How to Schedule a Daily Task in a C# Windows Service?
Jan 10, 2025 am 10:27 AMScheduling tasks in C# Windows Service
For situations where a task needs to be executed every midnight in a Windows service written in C#, it is recommended to use the task scheduler instead of relying on Thread.Sleep()
and time-based checks.
An effective approach is to implement a timer in the service. The timer should fire periodically, say every 10 minutes, and verify if the date has changed since the last execution. If a new date is detected, the timer is paused while the cleanup process is performed, ensuring that it is only performed once per day.
private Timer _timer; private DateTime _lastRun = DateTime.Now.AddDays(-1); protected override void OnStart(string[] args) { _timer = new Timer(10 * 60 * 1000); // 每10分钟 _timer.Elapsed += new System.Timers.ElapsedEventHandler(timer_Elapsed); _timer.Start(); //... } private void timer_Elapsed(object sender, System.Timers.ElapsedEventArgs e) { // 忽略时间,只比较日期 if (_lastRun.Date < DateTime.Now.Date) { // 在运行清理任务时停止计时器 _timer.Stop(); // // 执行清理任务 // _lastRun = DateTime.Now; _timer.Start(); } }
The above is the detailed content of How to Schedule a Daily Task in a C# Windows Service?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
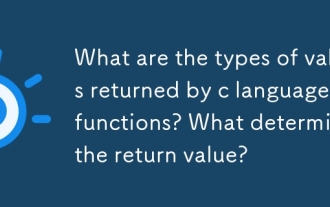
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
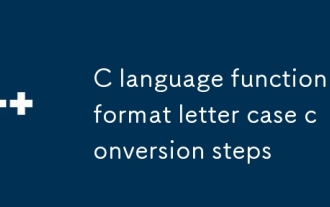
C language function format letter case conversion steps

Where is the return value of the c language function stored in memory?
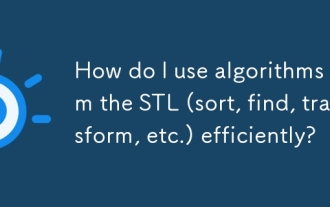
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
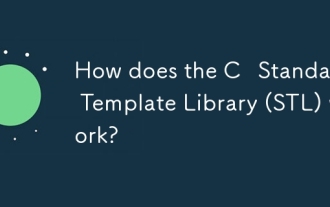
How does the C Standard Template Library (STL) work?
