Event-Driven Architecture
Event-Driven Architecture (AOE) is a design model used to develop systems that react to events in real time. This type of architecture is very useful in scenarios where it is necessary to process information quickly, such as in e-commerce and banking applications.
What is an event?
An event is any action or change that occurs in a system. For example, when a customer makes a purchase on a website, this can be considered an event. Other examples include button clicks or data updates.
How does AOE work?
The architecture works based on three main components:
Event producers: These are the parts of the system that generate events.
Event consumers: They are responsible for receiving and reacting to events.
Event broker: An intermediary who distributes events from producers to consumers.
When an event occurs, it is captured and sent to a broker, who delivers it to services or systems interested in that type of event.
Benefits of AOE
Scalability: Allows systems to grow efficiently, as events can be processed asynchronously.
Decoupling: Producers and consumers do not need to know each other's details, making the system more flexible.
Real-time responses: Ideal for applications that need to react quickly to changes.
A very simple example in Java OOP ☕:
// Definição do evento class PedidoCriadoEvent { private String idPedido; public PedidoCriadoEvent(String idPedido) { this.idPedido = idPedido; } public String getIdPedido() { return idPedido; } } // Interface para o consumidor (listener) interface PedidoCriadoListener { void onPedidoCriado(PedidoCriadoEvent event); } // Produtor do evento class SistemaDePedidos { private PedidoCriadoListener listener; public void registrarListener(PedidoCriadoListener listener) { this.listener = listener; } public void criarPedido(String idPedido) { System.out.println("Pedido criado com ID: " + idPedido); if (listener != null) { listener.onPedidoCriado(new PedidoCriadoEvent(idPedido)); } } } // Consumidor do evento class EnvioDeEmail implements PedidoCriadoListener { @Override public void onPedidoCriado(PedidoCriadoEvent event) { System.out.println("Enviando e-mail para o pedido: " + event.getIdPedido()); } } // Simulação public class Main { public static void main(String[] args) { SistemaDePedidos sistema = new SistemaDePedidos(); EnvioDeEmail envioDeEmail = new EnvioDeEmail(); sistema.registrarListener(envioDeEmail); sistema.criarPedido("12345"); } }
What happens in this example?
Producer: The OrderSystem class creates an order and notifies interested parties.
Consumer: The EmailSend class reacts to the event, sending an email to the created order.
Decoupling: TheOrderSystem does not know what the consumer will do with the event, it just issues it.
Yes, as I said, it was a very simple example, simulating communication between classes using Listeners.
Thanks
The above is the detailed content of Event-Driven Architecture. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










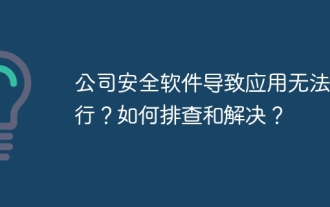
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
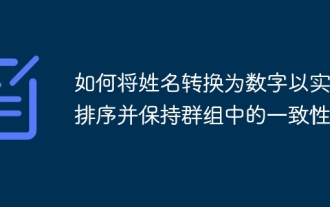
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
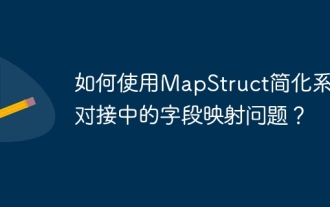
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
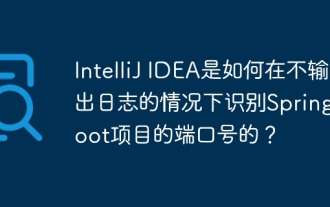
Start Spring using IntelliJIDEAUltimate version...
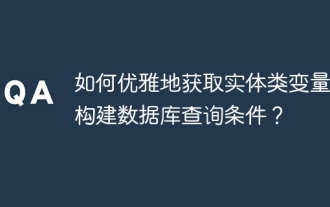
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
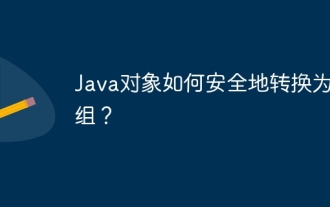
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
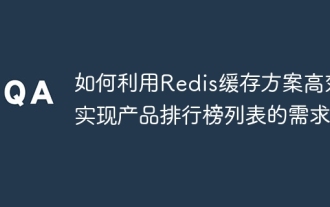
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
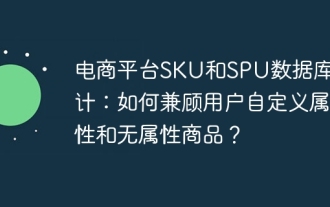
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
