How Can I Dynamically Order Data in LINQ Using a Parameter?
Jan 10, 2025 am 11:54 AMLINQ dynamic sorting: understanding the dynamic parameters of the OrderBy method
The OrderBy method in LINQ queries allows efficient sorting of data. But what if you want to dynamically specify sorting parameters based on parameters? This article will guide you through this.
Current implementation
Suppose you have a list of Student objects and want to sort them by their Address property:
List<Student> existingStudents = new List<Student> { new Student {...}, new Student {...} }; List<Student> orderbyAddress = existingStudents.OrderBy(c => c.Address).ToList();
However, this hardcodes the address ordering.
Dynamic sorting
To dynamically specify sorting parameters, you can leverage reflection to construct an expression tree:
public static IQueryable<T> OrderBy<T>(this IQueryable<T> source, string orderByProperty, bool desc) { // ... (此处应提供答案中的代码片段) }
How to use
You can now use the OrderBy extension method with dynamic parameters:
string param = "City"; List<Student> orderbyCity = existingStudents.OrderBy("City", true).ToList(); // 降序排序
This approach provides the flexibility to specify sorting parameters at runtime, allowing for customizable and dynamic sorting in LINQ queries.
The above is the detailed content of How Can I Dynamically Order Data in LINQ Using a Parameter?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
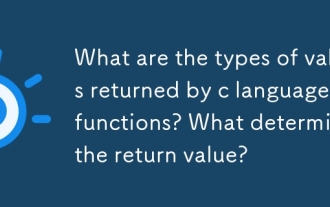
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
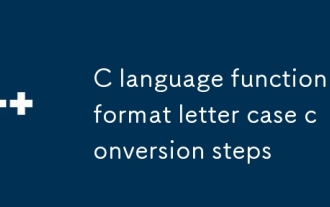
C language function format letter case conversion steps

Where is the return value of the c language function stored in memory?
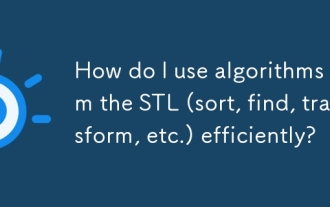
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
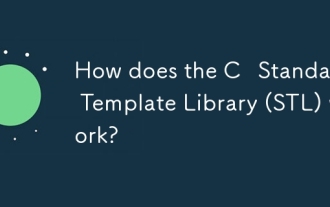
How does the C Standard Template Library (STL) work?
