


How to Efficiently Insert Multiple Parameterized Rows into a Database using C#?
Optimizing Database Inserts in C#: A Single Parameterized Query Approach
Inserting numerous rows into a database individually is inefficient. This article demonstrates a high-performance method using a single parameterized query in C# to achieve bulk insertion.
The key is to leverage stored procedures and table-valued parameters. This approach involves three main steps:
-
Defining a Table Type:
Create a custom SQL Server table type to define the structure of your data, specifying column names and data types.
-
Creating a Stored Procedure:
Develop a stored procedure that accepts your custom table type as a read-only, table-valued parameter. This procedure will then insert the data into your target database table. Remember, table-valued parameters must be
READONLY
. -
Implementing the C# Code:
In your C# application:
- Create a
DataTable
object, mirroring the structure of your custom table type. - Populate this
DataTable
with the data you intend to insert. - Execute the stored procedure, passing the
DataTable
as the table-valued parameter.
- Create a
Here's an example illustrating this process:
Table Type (SQL):
CREATE TYPE MyTableType AS TABLE ( Col1 INT, Col2 VARCHAR(20) ) GO
Stored Procedure (SQL):
CREATE PROCEDURE MyProcedure (@MyTable MyTableType READONLY) AS BEGIN INSERT INTO MyTable (Col1, Col2) SELECT Col1, Col2 FROM @MyTable; END; GO
C# Code:
using System.Data; using System.Data.SqlClient; // ... other code ... DataTable dt = new DataTable(); dt.Columns.Add("Col1", typeof(int)); dt.Columns.Add("Col2", typeof(string)); // Populate dt with your data here... e.g., //DataRow row = dt.NewRow(); //row["Col1"] = 1; //row["Col2"] = "Value1"; //dt.Rows.Add(row); using (SqlConnection con = new SqlConnection("ConnectionString")) { using (SqlCommand cmd = new SqlCommand("MyProcedure", con)) { cmd.CommandType = CommandType.StoredProcedure; cmd.Parameters.AddWithValue("@MyTable", dt); //AddWithValue handles type inference con.Open(); cmd.ExecuteNonQuery(); } }
This method significantly improves database insertion efficiency by reducing the number of database round trips, leading to faster and more scalable data processing.
The above is the detailed content of How to Efficiently Insert Multiple Parameterized Rows into a Database using C#?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


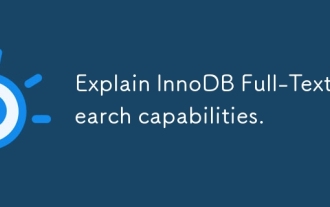
InnoDB's full-text search capabilities are very powerful, which can significantly improve database query efficiency and ability to process large amounts of text data. 1) InnoDB implements full-text search through inverted indexing, supporting basic and advanced search queries. 2) Use MATCH and AGAINST keywords to search, support Boolean mode and phrase search. 3) Optimization methods include using word segmentation technology, periodic rebuilding of indexes and adjusting cache size to improve performance and accuracy.
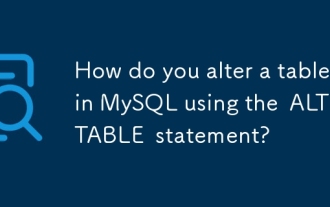
The article discusses using MySQL's ALTER TABLE statement to modify tables, including adding/dropping columns, renaming tables/columns, and changing column data types.
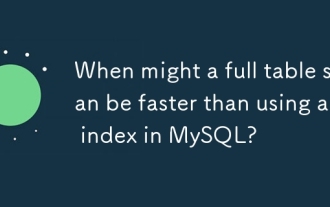
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
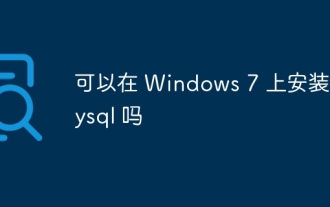
Yes, MySQL can be installed on Windows 7, and although Microsoft has stopped supporting Windows 7, MySQL is still compatible with it. However, the following points should be noted during the installation process: Download the MySQL installer for Windows. Select the appropriate version of MySQL (community or enterprise). Select the appropriate installation directory and character set during the installation process. Set the root user password and keep it properly. Connect to the database for testing. Note the compatibility and security issues on Windows 7, and it is recommended to upgrade to a supported operating system.
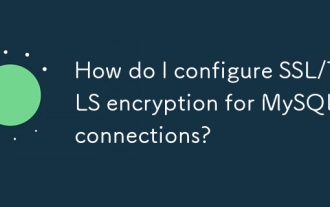
Article discusses configuring SSL/TLS encryption for MySQL, including certificate generation and verification. Main issue is using self-signed certificates' security implications.[Character count: 159]
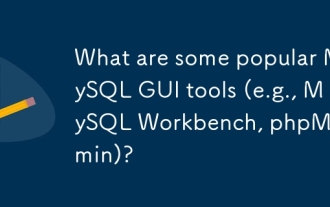
Article discusses popular MySQL GUI tools like MySQL Workbench and phpMyAdmin, comparing their features and suitability for beginners and advanced users.[159 characters]
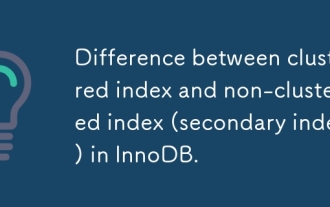
The difference between clustered index and non-clustered index is: 1. Clustered index stores data rows in the index structure, which is suitable for querying by primary key and range. 2. The non-clustered index stores index key values and pointers to data rows, and is suitable for non-primary key column queries.
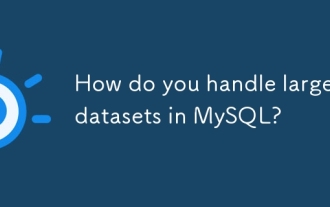
Article discusses strategies for handling large datasets in MySQL, including partitioning, sharding, indexing, and query optimization.
