Email Verifier using Go
This blog post demonstrates building a simple email verification tool using Go. It's a mini-project designed to illustrate the core concepts of email verification and its underlying mechanisms. We won't delve into every detail, but we'll cover enough to provide a solid understanding.
The process begins with verifying the domain extracted from an email address (e.g., google.com
).
Setting up your Go project:
Open your terminal and execute the following commands:
1. go mod init github.com/username/email-verifier // Replace 'username' with your GitHub username. 2. touch main.go
Implementing the Go code (main.go):
The main.go
file will contain the core logic. This initial snippet reads domain inputs from the command line:
package main import ( "bufio" "log" "net" "os" "strings" ) func main() { scanner := bufio.NewScanner(os.Stdin) for scanner.Scan() { verifyDomain(scanner.Text()) } if err := scanner.Err(); err != nil { log.Fatal("Error: could not read from input %v\n", err) } }
The verifyDomain
function handles the actual verification process. It checks for MX, SPF, and DMARC records:
func verifyDomain(domain string) { var hasMX, hasSPF, hasDMARC bool var spfRecord, dmarcRecord string // MX Record Check mxRecords, err := net.LookupMX(domain) if err != nil { log.Printf("Error: could not find MX record for %s due to %v\n", domain, err) } if len(mxRecords) > 0 { hasMX = true } // SPF Record Check txtRecords, err := net.LookupTXT("spf." + domain) if err != nil { log.Printf("Error: could not find SPF record for %s due to %v\n", domain, err) } for _, record := range txtRecords { if strings.HasPrefix(record, "v=spf1") { hasSPF = true spfRecord = record break } } // DMARC Record Check dmarcRecords, err := net.LookupTXT("_dmarc." + domain) if err != nil { log.Printf("Error: could not find DMARC record for %s due to %v\n", domain, err) } for _, record := range dmarcRecords { if strings.HasPrefix(record, "v=DMARC1") { hasDMARC = true dmarcRecord = record break } } log.Printf("Domain: %v,\n MX: %v,\n SPF: %v,\n DMARC: %v,\n SPF Rec: %v,\n DMARC Rec %v,\n\n", domain, hasMX, hasSPF, hasDMARC, spfRecord, dmarcRecord) }
Explanation of Email Verification Components:
- MX (Mail Exchanger) Record: Identifies the mail servers responsible for accepting email for a domain.
- SPF (Sender Policy Framework) Record: Specifies authorized mail servers to send emails on behalf of a domain. Helps detect spoofing.
- DMARC (Domain-based Message Authentication, Reporting & Conformance): Builds upon SPF and DKIM for enhanced email authentication and reporting. Specifies how to handle emails failing SPF or DKIM checks.
Running the code:
After saving the code, run it from your terminal: go run main.go
. Enter domain names (e.g., google.com
, example.com
) one at a time. The output will show whether MX, SPF, and DMARC records were found.
Example Output:
<code>Domain: google.com, MX: true, SPF: false, DMARC: true, SPF Rec: , DMARC Rec v=DMARC1; p=reject; rua=mailto:mailauth-reports@google.com, </code>
This output indicates that the domain google.com
has an MX record and a DMARC record, but the SPF record lookup failed in this example. The results will vary depending on the domain's DNS configuration.
Remember to replace "github.com/username/email-verifier"
with your actual GitHub repository information. Connect with me on LinkedIn, GitHub, and Twitter/X for further discussion!
The above is the detailed content of Email Verifier using Go. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










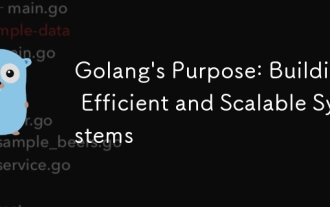
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
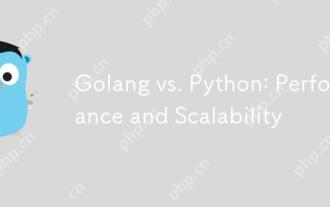
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
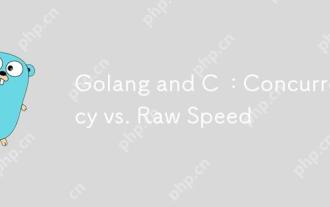
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
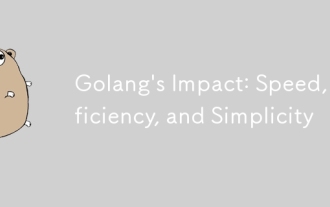
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
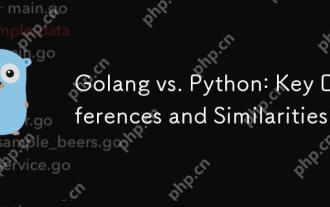
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
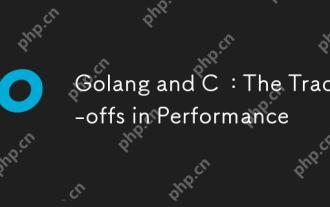
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
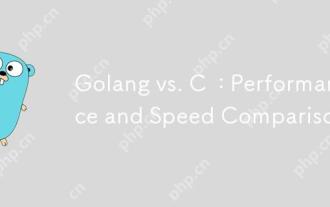
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
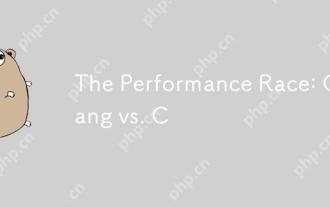
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
