Item Use lazy initialization sparingly
What is lazy initialization?
- Definition: Defer initialization of a field until it is accessed for the first time.
- Benefits: Avoids unnecessary initializations if the field is never used.
- Applications: Used for static and instance fields.
Best Practices and Examples
- Normal Boot (Preferred) Simple and effective.
Example:
private final FieldType field = computeFieldValue();
Use normal initialization for most fields, unless otherwise required.
- Lazy Initialization with Synchronized Getter When to use: To resolve startup circularities.
Example:
private FieldType field; synchronized FieldType getField() { if (field == null) { field = computeFieldValue(); } return field; }
3. Carrier Class Practice (For Static Fields)
- When to use: Efficient lazy initialization for static fields.
Example:
private static class FieldHolder { static final FieldType field = computeFieldValue(); } static FieldType getField() { return FieldHolder.field; }
Advantage: Initializes the class only when the field is accessed, with minimal cost after initialization.
4. Double Check Practice (For Instance Fields)
- When to use: For performance on lazy initialization on instance fields.
Example:
private volatile FieldType field; FieldType getField() { FieldType result = field; if (result == null) { // Primeira verificação (sem bloqueio) synchronized (this) { result = field; if (result == null) { // Segunda verificação (com bloqueio) field = result = computeFieldValue(); } } } return result; }
5. Single Check Practice (Repeated Initialization Allowed)
- When to use: Fields that can tolerate repeated initialization.
Example
private volatile FieldType field; FieldType getField() { if (field == null) { // Verificação única field = computeFieldValue(); } return field; }
6. Bold Single Check Practice
- When to use: Only if you tolerate extra initializations and if the field type is a primitive other than long or double.
Example:
private FieldType field; FieldType getField() { if (field == null) { // Sem volatile field = computeFieldValue(); } return field; }
General Considerations
Trade-offs:
- Lazy initialization minimizes initial cost but can increase field access cost.
- Evaluate with performance measurements.
Multithread Synchronization:
- Essential to avoid serious bugs.
- Use safe practices (e.g., volatile, locks).
Preferred Use:
- Static Fields: Carrier class practice.
- Instance Fields: Double Check.
- Repeated Startup Allowed: Single check.
Final Summary
- Boot normally whenever possible.
- Use lazy initialization only when necessary for performance or to resolve circularity issues
The above is the detailed content of Item Use lazy initialization sparingly. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










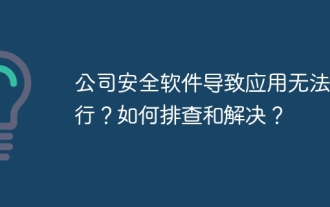
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
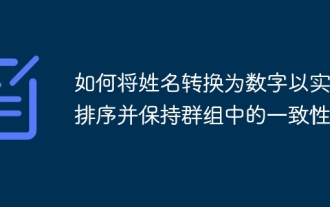
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
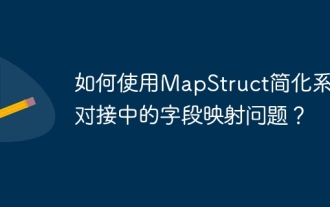
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
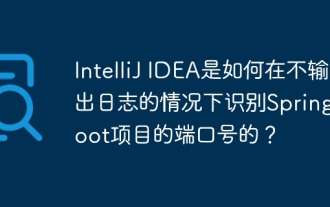
Start Spring using IntelliJIDEAUltimate version...
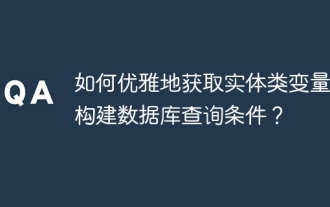
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
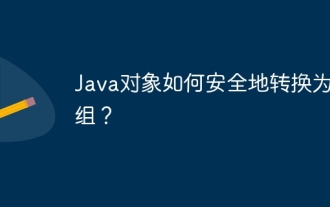
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
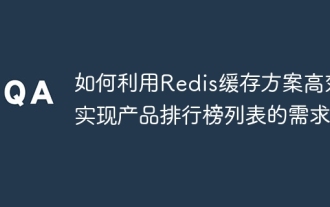
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
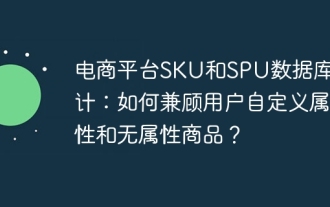
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
