


Why Does Saving an Image to a Directory Result in 'Access Denied' in C#?
Troubleshooting Image Saving Errors in .NET C#
Saving images to a directory in .NET C# can sometimes throw an "Access to the path '...' is denied" error, even with seemingly correct permissions. This often happens when targeting a directory instead of a specific file.
The Problem:
Attempting to save an image to a directory path (e.g., C:\inetpub\wwwroot\mysite\images\savehere
) directly results in an access denied error. The file system prevents overwriting an entire directory with a single file to avoid accidental data loss.
The Fix:
The solution is simple: specify a complete file path including the filename. Instead of just the directory, use a path like this:
'C:\inetpub\wwwroot\mysite\images\savehere\mumble.jpg'
For robust path construction, leverage the Path.Combine()
method to prevent potential path-related issues:
string directoryPath = "C:\inetpub\wwwroot\mysite\images\savehere"; string fileName = "mumble.jpg"; string filePath = Path.Combine(directoryPath, fileName); // ... save the image to filePath ...
This ensures correct path concatenation regardless of the operating system.
The above is the detailed content of Why Does Saving an Image to a Directory Result in 'Access Denied' in C#?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


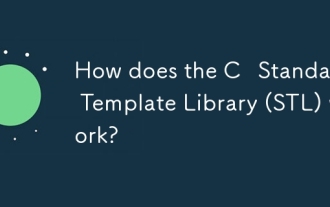
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
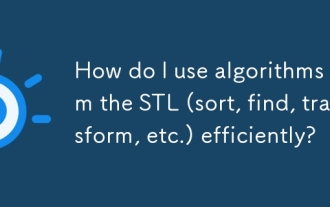
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
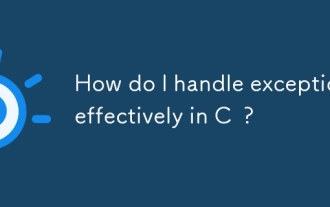
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
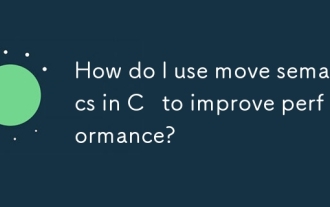
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
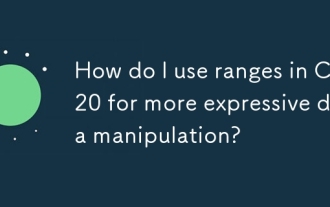
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
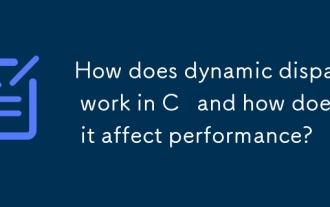
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
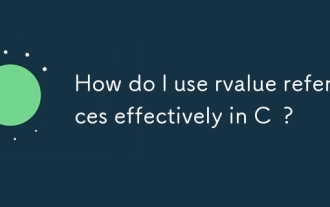
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
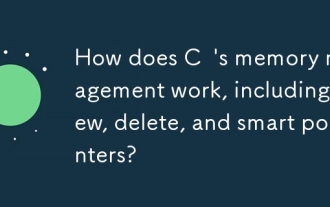
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
