Laravel Eloquent ORM in Bangla Part-Models Retrieving)
Laravel Eloquent provides convenient and readable methods to interact with the database, making it easy to obtain database data. Several data acquisition techniques are introduced below:
1. Get all records
Use the all()
method to get all records in the table:
use App\Models\Post; $posts = Post::all();
This will return a Collection. You can access data using foreach
loops or other collection methods:
foreach ($posts as $post) { echo $post->title; }
2. Get a single record
1. find()
Method: Get a single record based on the primary key.
$post = Post::find(1); if ($post) { echo $post->title; }
2. findOrFail()
Method: Get a single record based on the primary key. If the record does not exist, a 404 exception will be thrown.
$post = Post::findOrFail(1);
3. first()
Method: Get the first record that meets the conditions.
$post = Post::where('status', 'published')->first();
4. firstOrFail()
Method: Get the first record that meets the conditions. If the record does not exist, a 404 exception will be thrown.
$post = Post::where('status', 'published')->firstOrFail();
3. Use query conditions to filter records
Use where
and other conditional statements to filter data.
1. where
Method:
$posts = Post::where('status', 'published')->get();
2. Multiple conditions:
$posts = Post::where('status', 'published') ->where('user_id', 1) ->get();
3. orWhere
Method:
$posts = Post::where('status', 'published') ->orWhere('status', 'draft') ->get();
4. Select specific fields
Eloquent gets all fields by default. Use the select()
method to select a specific field:
$posts = Post::select('title', 'content')->get();
5. Pagination
Use the paginate()
method to get data in pages:
$posts = Post::paginate(10);
Show pagination links in Blade templates:
{{ $posts->links() }}
6. Data Chunking
When processing large amounts of data, memory usage can be effectively reduced:
Post::chunk(100, function ($posts) { foreach ($posts as $post) { echo $post->title; } });
7. Sorting results (Ordering)
Use the orderBy()
method to sort in the specified order:
$posts = Post::orderBy('created_at', 'desc')->get();
8. Limit and Offset
Use take()
or limit()
and skip()
methods to limit the number of records fetched:
$posts = Post::take(5)->get(); // 获取前 5 条记录 $posts = Post::skip(10)->take(5)->get(); // 跳过前 10 条,获取接下来的 5 条
9. Aggregate functions (Aggregates)
1. Count:
$count = Post::count();
2. Maximum value:
$maxViews = Post::max('views');
3. Minimum value:
$minViews = Post::min('views');
4. Average:
$avgViews = Post::avg('views');
5. Sum:
$totalViews = Post::sum('views');
10. Customized relationship retrieval
Eloquent supports obtaining data from other models through relationships.
1. Eager Loading:
$posts = Post::with('comments')->get();
2. Specify relationship:
$posts = Post::with(['comments', 'user'])->get();
11. Raw Queries
Execute custom SQL queries using Laravel’s DB facade:
use App\Models\Post; $posts = Post::all();
These methods provide flexible data acquisition methods to meet various database operation needs. Please choose the appropriate method according to the actual situation.
The above is the detailed content of Laravel Eloquent ORM in Bangla Part-Models Retrieving). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










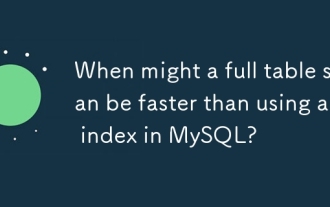
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
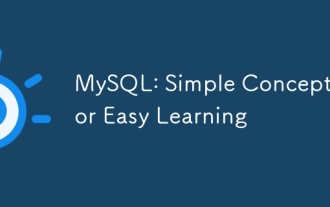
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
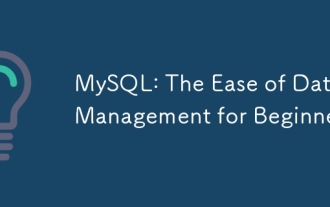
MySQL is suitable for beginners because it is simple to install, powerful and easy to manage data. 1. Simple installation and configuration, suitable for a variety of operating systems. 2. Support basic operations such as creating databases and tables, inserting, querying, updating and deleting data. 3. Provide advanced functions such as JOIN operations and subqueries. 4. Performance can be improved through indexing, query optimization and table partitioning. 5. Support backup, recovery and security measures to ensure data security and consistency.
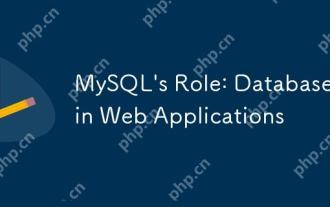
The main role of MySQL in web applications is to store and manage data. 1.MySQL efficiently processes user information, product catalogs, transaction records and other data. 2. Through SQL query, developers can extract information from the database to generate dynamic content. 3.MySQL works based on the client-server model to ensure acceptable query speed.
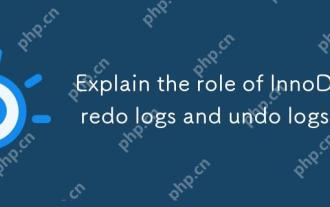
InnoDB uses redologs and undologs to ensure data consistency and reliability. 1.redologs record data page modification to ensure crash recovery and transaction persistence. 2.undologs records the original data value and supports transaction rollback and MVCC.
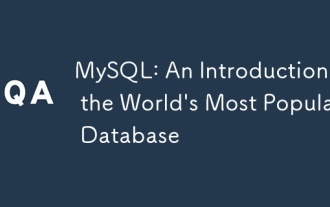
MySQL is an open source relational database management system, mainly used to store and retrieve data quickly and reliably. Its working principle includes client requests, query resolution, execution of queries and return results. Examples of usage include creating tables, inserting and querying data, and advanced features such as JOIN operations. Common errors involve SQL syntax, data types, and permissions, and optimization suggestions include the use of indexes, optimized queries, and partitioning of tables.
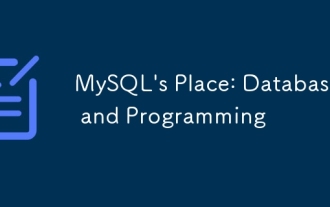
MySQL's position in databases and programming is very important. It is an open source relational database management system that is widely used in various application scenarios. 1) MySQL provides efficient data storage, organization and retrieval functions, supporting Web, mobile and enterprise-level systems. 2) It uses a client-server architecture, supports multiple storage engines and index optimization. 3) Basic usages include creating tables and inserting data, and advanced usages involve multi-table JOINs and complex queries. 4) Frequently asked questions such as SQL syntax errors and performance issues can be debugged through the EXPLAIN command and slow query log. 5) Performance optimization methods include rational use of indexes, optimized query and use of caches. Best practices include using transactions and PreparedStatemen
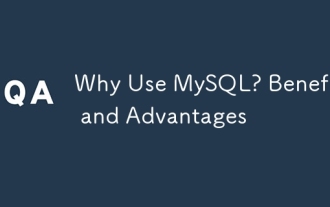
MySQL is chosen for its performance, reliability, ease of use, and community support. 1.MySQL provides efficient data storage and retrieval functions, supporting multiple data types and advanced query operations. 2. Adopt client-server architecture and multiple storage engines to support transaction and query optimization. 3. Easy to use, supports a variety of operating systems and programming languages. 4. Have strong community support and provide rich resources and solutions.
