


How to Calculate Date Differences Between Consecutive Rows for a Specific Account Number in SQL?
Efficiently Calculating Date Differences Between Consecutive Rows in SQL for a Specific Account
This article demonstrates two SQL queries to calculate the date difference between consecutive rows for a given account number. The methods presented offer varying degrees of efficiency.
Method 1: Using a LEFT JOIN
and MIN()
This approach employs a LEFT JOIN
to compare each row with subsequent rows for the same account. The MIN()
function finds the next date.
SELECT T1.ID, T1.AccountNumber, T1.Date, MIN(T2.Date) AS Date2, DATEDIFF("D", T1.Date, MIN(T2.Date)) AS DaysDiff FROM YourTable T1 LEFT JOIN YourTable T2 ON T1.AccountNumber = T2.AccountNumber AND T2.Date > T1.Date GROUP BY T1.ID, T1.AccountNumber, T1.Date;
This query joins the table (YourTable
) to itself, comparing each row (T1
) with all following rows for the same account number (T2
). The MIN(T2.Date)
finds the earliest subsequent date, and DATEDIFF
computes the difference in days. Grouping ensures a result for each original row.
Method 2: Using a Subquery for Improved Efficiency
For larger datasets, a subquery approach often proves more efficient. This method directly incorporates the date calculation within the main query.
SELECT ID, AccountNumber, Date, NextDate, DATEDIFF("D", Date, NextDate) AS DaysDiff FROM ( SELECT ID, AccountNumber, Date, ( SELECT MIN(Date) FROM YourTable T2 WHERE T2.AccountNumber = T1.AccountNumber AND T2.Date > T1.Date ) AS NextDate FROM YourTable T1 ) AS T
Here, a subquery is nested to find the NextDate
for each row. This avoids the potentially less efficient JOIN
operation. The outer query then calculates the DaysDiff
using DATEDIFF
.
Both methods achieve the same result: calculating the date difference between consecutive records for each account number. However, for optimal performance with large tables, Method 2 (using the subquery) is generally recommended. Choose the method best suited to your data volume and database system.
The above is the detailed content of How to Calculate Date Differences Between Consecutive Rows for a Specific Account Number in SQL?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










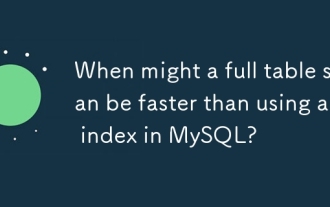
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
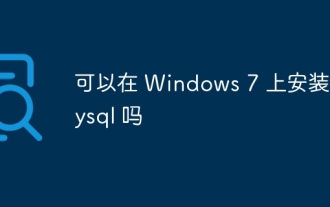
Yes, MySQL can be installed on Windows 7, and although Microsoft has stopped supporting Windows 7, MySQL is still compatible with it. However, the following points should be noted during the installation process: Download the MySQL installer for Windows. Select the appropriate version of MySQL (community or enterprise). Select the appropriate installation directory and character set during the installation process. Set the root user password and keep it properly. Connect to the database for testing. Note the compatibility and security issues on Windows 7, and it is recommended to upgrade to a supported operating system.
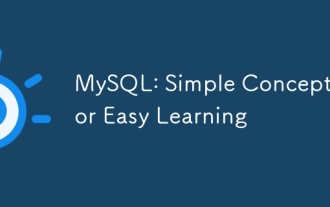
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
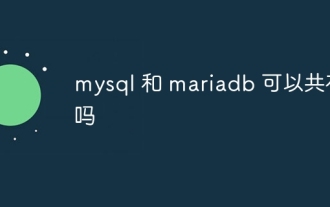
MySQL and MariaDB can coexist, but need to be configured with caution. The key is to allocate different port numbers and data directories to each database, and adjust parameters such as memory allocation and cache size. Connection pooling, application configuration, and version differences also need to be considered and need to be carefully tested and planned to avoid pitfalls. Running two databases simultaneously can cause performance problems in situations where resources are limited.
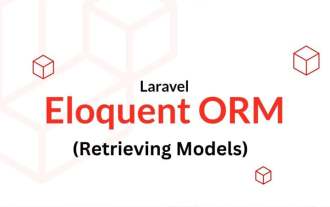
LaravelEloquent Model Retrieval: Easily obtaining database data EloquentORM provides a concise and easy-to-understand way to operate the database. This article will introduce various Eloquent model search techniques in detail to help you obtain data from the database efficiently. 1. Get all records. Use the all() method to get all records in the database table: useApp\Models\Post;$posts=Post::all(); This will return a collection. You can access data using foreach loop or other collection methods: foreach($postsas$post){echo$post->
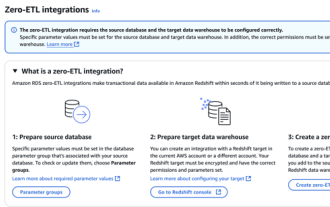
Data Integration Simplification: AmazonRDSMySQL and Redshift's zero ETL integration Efficient data integration is at the heart of a data-driven organization. Traditional ETL (extract, convert, load) processes are complex and time-consuming, especially when integrating databases (such as AmazonRDSMySQL) with data warehouses (such as Redshift). However, AWS provides zero ETL integration solutions that have completely changed this situation, providing a simplified, near-real-time solution for data migration from RDSMySQL to Redshift. This article will dive into RDSMySQL zero ETL integration with Redshift, explaining how it works and the advantages it brings to data engineers and developers.
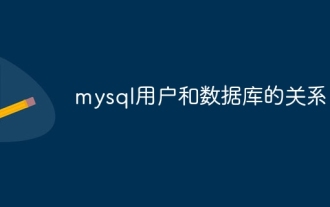
In MySQL database, the relationship between the user and the database is defined by permissions and tables. The user has a username and password to access the database. Permissions are granted through the GRANT command, while the table is created by the CREATE TABLE command. To establish a relationship between a user and a database, you need to create a database, create a user, and then grant permissions.
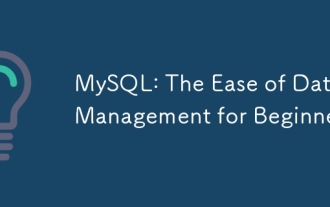
MySQL is suitable for beginners because it is simple to install, powerful and easy to manage data. 1. Simple installation and configuration, suitable for a variety of operating systems. 2. Support basic operations such as creating databases and tables, inserting, querying, updating and deleting data. 3. Provide advanced functions such as JOIN operations and subqueries. 4. Performance can be improved through indexing, query optimization and table partitioning. 5. Support backup, recovery and security measures to ensure data security and consistency.
