


How to Efficiently Convert an Integer to its Binary Representation in C#?
Jan 12, 2025 am 08:29 AMConversion of integer to binary representation in C#
Converting an integer to its binary representation is a common programming task. In C#, there are several ways to perform this conversion, including using the ToInt32 and ToString methods of the Convert class.
To demonstrate this process, let's solve a problem encountered by a user who was trying to convert an integer represented as a string into its binary representation:
<code>String input = "8"; String output = Convert.ToInt32(input, 2).ToString();</code>
This code throws an exception with the message "No parsable numbers found". This is because ToInt32 expects the input string to represent a decimal integer, not a binary integer, and the string "8" represents the decimal value 8.
To correctly convert an integer to its binary representation, we use the Convert.ToString method and specify a base of 2. Here is an updated code snippet:
<code>int value = 8; string binary = Convert.ToString(value, 2);</code>
This code converts an integer value (whose decimal value is 8) into a string representing its binary representation, resulting in the string "1000".
The above is the detailed content of How to Efficiently Convert an Integer to its Binary Representation in C#?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
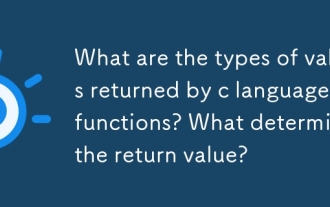
What are the types of values returned by c language functions? What determines the return value?
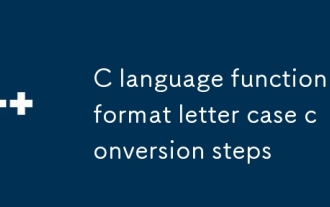
C language function format letter case conversion steps

What are the definitions and calling rules of c language functions and what are the

Where is the return value of the c language function stored in memory?
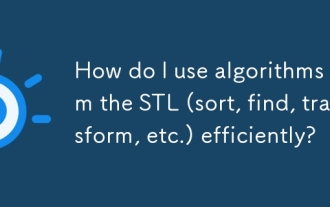
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
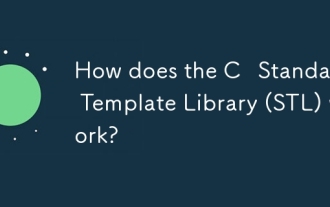
How does the C Standard Template Library (STL) work?
