


How to Map JSON Field Names to .NET Object Properties using JavaScriptSerializer (or Alternatives)?
Jan 10, 2025 am 06:50 AMUse JavaScriptSerializer.Deserialize: Map JSON field names to .NET object properties
Question:
How to map field names in JSON data to field names of a .NET object when using JavaScriptSerializer.Deserialize?
Answer:
The JavaScriptSerializer class does not provide direct field name mapping functionality. However, you can leverage the more flexible DataContractJsonSerializer class for this purpose.
To map field names:
- Add the DataContract attribute to your data object class:
1 2 3 4 |
|
- Use the DataMember attribute to specify the JSON property name corresponding to the object field:
1 2 3 4 5 |
|
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
|
Note:
- If you want to keep the DetailLevel field as an enumeration, you can use a custom JSON converter to handle conversion between string and enumeration values.
- DataContractJsonSerializer also supports field name mapping in Silverlight.
The above is the detailed content of How to Map JSON Field Names to .NET Object Properties using JavaScriptSerializer (or Alternatives)?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
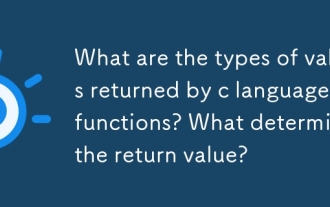
What are the types of values returned by c language functions? What determines the return value?
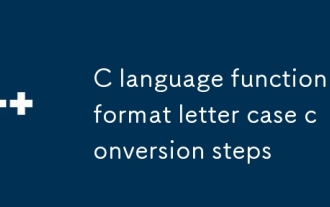
C language function format letter case conversion steps

What are the definitions and calling rules of c language functions and what are the

Where is the return value of the c language function stored in memory?
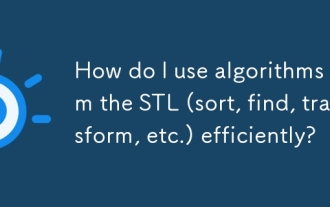
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
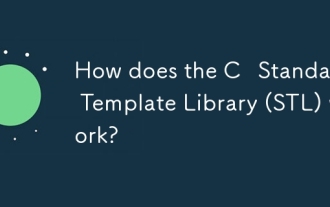
How does the C Standard Template Library (STL) work?
