Beyond MVC: Redefining Backend Development with DataForge
Backend development often relies on the MVC (Model-View-Controller) architecture, with frameworks like Laravel's Eloquent ORM providing a solid foundation. However, scaling complex projects presents challenges: managing reusable SQL logic, adapting queries for diverse output formats, handling intricate entity relationships, and designing scalable APIs. These hurdles inspired the creation of DataForge, a Laravel-based framework that transcends the limitations of traditional MVC. DataForge champions a product-centric approach, prioritizing modularity, scalability, and maintainability.
DataForge's Distinguishing Features
1. Modular SQL Class Design
Eloquent simplifies database interactions, but often necessitates repetitive code for varying query needs (e.g., retrieving lists versus single rows). DataForge addresses this with reusable SQL classes, enabling modular select types for efficient handling of multiple use cases.
Example:
$query = new Query('ProductList'); $query->select('list', 'p.id, p.name, p.price, c.name AS category'); $query->select('item', 'p.id, p.name, p.description, p.price, c.name AS category'); $query->select('options', 'p.id, p.name'); $query->select('total', 'COUNT(p.id) AS totalCount'); $query->filterOptional('p.category_id = {category_id}'); $query->filterOptional('p.name LIKE {%keyword%}'); $query->order('{sort}', '{order}');
This allows retrieval of:
- Product lists:
$products = Sql('Product:list', ['select' => 'list'])->fetchRowList();
- Single product details:
$product = Sql('Product:list', ['select' => 'item', 'id' => 123])->fetchRow();
- Total counts:
$count = Sql('Product:list', ['select' => 'total'])->fetchColumn();
2. Advanced Entity Capabilities
Eloquent models frequently blend data access and business logic, impacting maintainability. DataForge's Entity class improves modularity by cleanly separating these concerns, incorporating advanced features:
-
Lazy Loading: Attributes load only when accessed, optimizing performance by avoiding unnecessary queries.
$product = DataForge::getProduct(123); echo $product->Price;
(loadsgetPrice()
only when accessed). -
Inter-Entity Connections: Effortless relationship management between entities for streamlined data retrieval.
$product = DataForge::getProduct(123); $category = $product->Category;
(directly fetches the related Category entity).
Example: Product Entity
class Product extends Entity { function init($id) { return \Sql('Product:list', ['id' => $id, 'select' => 'entity'])->fetchRow(); } function getCategory() { return DataForge::getCategory($this->category_id); } }
3. Versatile API Interface
DataForge offers seven robust API endpoints, providing a structured, scalable approach to backend interaction:
- /api/list: Paginated record lists.
- /api/all: All matching records (no pagination).
- /api/item: Single record retrieval.
- /api/field: Single column value retrieval.
- /api/entity: Direct entity manipulation, including lazy loading and relationships.
- /api/Task: Complex workflows combining SQL and Entity operations.
- /api/GuestTask: Secure, limited guest access to specific workflows.
Example: Paginated product list: /api/list/Product:list?keyword=test&pageNo=1&limit=10&sort=price&order=asc
Example Response (JSON):
$query = new Query('ProductList'); $query->select('list', 'p.id, p.name, p.price, c.name AS category'); $query->select('item', 'p.id, p.name, p.description, p.price, c.name AS category'); $query->select('options', 'p.id, p.name'); $query->select('total', 'COUNT(p.id) AS totalCount'); $query->filterOptional('p.category_id = {category_id}'); $query->filterOptional('p.name LIKE {%keyword%}'); $query->order('{sort}', '{order}');
This modular approach simplifies API development while maintaining flexibility and scalability.
4. SQL-Based Workflow Management
Traditional frameworks often require distinct methods for fetching lists, single rows, or aggregated data. DataForge's modular select types in SQL classes allow dynamic adjustment of queries to return the desired data format without redundant coding.
DataForge's Enhancement of Laravel
Integrating DataForge into Laravel projects offers:
- Streamlined query logic through reusable SQL queries.
- Advanced entity relationships and lazy loading.
- Adaptability to multiple data formats (list, row, column) without code duplication.
- Simplified API development using pre-built endpoints.
- Enhanced performance and security with features like
filterOptional
andfilterAnyOneRequired
.
Further Information and Getting Started
DataForge is a comprehensive backend toolkit designed for building structured, scalable products efficiently.
- Documentation: data-forge.tech
- Updates: LinkedIn Page
- Repository: GitHub Repository
We encourage feedback and discussion on how DataForge compares to your current tools.
Discussion Points
- How do you currently manage SQL queries, APIs, and entity relationships?
- What challenges have you encountered with ORMs like Eloquent?
Let's collaborate to redefine backend development.
The above is the detailed content of Beyond MVC: Redefining Backend Development with DataForge. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










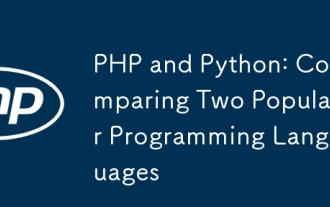
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
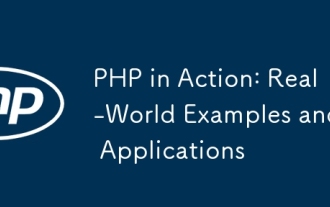
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
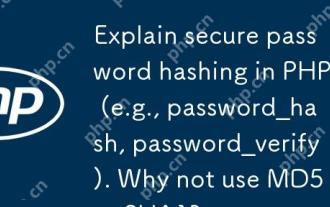
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
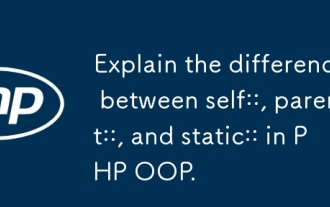
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
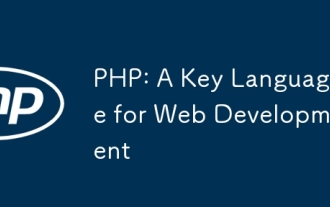
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
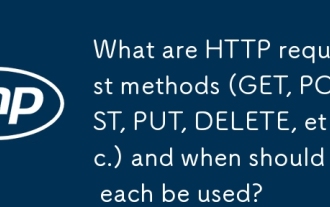
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
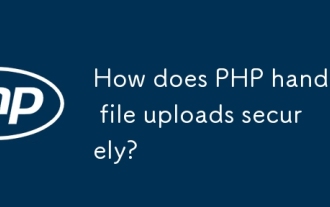
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
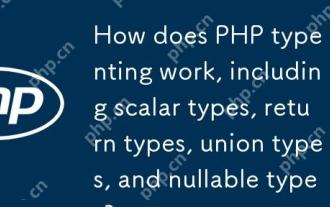
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
