How to Perform an Inner Join on DataTables in C# using LINQ?
Inner join to DataTables using LINQ in C#
Inner join is a relational database operation that retrieves data from two or more tables based on a common column. In C#, you can perform inner joins on DataTables using a variety of technologies, including LINQ.
LINQ method:
If LINQ is an option, the following code snippet demonstrates how to perform an inner join on two DataTables (T1 and T2):
DataTable dt1 = new DataTable(); dt1.Columns.Add("CustID", typeof(int)); dt1.Columns.Add("ColX", typeof(int)); dt1.Columns.Add("ColY", typeof(int)); DataTable dt2 = new DataTable(); dt2.Columns.Add("CustID", typeof(int)); dt2.Columns.Add("ColZ", typeof(int)); // 添加一些示例数据 for (int i = 1; i <= 5; i++) { dt1.Rows.Add(i, 10 + i, 20 + i); dt2.Rows.Add(i, 30 + i); } // 使用LINQ执行内连接 var results = from table1 in dt1.AsEnumerable() join table2 in dt2.AsEnumerable() on table1.Field<int>("CustID") equals table2.Field<int>("CustID") select new { CustID = table1.Field<int>("CustID"), ColX = table1.Field<int>("ColX"), ColY = table1.Field<int>("ColY"), ColZ = table2.Field<int>("ColZ") }; // 输出结果 foreach (var item in results) { Console.WriteLine(String.Format("ID = {0}, ColX = {1}, ColY = {2}, ColZ = {3}", item.CustID, item.ColX, item.ColY, item.ColZ)); }
This LINQ query performs an inner join using the on
clause, which specifies that the CustID
columns of the two tables should be equal. The result is returned as an anonymous type containing the CustID
, ColX
, ColY
, and ColZ
columns.
Output:
This code will output the following results:
ID = 1, ColX = 11, ColY = 21, ColZ = 31 ID = 2, ColX = 12, ColY = 22, ColZ = 32 ID = 3, ColX = 13, ColY = 23, ColZ = 33 ID = 4, ColX = 14, ColY = 24, ColZ = 34 ID = 5, ColX = 15, ColY = 25, ColZ = 35
The above is the detailed content of How to Perform an Inner Join on DataTables in C# using LINQ?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


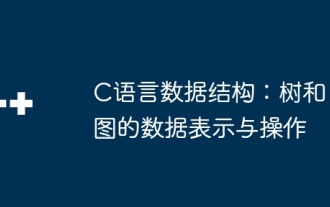
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
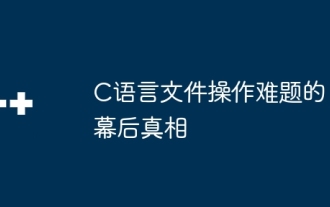
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
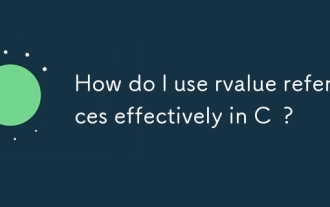
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
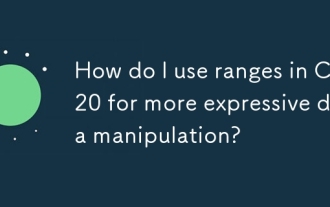
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
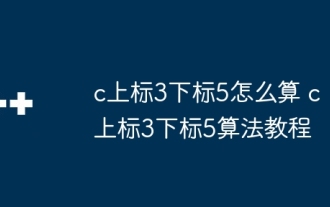
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
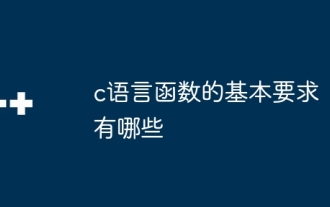
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
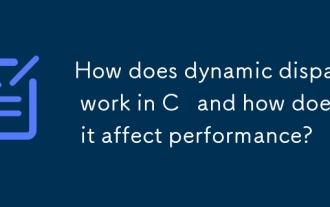
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
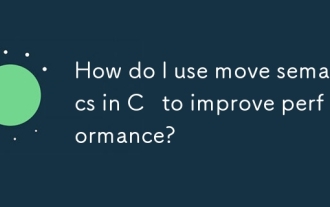
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
