How I write Go APIs in my experience with Fuego
My Experience Building Go APIs with Fuego
As a Go developer with several years of experience, I've explored various web frameworks. My journey included the standard library, Gin, and Fiber. While each has merits, I often found myself needing more structure or spending excessive time integrating multiple libraries for validation, serialization, and documentation. That's where Fuego changed the game.
Initially, Fuego seemed like just another framework. However, its use of modern Go features, particularly generics, to automatically generate OpenAPI specifications directly from code, intrigued me. I decided to test it on a small internal project, and here's my honest account.
First Impressions
Fuego's simplicity was immediately apparent. Setting up a basic server took mere minutes:
package main import "github.com/go-fuego/fuego" func main() { s := fuego.NewServer() fuego.Get(s, "/", func(c fuego.ContextNoBody) (string, error) { return "Hello, World!", nil }) s.Run() }
The familiarity was striking—similar to Gin, but with built-in OpenAPI support.
A Real-World Example
The "Hello World" example doesn't reflect real-world complexities. My application required JSON data handling, validation, and typed responses. Other frameworks necessitate custom JSON decoding, error handling, and middleware integration. Fuego streamlined this considerably using typed route handlers.
Here's a simplified route handler:
type UserInput struct { Name string `json:"name" validate:"required"` } type UserOutput struct { Message string `json:"message"` } func main() { s := fuego.NewServer() fuego.Post(s, "/user", handleUser) s.Run() } func handleUser(c fuego.ContextWithBody[UserInput]) (UserOutput, error) { in, err := c.Body() if err != nil { return UserOutput{}, err } return UserOutput{Message: "Hello, " + in.Name}, nil }
Key improvements:
- Typed Handlers:
fuego.ContextWithBody[UserInput]
automatically deserializes JSON into theUserInput
struct. - Validation:
validate:"required"
ensures theName
field is present; Fuego handles errors gracefully. - Responses: Returning a
UserOutput
struct automatically serializes it to JSON.
This eliminated significant boilerplate code—no json.Unmarshal
, external validation libraries, or custom error handling.
Why Fuego Stands Out
-
Native Go Feel: Unlike frameworks that heavily wrap
net/http
, Fuego feels remarkably native. It utilizesnet/http
directly, allowing seamless integration of standard middleware and handlers. I reused existing authentication middleware without issues. -
Automatic OpenAPI Generation: I previously managed separate YAML files or relied on comments for OpenAPI specs, a tedious and error-prone process. Fuego automatically generates the spec from route handler types, ensuring documentation always stays current.
-
Validation and Error Handling: The integrated validation (using
go-playground/validator
) was intuitive, and error handling was simplified. InvalidUserInput
structs resulted in structured error messages adhering to RFC standards.
Data Transformations
To ensure all incoming Name
fields were lowercase, I leveraged Fuego's InTransform
method:
package main import "github.com/go-fuego/fuego" func main() { s := fuego.NewServer() fuego.Get(s, "/", func(c fuego.ContextNoBody) (string, error) { return "Hello, World!", nil }) s.Run() }
This automatically transforms data before reaching the route handler.
Challenges Encountered
-
Smaller Ecosystem: Fuego's smaller user base compared to Gin or Echo resulted in fewer readily available community resources. However, the repository's examples and documentation proved sufficient.
-
Limited Built-in Middleware: While Fuego provides some middleware, it's not as extensive as some older frameworks.
net/http
compatibility allowed using external libraries or custom middleware.
Conclusion
Fuego offers a compelling balance of convenience and flexibility. It accelerates API development with built-in validation, serialization, and documentation generation, while remaining true to Go's principles. Using typed structs and letting Fuego manage the rest significantly improved my workflow.
Key benefits:
- Increased Productivity: Cleaner code and reduced boilerplate.
- Automated Documentation: Always up-to-date OpenAPI specifications.
-
Smooth Transitions: Easy integration with existing
net/http
handlers.
If you're seeking a modern, flexible Go framework, especially if you're weary of manual OpenAPI maintenance, I strongly recommend Fuego. It simplified my development process while staying true to Go's minimalist philosophy. The GitHub repository provides comprehensive information and a promising roadmap. I'm enthusiastic about its future and will continue using it for future projects.
The above is the detailed content of How I write Go APIs in my experience with Fuego. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
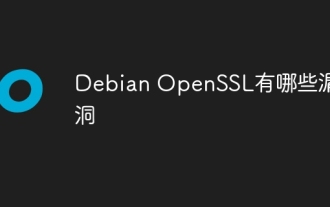
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
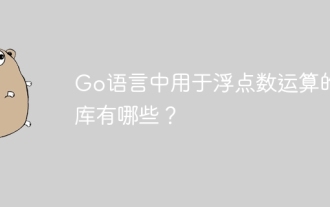
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
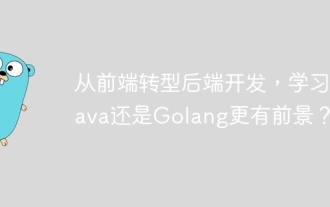
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
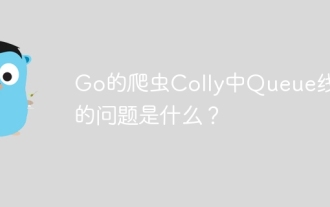
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
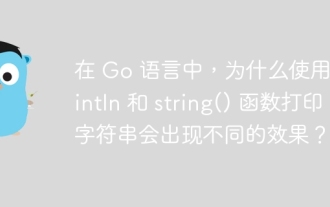
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
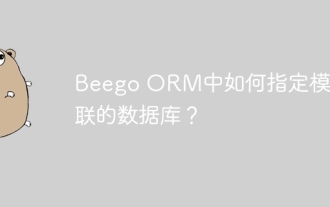
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
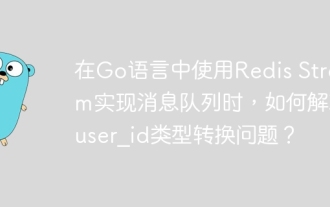
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
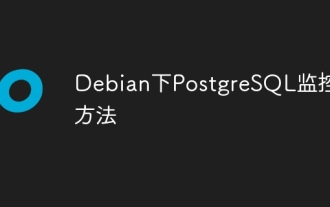
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
