


How Can I Safely Select the Correct Generic Method Overload Using Reflection in C#?
Jan 11, 2025 pm 01:27 PMUse reflection to choose the correct generic method
When using generic methods via reflection, choosing the correct overload can be challenging when there are multiple generic overloads. This problem occurs when the method name is ambiguous, such as the "Where" method in the System.Linq.Queryable class.
Instead of relying on assumptions or checking method names, there is a compile-time safe way to select the correct generic overload using delegates.
Static method
Consider the following static method with multiple generic overloads:
public static void DoSomething<TModel>(TModel model) public static void DoSomething<TViewModel, TModel>(TViewModel viewModel, TModel model)
To select the first overload (void return type, one generic parameter), create an operation delegate matching its signature:
var method = new Action<object>(MyClass.DoSomething<object>);
For the second overload (void return type, two generic parameters), use an action delegate with two object parameters:
var method = new Action<object, object>(MyClass.DoSomething<object, object>);
This method selects the correct overload based on the delegate's generic count and argument count.
To obtain a MethodInfo object, use the delegate's Method property and call MakeGenericMethod():
var methodInfo = method.Method.MakeGenericMethod(type1, type2);
Static extension method
For instance methods, use a similar method select method, but call GetGenericMethodDefinition() before passing the type to MakeGenericMethod():
var methodInfo = method.Method.GetGenericMethodDefinition().MakeGenericMethod(type1);
Decoupling MethodInfo and parameter types
You can get a generic MethodInfo object by calling GetGenericMethodDefinition(), thereby decoupling the selection of MethodInfo objects from the parameter types:
var methodInfo = method.Method.GetGenericMethodDefinition();
Then, pass the necessary types to MakeGenericMethod() when calling the method:
processCollection(methodInfo, type2); ... protected void processCollection(MethodInfo method, Type type2) { var type1 = typeof(MyDataClass); object output = method.MakeGenericMethod(type1, type2).Invoke(null, new object[] { collection }); }
The above is the detailed content of How Can I Safely Select the Correct Generic Method Overload Using Reflection in C#?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
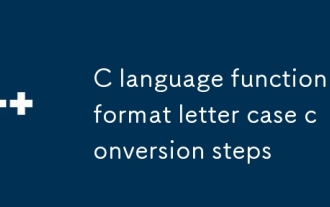
C language function format letter case conversion steps
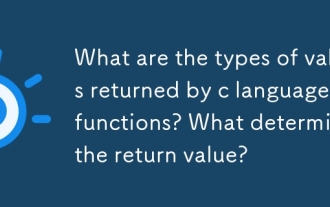
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
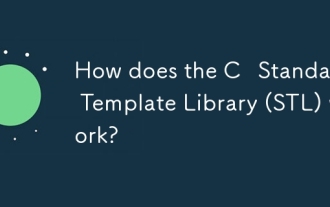
How does the C Standard Template Library (STL) work?

Where is the return value of the c language function stored in memory?
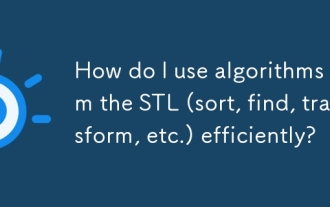
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
