How to Pivot a Column in SQL Using CASE WHEN and SUM()?
Using CASE WHEN and SUM() to Pivot a Column in SQL
Data transformation often requires reshaping tables, moving data from rows into columns—a process known as pivoting. This example demonstrates how to pivot a column in SQL using CASE WHEN
and SUM()
.
Let's consider a sample table named "Bank":
Bank: name val amount John 1 2000 Peter 1 1999 Peter 2 1854 John 2 1888
Our goal is to transform this table into the following format:
name amountVal1 amountVal2 John 2000 1888 Peter 1999 1854
Why a Simple CASE WHEN Fails
A naive approach using only CASE WHEN
might look like this:
SELECT name, CASE WHEN val = 1 THEN amount ELSE 0 END AS amountVal1, CASE WHEN val = 2 THEN amount ELSE 0 END AS amountVal2 FROM bank;
This produces an incorrect result:
name amountVal1 amountVal2 John 2000 0 Peter 1999 0 John 0 1888 Peter 0 1854
The problem is that each row is treated independently. We need to aggregate the results.
The Correct Solution with SUM()
The solution lies in using the SUM()
aggregate function to sum the amount
values for each name
and val
combination:
SELECT name, SUM(CASE WHEN val = 1 THEN amount ELSE 0 END) AS amountVal1, SUM(CASE WHEN val = 2 THEN amount ELSE 0 END) AS amountVal2 FROM bank GROUP BY name;
This query correctly produces the pivoted table:
name amountVal1 amountVal2 John 2000 1888 Peter 1999 1854
The GROUP BY name
clause ensures that the SUM()
function aggregates the amounts for each unique name. The CASE WHEN
statements conditionally assign amounts to the appropriate columns (amountVal1
and amountVal2
), handling cases where a particular val
is absent for a given name by using 0 as the default value. This technique provides a concise and efficient way to perform column pivoting in SQL.
The above is the detailed content of How to Pivot a Column in SQL Using CASE WHEN and SUM()?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










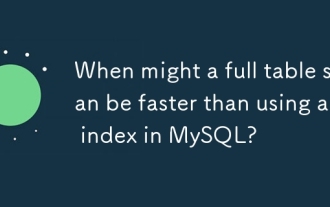
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
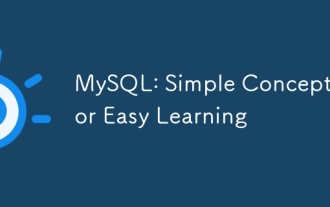
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
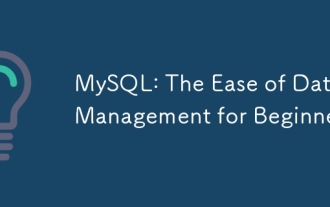
MySQL is suitable for beginners because it is simple to install, powerful and easy to manage data. 1. Simple installation and configuration, suitable for a variety of operating systems. 2. Support basic operations such as creating databases and tables, inserting, querying, updating and deleting data. 3. Provide advanced functions such as JOIN operations and subqueries. 4. Performance can be improved through indexing, query optimization and table partitioning. 5. Support backup, recovery and security measures to ensure data security and consistency.
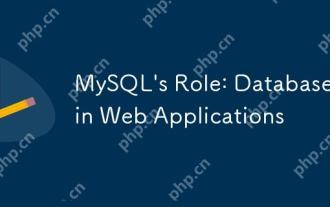
The main role of MySQL in web applications is to store and manage data. 1.MySQL efficiently processes user information, product catalogs, transaction records and other data. 2. Through SQL query, developers can extract information from the database to generate dynamic content. 3.MySQL works based on the client-server model to ensure acceptable query speed.
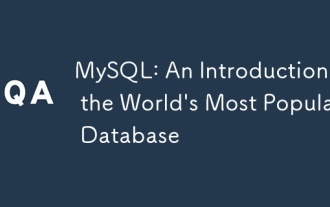
MySQL is an open source relational database management system, mainly used to store and retrieve data quickly and reliably. Its working principle includes client requests, query resolution, execution of queries and return results. Examples of usage include creating tables, inserting and querying data, and advanced features such as JOIN operations. Common errors involve SQL syntax, data types, and permissions, and optimization suggestions include the use of indexes, optimized queries, and partitioning of tables.
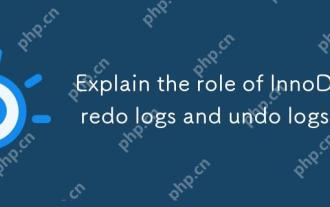
InnoDB uses redologs and undologs to ensure data consistency and reliability. 1.redologs record data page modification to ensure crash recovery and transaction persistence. 2.undologs records the original data value and supports transaction rollback and MVCC.
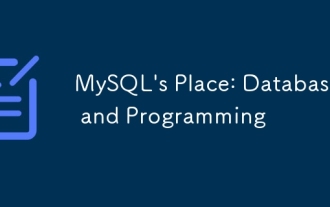
MySQL's position in databases and programming is very important. It is an open source relational database management system that is widely used in various application scenarios. 1) MySQL provides efficient data storage, organization and retrieval functions, supporting Web, mobile and enterprise-level systems. 2) It uses a client-server architecture, supports multiple storage engines and index optimization. 3) Basic usages include creating tables and inserting data, and advanced usages involve multi-table JOINs and complex queries. 4) Frequently asked questions such as SQL syntax errors and performance issues can be debugged through the EXPLAIN command and slow query log. 5) Performance optimization methods include rational use of indexes, optimized query and use of caches. Best practices include using transactions and PreparedStatemen
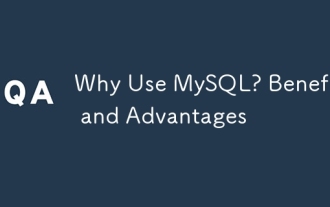
MySQL is chosen for its performance, reliability, ease of use, and community support. 1.MySQL provides efficient data storage and retrieval functions, supporting multiple data types and advanced query operations. 2. Adopt client-server architecture and multiple storage engines to support transaction and query optimization. 3. Easy to use, supports a variety of operating systems and programming languages. 4. Have strong community support and provide rich resources and solutions.
