Introducing BlockBuster: is my asyncio event loop blocked?
Python 3.5 introduced asynchronous I/O as an alternative to threads to handle concurrency. The advantage of asynchronous I/O and the asyncio implementation in Python is that by not spawning memory-intensive operating system threads, the system uses fewer resources and is more scalable. Furthermore, in asyncio, scheduling points are clearly defined via the await
syntax, whereas in thread-based concurrency, the GIL may be released at unpredictable code points. As a result, asyncio-based concurrency systems are easier to understand and debug. Finally, the asyncio task can be canceled, which is not easy to do when using threads.
However, in order to truly benefit from these advantages, it is important to avoid blocking calls in async coroutines. Blocking calls can be network calls, file system calls, sleep
calls, etc. These blocking calls are harmful because, under the hood, asyncio uses a single-threaded event loop to run coroutines concurrently. So if you make a blocking call in a coroutine, it blocks the entire event loop and all coroutines, affecting the overall performance of your application.
The following is an example of a blocking call that prevents code from executing concurrently:
import asyncio import datetime import time async def example(name): print(f"{datetime.datetime.now()}: {name} start") time.sleep(1) # time.sleep 是一个阻塞函数 print(f"{datetime.datetime.now()}: {name} stop") async def main(): await asyncio.gather(example("1"), example("2")) asyncio.run(main())
The running result is similar to:
<code>2025-01-07 18:50:15.327677: 1 start 2025-01-07 18:50:16.328330: 1 stop 2025-01-07 18:50:16.328404: 2 start 2025-01-07 18:50:17.333159: 2 stop</code>
As you can see, the two coroutines are not running concurrently.
To overcome this problem you need to use a non-blocking equivalent or defer execution to the thread pool:
import asyncio import datetime import time async def example(name): print(f"{datetime.datetime.now()}: {name} start") await asyncio.sleep(1) # 将阻塞的 time.sleep 调用替换为非阻塞的 asyncio.sleep 协程 print(f"{datetime.datetime.now()}: {name} stop") async def main(): await asyncio.gather(example("1"), example("2")) asyncio.run(main())
The running result is similar to:
<code>2025-01-07 18:53:53.579738: 1 start 2025-01-07 18:53:53.579797: 2 start 2025-01-07 18:53:54.580463: 1 stop 2025-01-07 18:53:54.580572: 2 stop</code>
Here two coroutines run concurrently.
Now the problem is that it's not always easy to identify whether a method is blocking or not. Especially if the code base is large or uses third-party libraries. Sometimes, blocking calls are made in deep parts of the code.
For example, does this code block?
import blockbuster from importlib.metadata import version async def get_version(): return version("blockbuster")
Does Python load package metadata into memory on startup? Is it done when the blockbuster
module is loaded? Or when we call version()
? Are the results cached and will subsequent calls be non-blocking? The correct answer is done when calling version()
, which involves reading the installed package's METADATA file. And the results are not cached. Therefore, version()
is a blocking call and should always be deferred to the thread. It's hard to know this fact without digging into importlib
's code.
One way to detect blocking calls is to activate asyncio's debug mode to log blocking calls that take too long. But this is not the most efficient approach, as many blocking times shorter than the trigger timeout will still hurt performance, and blocking times in test/development may be different than in production. For example, database calls may take longer in a production environment if the database must fetch a large amount of data.
This is where BlockBuster comes in! When activated, BlockBuster will patch several blocking Python framework methods that will throw errors if they are called from the asyncio event loop. The default patching methods include methods of os
, io
, time
, socket
, and sqlite
modules. For a complete list of methods detected by BlockBuster, see the project readme. You can then activate BlockBuster in unit test or development mode to catch any blocking calls and fix them. If you know the awesome BlockHound library for the JVM, it's the same principle, but for Python. BlockHound was a great source of inspiration for BlockBuster, thanks to the creators.
Let’s see how to use BlockBuster on the above blocking code snippet.
First, we need to install the blockbuster
package
import asyncio import datetime import time async def example(name): print(f"{datetime.datetime.now()}: {name} start") time.sleep(1) # time.sleep 是一个阻塞函数 print(f"{datetime.datetime.now()}: {name} stop") async def main(): await asyncio.gather(example("1"), example("2")) asyncio.run(main())
We can then use the pytest fixture and the blockbuster_ctx()
method to activate the BlockBuster at the beginning of each test and deactivate it during teardown.
<code>2025-01-07 18:50:15.327677: 1 start 2025-01-07 18:50:16.328330: 1 stop 2025-01-07 18:50:16.328404: 2 start 2025-01-07 18:50:17.333159: 2 stop</code>
If you run this with pytest you will get
import asyncio import datetime import time async def example(name): print(f"{datetime.datetime.now()}: {name} start") await asyncio.sleep(1) # 将阻塞的 time.sleep 调用替换为非阻塞的 asyncio.sleep 协程 print(f"{datetime.datetime.now()}: {name} stop") async def main(): await asyncio.gather(example("1"), example("2")) asyncio.run(main())
Note: Typically, in a real project, the
blockbuster()
fixture will be set up in aconftest.py
file.
Conclusion
I believe BlockBuster is very useful in asyncio projects. It has helped me detect many blocking call issues in projects I've worked on. But it's not a panacea. In particular, some third-party libraries do not use Python framework methods to interact with the network or file system, but instead wrap C libraries. For these libraries, you can add rules in your test setup to trigger blocking calls to these libraries. BlockBuster is also open source: contributions are very welcome to add rules for your favorite libraries in the core project. If you see issues and areas for improvement, I'd love to receive your feedback in the project issue tracker.
Some links:
- GitHub Project
- Question
- Packages on Pypi
The above is the detailed content of Introducing BlockBuster: is my asyncio event loop blocked?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










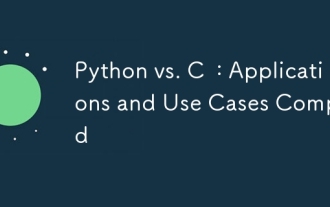
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
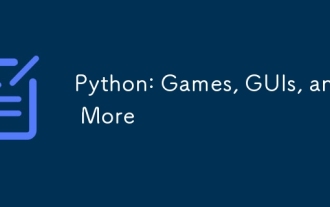
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
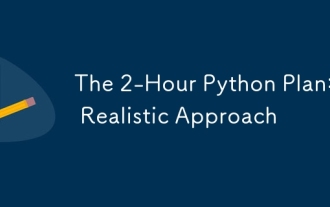
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
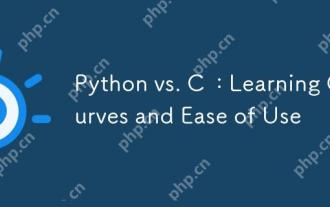
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
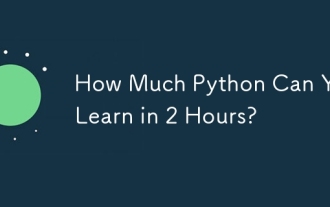
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
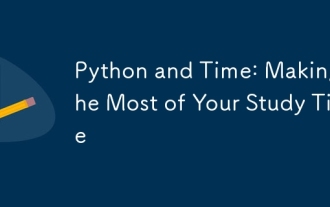
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
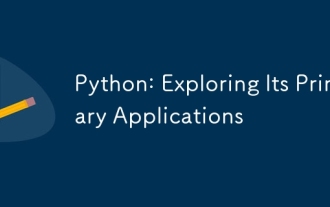
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
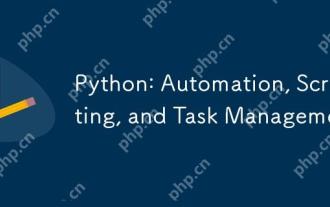
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
