String Matching in an Array
1408. String Matching in an Array
Difficulty: Easy
Topics: Array, String, String Matching
Given an array of string words, return all strings in words that is a substring of another word. You can return the answer in any order.
A substring is a contiguous sequence of characters within a string
Example 1:
- Input: words = ["mass","as","hero","superhero"]
- Output: ["as","hero"]
- Explanation: "as" is substring of "mass" and "hero" is substring of "superhero". ["hero","as"] is also a valid answer.
Example 2:
- Input: words = ["leetcode","et","code"]
- Output: ["et","code"]
- Explanation: "et", "code" are substring of "leetcode".
Example 3:
- Input: words = ["blue","green","bu"]
- Output: []
- Explanation: No string of words is substring of another string.
Constraints:
- 1 <= words.length <= 100
- 1 <= words[i].length <= 30
- words[i] contains only lowercase English letters.
- All the strings of words are unique.
Hint:
- Bruteforce to find if one string is substring of another or use KMP algorithm.
Solution:
We need to find all strings in the words array that are substrings of another word in the array, you can use a brute force approach. The approach involves checking each string in the list and verifying if it's a substring of any other string.
Let's implement this solution in PHP: 1408. String Matching in an Array
<?php /** * @param String[] $words * @return String[] */ function stringMatching($words) { ... ... ... /** * go to ./solution.php */ } // Example 1 $words = ["mass", "as", "hero", "superhero"]; print_r(stringMatching($words)); // Example 2 $words = ["leetcode", "et", "code"]; print_r(stringMatching($words)); // Example 3 $words = ["blue", "green", "bu"]; print_r(stringMatching($words)); ?> <h3> Explanation: </h3> <ol> <li>The function stringMatching loops through all the words in the input array.</li> <li>For each word, it compares it with every other word in the array using a nested loop.</li> <li>It uses PHP's strpos() function to check if one string is a substring of another. The strpos() function returns false if the substring is not found.</li> <li>If a substring is found, we add the word to the result array and break out of the inner loop, as we only need to record the word once.</li> <li>Finally, the function returns the result array containing all the substrings.</li> </ol> <h3> Time Complexity: </h3> <ul> <li>The time complexity is <em><strong>O(n<sup>2</sup> x m)</strong></em>, where <em><strong>n</strong></em> is the number of words and <em><strong>m</strong></em> is the maximum length of a word. This is because we are performing a substring search for each word within every other word.</li> </ul> <h3> Example Outputs: </h3> <p>For input ["mass", "as", "hero", "superhero"], the output will be:<br> </p> <pre class="brush:php;toolbar:false">Array ( [0] => as [1] => hero )
For input ["leetcode", "et", "code"], the output will be:
Array ( [0] => et [1] => code )
For input ["blue", "green", "bu"], the output will be:
Array ( )
This solution works well for the given problem constraints.
Contact Links
If you found this series helpful, please consider giving the repository a star on GitHub or sharing the post on your favorite social networks ?. Your support would mean a lot to me!
If you want more helpful content like this, feel free to follow me:
- GitHub
The above is the detailed content of String Matching in an Array. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


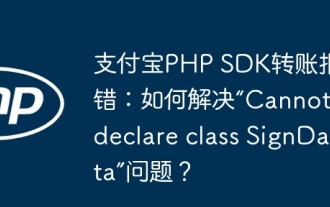
Alipay PHP...
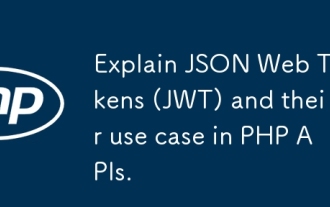
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
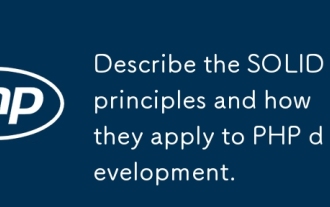
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
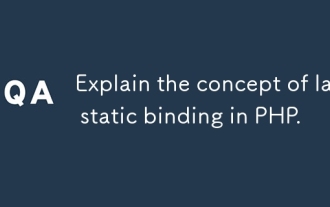
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
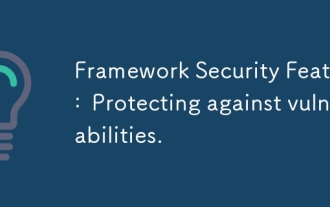
Article discusses essential security features in frameworks to protect against vulnerabilities, including input validation, authentication, and regular updates.
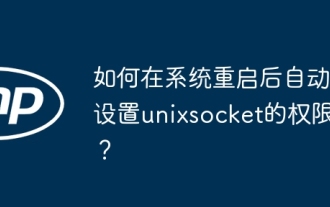
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
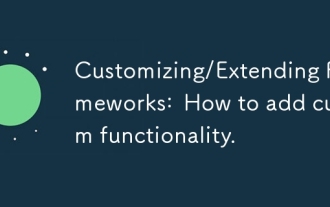
The article discusses adding custom functionality to frameworks, focusing on understanding architecture, identifying extension points, and best practices for integration and debugging.
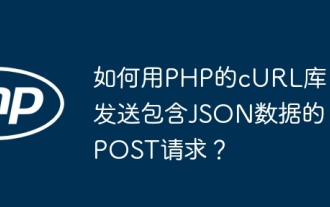
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
