How Can I Effectively Unit Test Code Dependent on `DateTime.Now`?
Jan 12, 2025 am 08:01 AMUnit Testing and DateTime.Now
: Best Practices
Unit testing often requires controlling the system time to simulate various scenarios. Directly altering the system clock is impractical and risky. The solution lies in dependency injection and abstraction.
The Power of Abstraction
The key is to abstract the time source and inject it into your code. This allows easy substitution of the real time provider with a mock during testing.
Let's create an interface:
interface ITimeProvider { DateTime UtcNow { get; } }
This interface defines a contract for obtaining the current UTC time. We'll implement it with a default class:
class DefaultTimeProvider : ITimeProvider { public DateTime UtcNow => DateTime.UtcNow; }
Now, let's use this in a dependent class:
class MyDependentClass { private readonly ITimeProvider _timeProvider; public MyDependentClass(ITimeProvider timeProvider) { _timeProvider = timeProvider; } public void MyMethod() { var currentTime = _timeProvider.UtcNow; // ... use currentTime ... } }
In tests, you can inject a mock ITimeProvider
returning a predetermined DateTime
value, providing complete control over time within your tests.
The Ambient Context Approach
Alternatively, an ambient context can be used:
public static class TimeContext { private static ITimeProvider _current = new DefaultTimeProvider(); public static ITimeProvider Current { get => _current; set => _current = value; } }
This provides a globally accessible time provider. In your tests, you can temporarily set TimeContext.Current
to a mock, and remember to restore it to the default after each test to avoid side effects.
Remember: Clean up your ambient context after each test to prevent unintended consequences in subsequent tests or the application itself. Using a using
statement or a finally
block is highly recommended for this purpose.
The above is the detailed content of How Can I Effectively Unit Test Code Dependent on `DateTime.Now`?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
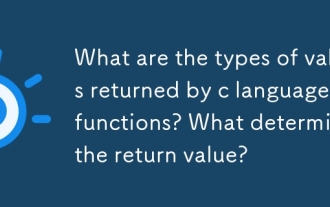
What are the types of values returned by c language functions? What determines the return value?
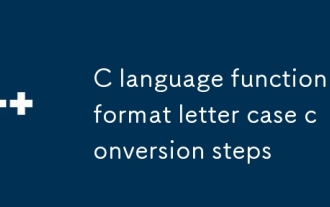
C language function format letter case conversion steps

What are the definitions and calling rules of c language functions and what are the

Where is the return value of the c language function stored in memory?
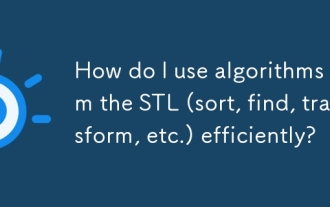
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
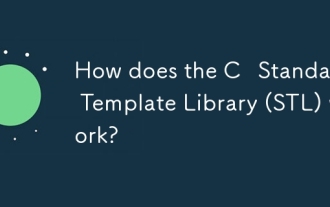
How does the C Standard Template Library (STL) work?
