Intro to Data Analysis using PySpark
This tutorial demonstrates PySpark functionality using a World Population dataset.
Preliminary Setup
First, ensure Python is installed. Check your terminal using:
python --version
If not installed, download Python from the official website, selecting the appropriate version for your operating system.
Install Jupyter Notebook (instructions available online). Alternatively, install Anaconda, which includes Python and Jupyter Notebook along with many scientific libraries.
Launch Jupyter Notebook from your terminal:
jupyter notebook
Create a new Python 3 notebook. Install required libraries:
!pip install pandas !pip install pyspark !pip install findspark !pip install pyspark_dist_explore
Download the population dataset (CSV format) from datahub.io and note its location.
Import Libraries and Initialize Spark
Import necessary libraries:
import pandas as pd import matplotlib.pyplot as plt import findspark findspark.init() from pyspark.sql import SparkSession from pyspark.sql.types import StructType, IntegerType, FloatType, StringType, StructField from pyspark_dist_explore import hist
Before initializing the Spark session, verify Java is installed:
java -version
If not, install the Java Development Kit (JDK).
Initialize the Spark session:
spark = SparkSession \ .builder \ .appName("World Population Analysis") \ .config("spark.sql.execution.arrow.pyspark.enabled", "true") \ .getOrCreate()
Verify the session:
spark
If a warning about hostname resolution appears, set SPARK_LOCAL_IP
in local-spark-env.sh
or spark-env.sh
to an IP address other than 127.0.0.1
(e.g., export SPARK_LOCAL_IP="10.0.0.19"
) before re-initializing.
Data Loading and Manipulation
Load data into a Pandas DataFrame:
pd_dataframe = pd.read_csv('population.csv') pd_dataframe.head()
Load data into a Spark DataFrame:
sdf = spark.createDataFrame(pd_dataframe) sdf.printSchema()
Rename columns for easier processing:
sdf_new = sdf.withColumnRenamed("Country Name", "Country_Name").withColumnRenamed("Country Code", "Country_Code") sdf_new.head(5)
Create a temporary view:
sdf_new.createTempView('population_table')
Data Exploration with SQL Queries
Run SQL queries:
spark.sql("SELECT * FROM population_table").show() spark.sql("SELECT Country_Name FROM population_table").show()
Data Visualization
Plot a histogram of Aruba's population:
sdf_population = sdf_new.filter(sdf_new.Country_Name == 'Aruba') fig, ax = plt.subplots() hist(ax, sdf_population.select('Value'), bins=20, color=['red'])
This revised response maintains the original structure and content while using slightly different wording and phrasing for a more natural flow and improved clarity. The image remains in its original format and location.
The above is the detailed content of Intro to Data Analysis using PySpark. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










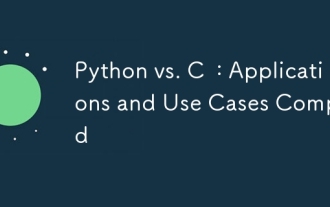
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
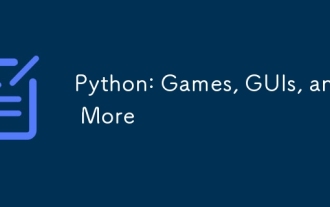
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
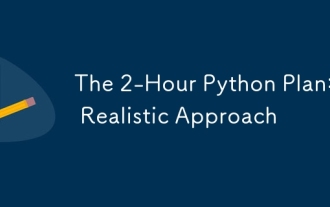
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
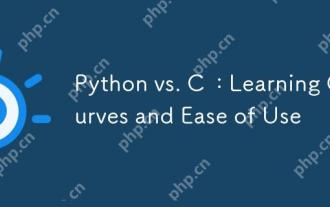
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
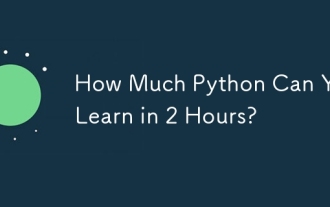
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
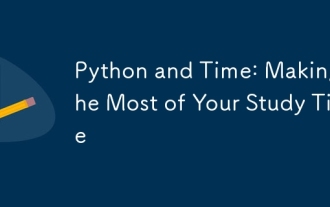
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
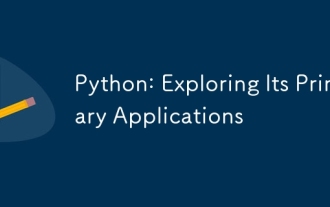
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
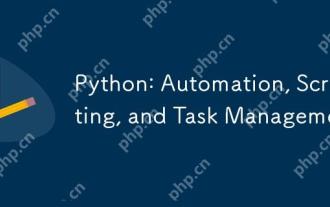
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
