


How Do Static Variables Function and Why Can't They Be Declared Inside Methods in C#?
C# Static Variables: Purpose and Usage
In C#, static variables occupy a unique position in the programming world. They are shared among all instances of the class, providing a convenient way to store information that is consistent throughout the class.
Why declare static variables?
Static variables have three main uses:
- Global access: Static variables can be accessed without creating an instance of the class. This is particularly useful for storing configuration settings or values that apply to all instances.
- Singleton Pattern: You can enforce the singleton pattern by restricting the declaration of a property or method to static, ensuring that there is only one instance of the class.
- Memory Optimization: Static variables are stored in shared memory space, which can optimize memory utilization when creating multiple instances of a class.
Why can’t static variables be declared inside a method?
Unlike instance variables that are associated with a specific instance of a class, static variables belong to the class itself. Therefore, they must be declared at the class level, outside of any methods.
Example
Consider the following example:
class Book { public static int myInt = 0; } public class Exercise { static void Main() { Book book = new Book(); Console.WriteLine(book.myInt); // 显示错误 } }
In this code, the error occurs because the static variable myInt cannot be accessed through an instance of the class. To access it, you need to reference it directly:
Console.WriteLine(Book.myInt);
Practical application of static variables
Let’s delve into a more detailed example illustrating the use of static variables. The following code demonstrates how a static variable stores a running total:
class Counter { public static int TotalCount { get; set; } public void AddCount(int value) { TotalCount += value; } } public class Program { static void Main() { var counter1 = new Counter(); counter1.AddCount(5); var counter2 = new Counter(); counter2.AddCount(10); Console.WriteLine(Counter.TotalCount); // 输出:15 } }
In this case, the TotalCount variable shared between all Counter instances is used to hold the running total of the added values. Each time a new Counter is created, it inherits the latest value stored in TotalCount.
Conclusion
Static variables in C# provide a powerful mechanism to manage shared information among classes. By understanding their purpose and usage, developers can optimize memory utilization, enforce singleton patterns, and facilitate global access to important data.
The above is the detailed content of How Do Static Variables Function and Why Can't They Be Declared Inside Methods in C#?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










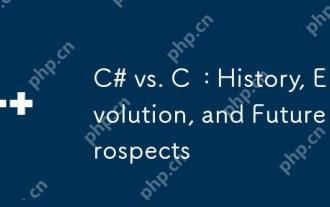
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
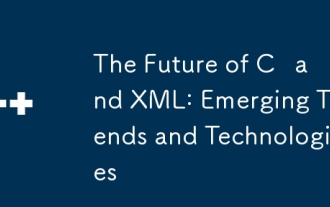
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
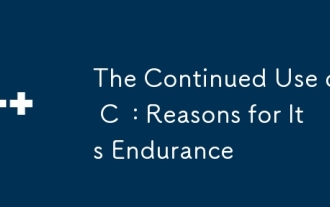
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
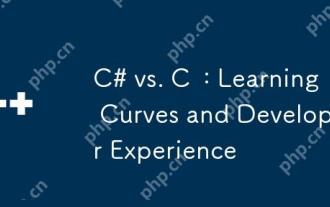
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
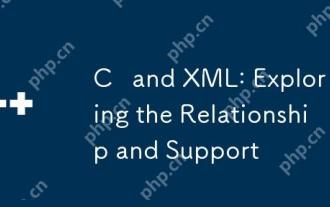
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
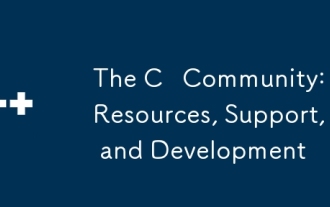
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
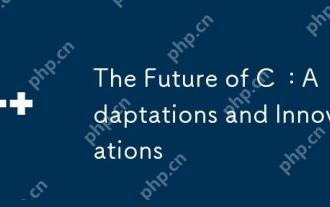
The future of C will focus on parallel computing, security, modularization and AI/machine learning: 1) Parallel computing will be enhanced through features such as coroutines; 2) Security will be improved through stricter type checking and memory management mechanisms; 3) Modulation will simplify code organization and compilation; 4) AI and machine learning will prompt C to adapt to new needs, such as numerical computing and GPU programming support.
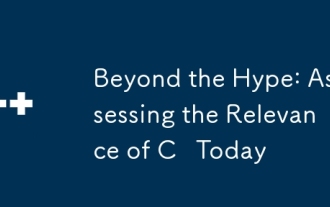
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
