django.http import JsonResponse import json picture_url = "https://picsum.photos/id/247/720/405" def get_picture(request): return JsonResponse({"picture_url": picture_url}) def set_picture(request): if request.method == "POST": global picture_url picture_url = json.loads(request.body)["picture_url"] return JsonResponse({"picture_url": picture_url}) urlpatterns = [ path("get-picture", get_picture), path("set-picture", set_picture) ]
The corresponding front-end functions (simplified):
// GET request to retrieve the image URL async function get_picture() { const res = await fetch("http://localhost:8000/get-picture"); const data = await res.json(); return data.picture_url; } // POST request to update the image URL async function set_picture(picture_url) { const res = await fetch("http://localhost:8000/set-picture", { method: "POST", body: JSON.stringify({ "picture_url": picture_url }) }); }
To handle Cross-Origin Resource Sharing (CORS), we'll use the django-cors-headers
package.
Enabling CORS and CSRF Protection
Install django-cors-headers
:
pip install django-cors-headers
Configure settings.py
:
INSTALLED_APPS = [ "corsheaders", # ... other apps ] MIDDLEWARE = [ "corsheaders.middleware.CorsMiddleware", # ... other middleware ] CORS_ALLOWED_ORIGINS = ["http://localhost:4040"] # Adjust port as needed CSRF_TRUSTED_ORIGINS = ["http://localhost:4040"] # Add your frontend origin
While GET requests will now function correctly, POST requests will fail due to CSRF protection. To resolve this, we need to manage CSRF tokens manually.
Fetching and Using the CSRF Token
Create a new view to serve the CSRF token:
from django.views.decorators.csrf import ensure_csrf_cookie from django.http import JsonResponse @ensure_csrf_cookie def get_csrf_token(request): return JsonResponse({"success": True}) urlpatterns = [ # ... other paths path("get-csrf-token", get_csrf_token), ]
Update the front-end to fetch the token (using js-cookie
):
fetch("http://localhost:8000/get-csrf-token", { credentials: "include" });
The credentials: "include"
option ensures the browser handles any Set-Cookie
headers, storing the csrftoken
cookie. Inspect the network tab in your browser's developer tools to verify the cookie is set.
Modifying the POST Request
Finally, modify the set_picture
function to include the CSRF token in the header:
async function set_picture(picture_url) { const res = await fetch("http://localhost:8000/set-picture", { method: "POST", credentials: "include", headers: { 'X-CSRFToken': Cookies.get("csrftoken") }, body: JSON.stringify({ "picture_url": picture_url }) }); }
This adds the X-CSRFToken
header with the value from the csrftoken
cookie, enabling successful POST requests.
Important Considerations
This approach has limitations, especially when deploying the front-end and back-end on different domains. Browser security policies may prevent the setting or accessing of third-party cookies, impacting CSRF token management.
Resources
- Django CSRF Documentation
- Django CSRF Reference
- CORS Documentation
- django-cors-headers
- Django CSRF_TRUSTED_ORIGINS
- Source Code
The above is the detailed content of Using CSRF Protection with Django and AJAX Requests. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


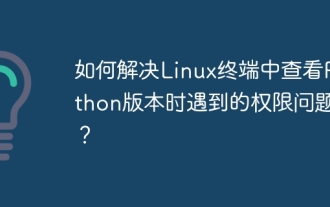
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
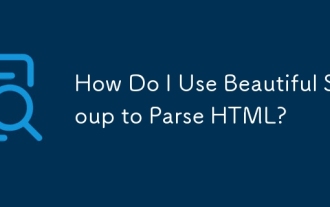
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
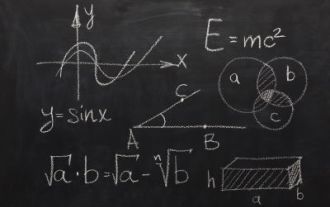
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
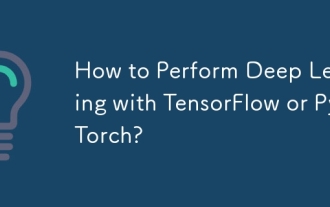
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
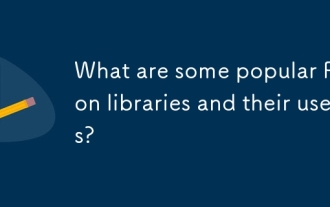
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
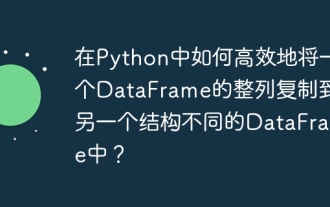
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
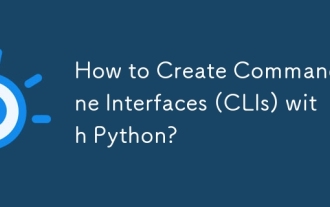
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.
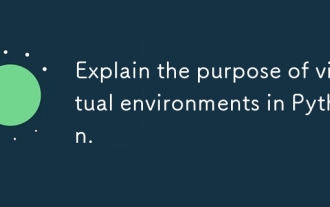
The article discusses the role of virtual environments in Python, focusing on managing project dependencies and avoiding conflicts. It details their creation, activation, and benefits in improving project management and reducing dependency issues.
