How Do Parameterized Queries in SQL Prevent SQL Injection Attacks?
SQL parameterized query with question mark
When consulting the SQL documentation, you may encounter question marks (?) in queries. These placeholders represent parameterized queries and are widely used to execute dynamic SQL in programs.
Parameterized queries have many advantages. They simplify code by decoupling parameter values from the query itself, making it more efficient and flexible. Additionally, they enhance security by preventing SQL injection attacks.
For example, in a pseudocode example:
<code>ODBCCommand cmd = new ODBCCommand("SELECT thingA FROM tableA WHERE thingB = 7") result = cmd.Execute()</code>
can be rewritten as:
<code>ODBCCommand cmd = new ODBCCommand("SELECT thingA FROM tableA WHERE thingB = ?") cmd.Parameters.Add(7) result = cmd.Execute()</code>
This technique ensures proper string escaping, eliminating the risk of SQL injection. Consider the following scenario:
<code>string s = getStudentName() cmd.CommandText = "SELECT * FROM students WHERE (name = '" + s + "')" cmd.Execute()</code>
If the user enters the string Robert'); DROP TABLE students; --, a SQL injection attack may occur. However, using parameterized queries:
<code>s = getStudentName() cmd.CommandText = "SELECT * FROM students WHERE name = ?" cmd.Parameters.Add(s) cmd.Execute()</code>
The library function will clean the input to prevent malicious code execution.
Alternatively, Microsoft SQL Server uses named parameters, which improves readability and clarity:
<code>cmd.Text = "SELECT thingA FROM tableA WHERE thingB = @varname" cmd.Parameters.AddWithValue("@varname", 7) result = cmd.Execute()</code>
The above is the detailed content of How Do Parameterized Queries in SQL Prevent SQL Injection Attacks?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










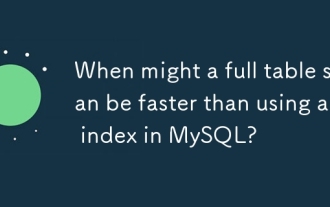
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
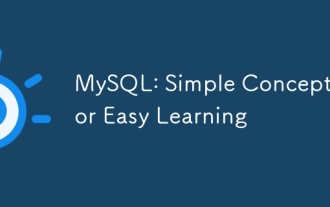
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
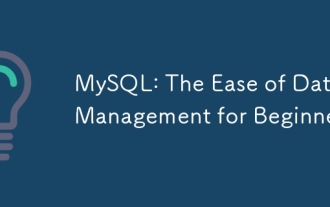
MySQL is suitable for beginners because it is simple to install, powerful and easy to manage data. 1. Simple installation and configuration, suitable for a variety of operating systems. 2. Support basic operations such as creating databases and tables, inserting, querying, updating and deleting data. 3. Provide advanced functions such as JOIN operations and subqueries. 4. Performance can be improved through indexing, query optimization and table partitioning. 5. Support backup, recovery and security measures to ensure data security and consistency.
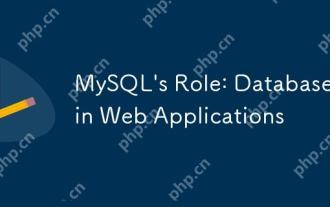
The main role of MySQL in web applications is to store and manage data. 1.MySQL efficiently processes user information, product catalogs, transaction records and other data. 2. Through SQL query, developers can extract information from the database to generate dynamic content. 3.MySQL works based on the client-server model to ensure acceptable query speed.
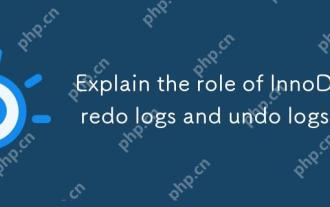
InnoDB uses redologs and undologs to ensure data consistency and reliability. 1.redologs record data page modification to ensure crash recovery and transaction persistence. 2.undologs records the original data value and supports transaction rollback and MVCC.
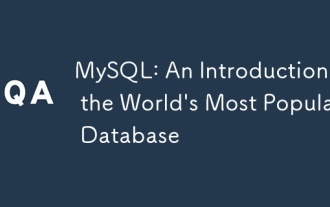
MySQL is an open source relational database management system, mainly used to store and retrieve data quickly and reliably. Its working principle includes client requests, query resolution, execution of queries and return results. Examples of usage include creating tables, inserting and querying data, and advanced features such as JOIN operations. Common errors involve SQL syntax, data types, and permissions, and optimization suggestions include the use of indexes, optimized queries, and partitioning of tables.
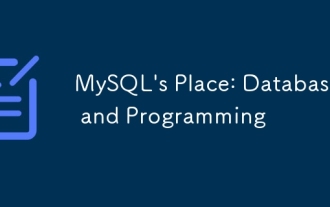
MySQL's position in databases and programming is very important. It is an open source relational database management system that is widely used in various application scenarios. 1) MySQL provides efficient data storage, organization and retrieval functions, supporting Web, mobile and enterprise-level systems. 2) It uses a client-server architecture, supports multiple storage engines and index optimization. 3) Basic usages include creating tables and inserting data, and advanced usages involve multi-table JOINs and complex queries. 4) Frequently asked questions such as SQL syntax errors and performance issues can be debugged through the EXPLAIN command and slow query log. 5) Performance optimization methods include rational use of indexes, optimized query and use of caches. Best practices include using transactions and PreparedStatemen
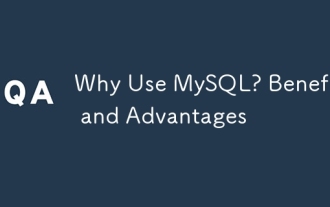
MySQL is chosen for its performance, reliability, ease of use, and community support. 1.MySQL provides efficient data storage and retrieval functions, supporting multiple data types and advanced query operations. 2. Adopt client-server architecture and multiple storage engines to support transaction and query optimization. 3. Easy to use, supports a variety of operating systems and programming languages. 4. Have strong community support and provide rich resources and solutions.
