How to Calculate Date Differences Between Consecutive Rows in SQL?
SQL consecutive row date difference calculation
Determining the time interval between consecutive records is crucial for data analysis when working with large data sets. In this example, a SQL query is needed to calculate the date difference between consecutive rows with the same account number.
The following is a possible query:
SELECT T1.ID, T1.AccountNumber, T1.Date, MIN(T2.Date) AS NextDate, DATEDIFF(day, T1.Date, MIN(T2.Date)) AS DaysDiff FROM YourTable T1 LEFT JOIN YourTable T2 ON T1.AccountNumber = T2.AccountNumber AND T2.Date > T1.Date GROUP BY T1.ID, T1.AccountNumber, T1.Date;
This query uses LEFT JOIN
to compare each row in table T1
(the original table) to all subsequent rows in table T2
that have the same account number. It then uses the DATEDIFF
function to calculate the date difference and groups the results by ID
, AccountNumber
, and Date
to remove duplicate rows. DATEDIFF(day, ...)
Specifies the number of days to calculate the difference.
Another method is as follows:
SELECT ID, AccountNumber, Date, LEAD(Date, 1, NULL) OVER (PARTITION BY AccountNumber ORDER BY Date) AS NextDate, DATEDIFF(day, Date, LEAD(Date, 1, NULL) OVER (PARTITION BY AccountNumber ORDER BY Date)) AS DaysDiff FROM YourTable;
This query uses window functions LEAD
to get the next row date for each account number. LEAD(Date, 1, NULL) OVER (PARTITION BY AccountNumber ORDER BY Date)
Get the date of the next row of the current row, or NULL if there is no next row. Then calculate the date difference and present the result in the required format. This method is generally more efficient than LEFT JOIN
, especially on large datasets.
Both methods can calculate the date difference of consecutive rows. Which method to choose depends on your database system and performance requirements. The second method (using LEAD
) is usually more concise and efficient.
The above is the detailed content of How to Calculate Date Differences Between Consecutive Rows in SQL?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










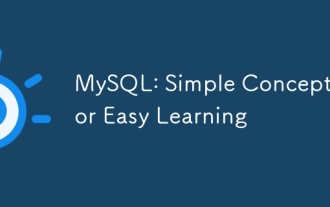
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
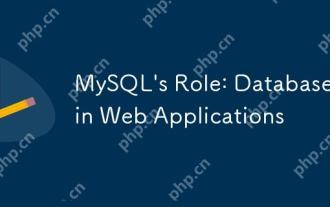
The main role of MySQL in web applications is to store and manage data. 1.MySQL efficiently processes user information, product catalogs, transaction records and other data. 2. Through SQL query, developers can extract information from the database to generate dynamic content. 3.MySQL works based on the client-server model to ensure acceptable query speed.
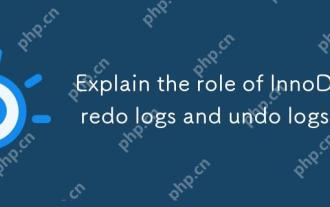
InnoDB uses redologs and undologs to ensure data consistency and reliability. 1.redologs record data page modification to ensure crash recovery and transaction persistence. 2.undologs records the original data value and supports transaction rollback and MVCC.
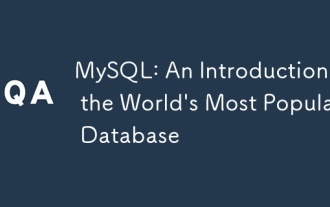
MySQL is an open source relational database management system, mainly used to store and retrieve data quickly and reliably. Its working principle includes client requests, query resolution, execution of queries and return results. Examples of usage include creating tables, inserting and querying data, and advanced features such as JOIN operations. Common errors involve SQL syntax, data types, and permissions, and optimization suggestions include the use of indexes, optimized queries, and partitioning of tables.
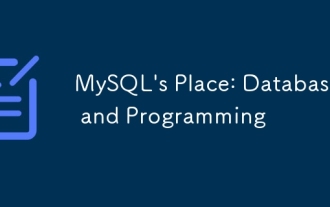
MySQL's position in databases and programming is very important. It is an open source relational database management system that is widely used in various application scenarios. 1) MySQL provides efficient data storage, organization and retrieval functions, supporting Web, mobile and enterprise-level systems. 2) It uses a client-server architecture, supports multiple storage engines and index optimization. 3) Basic usages include creating tables and inserting data, and advanced usages involve multi-table JOINs and complex queries. 4) Frequently asked questions such as SQL syntax errors and performance issues can be debugged through the EXPLAIN command and slow query log. 5) Performance optimization methods include rational use of indexes, optimized query and use of caches. Best practices include using transactions and PreparedStatemen
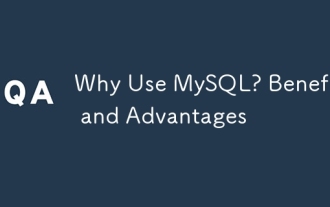
MySQL is chosen for its performance, reliability, ease of use, and community support. 1.MySQL provides efficient data storage and retrieval functions, supporting multiple data types and advanced query operations. 2. Adopt client-server architecture and multiple storage engines to support transaction and query optimization. 3. Easy to use, supports a variety of operating systems and programming languages. 4. Have strong community support and provide rich resources and solutions.
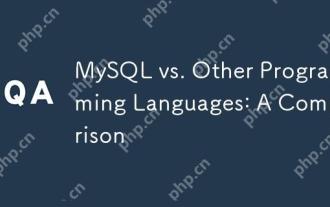
Compared with other programming languages, MySQL is mainly used to store and manage data, while other languages such as Python, Java, and C are used for logical processing and application development. MySQL is known for its high performance, scalability and cross-platform support, suitable for data management needs, while other languages have advantages in their respective fields such as data analytics, enterprise applications, and system programming.
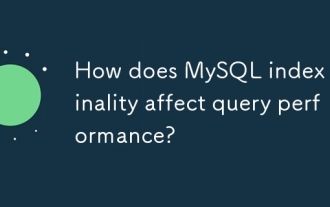
MySQL index cardinality has a significant impact on query performance: 1. High cardinality index can more effectively narrow the data range and improve query efficiency; 2. Low cardinality index may lead to full table scanning and reduce query performance; 3. In joint index, high cardinality sequences should be placed in front to optimize query.
