Versioning in Go Huma
This guide details implementing versioned documentation in a Go Huma API. We'll create separate documentation for each API version (e.g., /v1/docs
, /v2/docs
).
The core approach involves configuring the documentation path and using middleware to dynamically load version-specific documentation content.
Configuration:
The DocsPath
in the Huma configuration dictates the documentation URL structure. We set it to /{version}/docs
to accommodate version prefixes:
config.DocsPath = "/{version}/docs"
Middleware for Version Handling:
Middleware intercepts requests to determine the API version from the URL path and loads the corresponding documentation. This example uses a chi
router:
router := chi.NewMux() router.Use(func(next http.Handler) http.Handler { return http.HandlerFunc(func(w http.ResponseWriter, r *http.Request) { urlPathParts := strings.Split(r.URL.Path, "/") versions := []string{"v1", "v2", "v3"} // Supported versions if helpers.Contains(versions, urlPathParts[1]) { // Check if a valid version is present versionPath := urlPathParts[1] versionNumberString := strings.TrimPrefix(versionPath, "v") versionNumber, _ := strconv.Atoi(versionNumberString) config := huma.DefaultConfig("API V"+versionNumberString, versionNumberString+".0.0") overviewFilePath := fmt.Sprintf("docs/v%s/overview.md", versionNumberString) // Path to version-specific overview overview, err := ioutil.ReadFile(overviewFilePath) if err != nil { http.Error(w, fmt.Sprintf("Error reading documentation: %v", err), http.StatusInternalServerError) //Improved error handling return } config.Info.Description = string(overview) api := humachi.New(router, config) switch versionNumber { case 1: api = v1handlers.AddV1Middleware(api) v1handlers.AddV1Routes(api) case 2: api = v2handlers.AddV2Middleware(api) v2handlers.AddV2Routes(api) case 3: //Explicitly handle version 3 api = v3handlers.AddV3Middleware(api) router = v3handlers.AddV3ErrorResponses(router) //Handle error responses separately if needed v3handlers.AddV3Routes(api) default: http.Error(w, "Unsupported API version", http.StatusBadRequest) //Handle unsupported versions return } } next.ServeHTTP(w, r) }) }) //Final Huma config (for default/fallback behavior if no version is specified) config := huma.DefaultConfig("API V3", "3.0.0") config.DocsPath = "/{version}/docs" humachi.New(router, config)
This middleware extracts the version, reads the corresponding overview.md
file (adjust the path as needed), sets the description in the Huma config, and then registers the appropriate handlers for that version. Error handling is improved to provide more informative responses. Note the explicit handling of version 3 and a default case for unsupported versions. Remember to replace placeholders like v1handlers
, v2handlers
, v3handlers
, and helpers.Contains
with your actual implementations. The helpers.Contains
function should check if a string exists in a slice of strings.
This setup ensures that the correct documentation is served based on the requested API version. Remember to create the docs/v{version}/overview.md
files for each version.
The above is the detailed content of Versioning in Go Huma. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










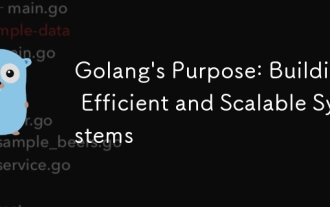
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
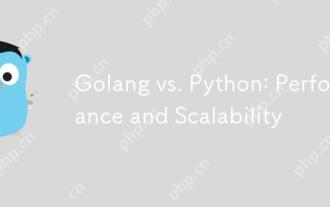
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
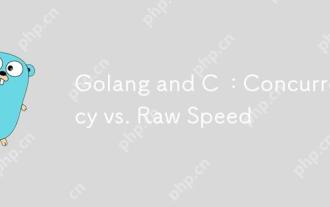
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
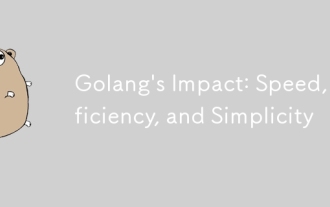
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
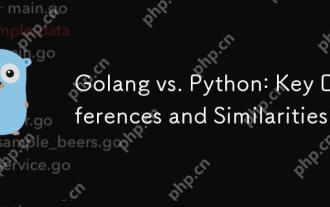
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
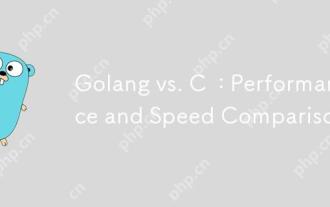
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
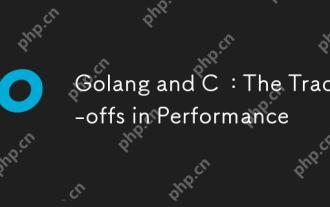
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
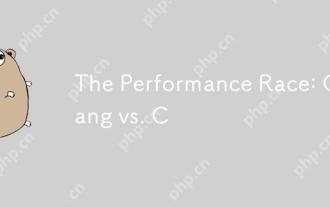
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
