How To Get an API Data and Store in AWS S3
Jan 08, 2025 pm 08:39 PMThis tutorial demonstrates how to retrieve data from the OpenWeather API using Python and store it in AWS S3. This straightforward method allows you to fetch and cloud-store API data for later use. Even if you're new to this, the steps are clearly outlined. For a different approach using React, see our article on fetching API data with React.
What You Will Learn:
This tutorial covers:
- Retrieving weather data from the OpenWeather API with Python.
- Setting up an S3 bucket for data storage.
- Uploading the fetched data to AWS S3.
Prerequisites:
Before starting, ensure you have:
- An AWS account (sign up here).
- A GitHub repository for your code (sign up here).
- A code editor (VS Code is recommended).
Step 1: Creating an AWS S3 Bucket
To store your data, create an S3 bucket:
- Log in to your AWS account.
- Search for "S3".
- Click "Create bucket" and follow the instructions.
- Choose a unique bucket name (e.g.,
my-weather-data
). - Select a region.
- Click "Create".
Step 2: Fetching Data from the OpenWeather API
Create an OpenWeather account.
Obtaining Your API Key:
- Sign Up: Register on the OpenWeather website. Your API key will be available on the next page.
- Locate Your API Key: The API key is usually found under an "API Key" tab.
- Alternative Access: You can also find it in your profile settings.
Install the requests
library:
pip install requests
Fetch weather data:
import requests import json api_key = 'YOUR_API_KEY' # Replace with your key city = 'London' def get_weather_data(): url = f'http://api.openweathermap.org/data/2.5/weather?q={city}&appid={api_key}' response = requests.get(url) return response.json() weather_data = get_weather_data() print(weather_data)
This script retrieves current weather data for the specified city.
Step 3: Setting Up the AWS SDK for Python (Boto3)
Install Boto3:
pip install boto3
Configure your AWS credentials following the configuration guide. You'll need your Access Key ID and Secret Access Key.
Step 4: Uploading Data to AWS S3
Set up the S3 client:
import boto3 aws_access_key_id = 'YOUR_ACCESS_KEY' # Replace aws_secret_access_key = 'YOUR_SECRET_KEY' # Replace region_name = 'eu-west-2' # Replace with your region s3 = boto3.client('s3', aws_access_key_id=aws_access_key_id, aws_secret_access_key=aws_secret_access_key, region_name=region_name)
Upload the data:
def upload_to_s3(data): bucket_name = 'my-weather-data' # Replace with your bucket name file_name = 'weather_data.json' s3.put_object(Bucket=bucket_name, Key=file_name, Body=json.dumps(data), ContentType='application/json') print('Upload successful!') upload_to_s3(weather_data)
How it Works:
The script uses requests
to fetch JSON data and boto3
to upload it to your S3 bucket as weather_data.json
.
Step 5: Verifying the Upload
Check your S3 Management Console to confirm the weather_data.json
file is in your bucket.
Conclusion:
This tutorial showed how to fetch and store weather data from OpenWeather API in AWS S3 using Python. This is a valuable technique for managing and accessing API data in the cloud.
The above is the detailed content of How To Get an API Data and Store in AWS S3. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
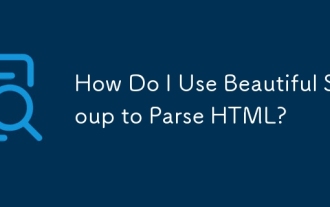
How Do I Use Beautiful Soup to Parse HTML?
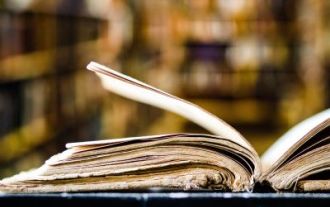
How to Use Python to Find the Zipf Distribution of a Text File
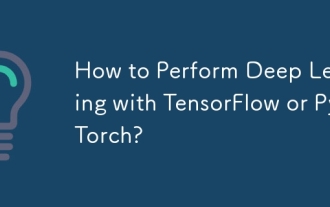
How to Perform Deep Learning with TensorFlow or PyTorch?
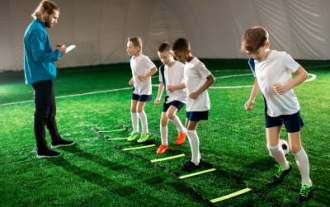
Introduction to Parallel and Concurrent Programming in Python
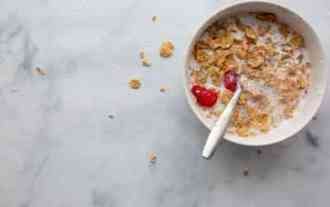
Serialization and Deserialization of Python Objects: Part 1
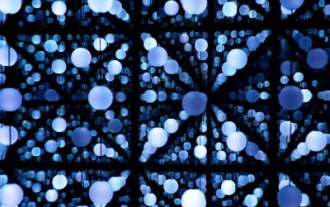
How to Implement Your Own Data Structure in Python
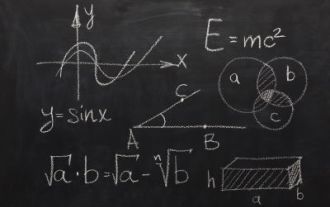
Mathematical Modules in Python: Statistics
