How to Retrieve a Property Name as a String in C#?
Accessing Property Names as Strings in C#
In C# programming, particularly when using reflection, it's frequently necessary to obtain a property's name as a string. This proves invaluable for tasks such as dynamic method invocation or safeguarding against accidental property renaming.
Leveraging the nameof
Operator (C# 6.0 and later)
Since C# 6.0, the nameof
operator offers a simple and efficient solution. The expression nameof(SomeProperty)
directly yields the string "SomeProperty" at compile time.
A Generic Property Name Retrieval Method
For versions of C# prior to 6.0, a generic method provides a workaround:
public static string GetPropertyName<T>(Expression<Func<T>> propertyLambda) { var me = propertyLambda.Body as MemberExpression; if (me == null) { throw new ArgumentException("Invalid lambda expression"); } return me.Member.Name; }
This method accepts a lambda expression referencing a property and returns its name.
Practical Application
Here's how to utilize the GetPropertyName
method:
// For a static property: string propertyName = GetPropertyName(() => SomeClass.SomeProperty); // For an instance property: string propertyName = GetPropertyName(() => someObject.SomeProperty);
Summary
Whether using the modern nameof
operator or the GetPropertyName
method, retrieving property names as strings is simplified, enhancing code maintainability and robustness when dealing with reflection and refactoring.
The above is the detailed content of How to Retrieve a Property Name as a String in C#?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


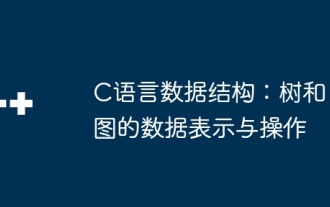
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
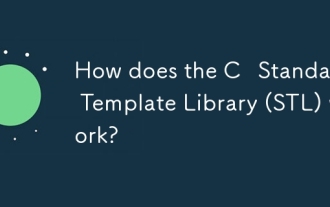
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
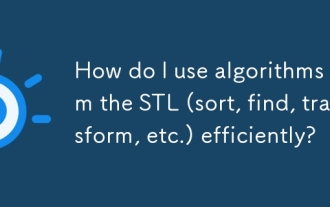
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
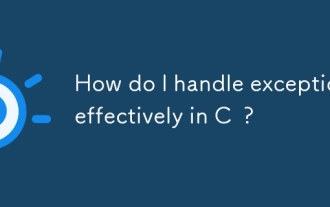
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
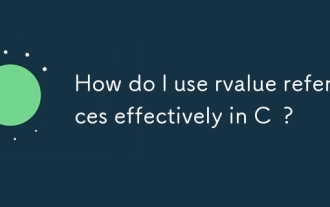
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
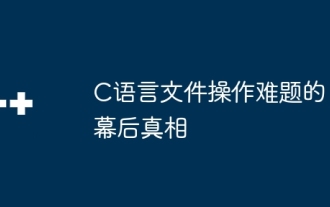
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
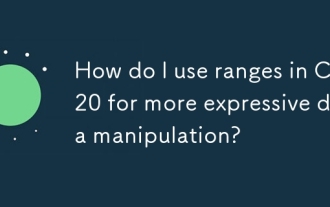
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
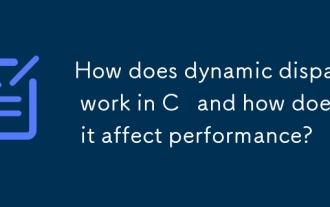
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
