


Mastering Pointers in Go: Enhancing Safety, Performance, and Code Maintainability
Pointers in Go language: a powerful tool for efficient data operations and memory management
Pointers in Go language provide developers with a powerful tool to directly access and manipulate the memory address of variables. Unlike traditional variables, which store actual data values, pointers store the memory location where those values reside. This unique feature enables pointers to modify original data in memory, providing an efficient method of data processing and program performance optimization.
Memory addresses are represented in hexadecimal format (e.g., 0xAFFFF) and are the basis for pointers. When you declare a pointer variable, it is essentially a special variable that holds the memory address of another variable, rather than the data itself.
For example, the pointer p in the Go language contains the reference 0x0001, which directly points to the memory address of another variable x. This relationship allows p to interact directly with the value of x, demonstrating the power and usefulness of pointers in the Go language.
Here is a visual representation of how pointers work:
Deeply explore pointers in Go language
To declare a pointer in Go language, the syntax is var p *T
, where T represents the type of variable that the pointer will reference. Consider the following example, where p is a pointer to an int variable:
1 2 |
|
Here, p stores the address of a, and through pointer dereference (*p), the value of a can be accessed or modified. This mechanism is the basis for efficient data manipulation and memory management in the Go language.
Let’s look at a basic example:
1 2 3 4 5 6 7 8 9 10 11 |
|
Output
1 2 3 4 5 |
|
Pointers in Go language are different from pointers in C/C
A common misunderstanding about when to use pointers in Go stems from comparing pointers in Go directly to pointers in C. Understanding the difference between the two allows you to grasp how pointers work in each language's ecosystem. Let’s dive into these differences:
- No pointer arithmetic
Unlike C language, pointer arithmetic in C language allows direct manipulation of memory addresses, while Go language does not support pointer arithmetic. This deliberate design choice of the Go language leads to several significant advantages:
- Prevent buffer overflow vulnerabilities: By eliminating pointer arithmetic, the Go language fundamentally reduces the risk of buffer overflow vulnerabilities, which are a common security problem in C language programs. Allows attackers to execute arbitrary code.
- Make code safer and easier to maintain: Without the complexity of direct memory operations, Go language code is easier to understand, safer, and easier to maintain. Developers can focus on the application's logic rather than the complexities of memory management.
- Reduce memory-related errors: Eliminating pointer arithmetic minimizes common pitfalls such as memory leaks and segfaults, making Go programs more robust and stable.
- Simplified garbage collection: The Go language's approach to pointers and memory management simplifies garbage collection because the compiler and runtime have a clearer understanding of object life cycles and memory usage patterns. This simplification leads to more efficient garbage collection, thus improving performance.
By eliminating pointer arithmetic, the Go language prevents the misuse of pointers, resulting in more reliable and easier to maintain code.
- Memory management and dangling pointers
In Go language, memory management is much simpler than in languages like C due to its garbage collector.
1 2 |
|
- No need for manual memory allocation/release: The Go language abstracts the complexities of memory allocation and deallocation through its garbage collector, simplifying programming and minimizing errors.
- No dangling pointers: A dangling pointer is a pointer that occurs when the memory address referenced by the pointer is freed or reallocated without updating the pointer. Dangling pointers are a common source of errors in manual memory management systems. Go's garbage collector ensures that an object is only cleaned when there are no existing references to it, effectively preventing dangling pointers.
- Prevent memory leaks: Memory leaks, often caused by forgetting to release memory that is no longer needed, have been significantly mitigated in the Go language. While in Go, objects with reachable pointers are not freed, preventing leaks due to lost references, in C, programmers must diligently manage memory manually to avoid such problems.
- Null pointer behavior
In Go language, trying to dereference a null pointer will cause panic. This behavior requires developers to carefully handle all possible null reference situations and avoid accidental modifications. While this may increase the overhead of code maintenance and debugging, it can also serve as a safety measure against certain types of errors:
1 2 3 4 5 6 7 8 9 10 11 |
|
The output indicates a panic due to an invalid memory address or null pointer dereference:
1 2 3 4 5 |
|
Because student is a null pointer and is not associated with any valid memory address, trying to access its fields (Name and Age) will cause a runtime panic.
In contrast, in C language, dereferencing a null pointer is considered unsafe. Uninitialized pointers in C point to random (undefined) parts of memory, which makes them even more dangerous. Dereferencing such an undefined pointer can mean that the program continues to run with corrupted data, leading to unpredictable behavior, data corruption, or even worse results.
This approach does have its trade-offs - it results in a Go compiler that is more complex than a C compiler. As a result, this complexity can sometimes make Go programs appear to execute slower than their C counterparts.
- Common misconception: “Pointers are always faster”
A common belief is that leveraging pointers can improve the speed of an application by minimizing data copies. This concept stems from the architecture of Go as a garbage-collected language. When a pointer is passed to a function, the Go language performs escape analysis to determine whether the associated variable should reside on the stack or be allocated on the heap. While important, this process introduces a level of overhead. Additionally, if the results of the analysis decide to allocate heap for a variable, more time will be consumed in the garbage collection (GC) cycle. This dynamic illustrates that while pointers reduce direct data copies, their impact on performance is subtle and affected by the underlying mechanisms of memory management and garbage collection in the Go language.
Escape Analysis
The Go language uses escape analysis to determine the dynamic range of values in its environment. This process is an integral part of how the Go language manages memory allocation and optimization. Its core goal is to allocate Go values within function stack frames whenever possible. The Go compiler takes on the task of determining in advance which memory allocations can be safely freed, and subsequently issues machine instructions to handle this cleanup process efficiently.
The compiler performs static code analysis to determine whether a value should be allocated on the stack frame of the function that constructed it, or whether it must "escape" to the heap. It is important to note that the Go language does not provide any specific keywords or functions that allow developers to explicitly direct this behavior. Rather, it is the conventions and patterns in the way the code is written that influence this decision-making process.
Values can escape into the heap for a number of reasons. If the compiler cannot determine the size of the variable, if the variable is too large to fit on the stack, or if the compiler cannot reliably tell whether the variable will be used after the function ends, the value is likely to be allocated on the heap. Additionally, if the function stack frame becomes stale, this may also trigger values to escape into the heap.
But, can we finally determine whether the value is stored on the heap or the stack? The reality is that only the compiler has complete knowledge of where a value ends up being stored at any given time.
Whenever a value is shared outside the immediate scope of a function's stack frame, it will be allocated on the heap. This is where escape analysis algorithms come into play, identifying these scenarios to ensure that the program maintains its integrity. This integrity is critical to maintaining accurate, consistent, and efficient access to any value in the program. Escape analysis is therefore a fundamental aspect of the Go language's approach to memory management, optimizing the performance and safety of executed code.
Check out this example to understand the basic mechanism behind escape analysis:
1 2 |
|
The//go:noinline directive prevents these functions from being inlined, ensuring that our example shows clear calls for escape analysis illustration purposes.
We define two functions, createStudent1 and createStudent2, to demonstrate the different results of escape analysis. Both versions attempt to create user instances, but they differ in their return type and how they handle memory.
- createStudent1: value semantics
In createStudent1, create the student instance and return it by value. This means that when the function returns, a copy of st is created and passed up the call stack. The Go compiler determines that &st does not escape to the heap in this case. This value exists on createStudent1's stack frame and a copy is created for main's stack frame.
Figure 1 – Value Semantics 2. createStudent2: pointer semantics
In contrast, createStudent2 returns a pointer to the student instance, designed to share the student value across stack frames. This situation emphasizes the critical role of escape analysis. If not managed properly, shared pointers run the risk of accessing invalid memory.
If the situation described in Figure 2 did occur, it would pose a significant integrity issue. The pointer will point to memory in the expired call stack. Subsequent function calls to main will cause the memory previously pointed to to be reallocated and reinitialized.
Figure 2 – Pointer semantics
Here, escape analysis steps in to maintain the integrity of the system. Given this situation, the compiler determines that it is unsafe to allocate the student value within the stack frame of createStudent2. Therefore, it chooses to allocate this value on the heap instead, which is a decision made at construction time.
A function can directly access memory within its own frame through the frame pointer. However, accessing memory outside its frame requires indirection through pointers. This means that values destined to escape to the heap will also be accessed indirectly.
In the Go language, the process of constructing a value does not inherently indicate the location of the value in memory. Only when executing the return statement does it become obvious that the value must escape to the heap.
Thus, after the execution of such a function, the stack can be conceptualized in a way that reflects these dynamics.
After the function call, the stack can be visualized as shown below.
The st variable on the stack frame of createStudent2 represents a value that is located on the heap instead of the stack. This means that accessing a value using st requires pointer access, rather than direct access as the syntax suggests.
To understand the compiler's decisions regarding memory allocation, you can request a detailed report. This can be achieved by using the -gcflags switch with the -m option in the go build command.
1 2 |
|
Consider the output of this command:
1 2 3 4 5 6 7 8 9 10 11 |
|
This output shows the results of the compiler's escape analysis. Here’s the breakdown:
- The compiler reports that it cannot inline some functions (createUser1, createUser2 and main) due to a specific directive (go:noinline) or because they are non-leaf functions.
- For createUser1, the output shows that the reference to st within the function does not escape to the heap. This means that the object's lifetime is limited to the stack frame of the function. Instead, during createUser2, it states that &st escapes to the heap. This is clearly related to the return statement, which causes the variable u allocated inside the function to be moved into heap memory. This is necessary because the function returns a reference to st, which needs to exist outside the scope of the function.
Garbage Collection
The Go language includes a built-in garbage collection mechanism that automatically handles memory allocation and release, in sharp contrast to languages such as C/C that require manual memory management. While garbage collection relieves developers from the complexity of memory management, it introduces latency as a trade-off.
A notable feature of the Go language is that passing pointers may be slower than passing values directly. This behavior is due to the nature of Go as a garbage collected language. Whenever a pointer is passed to a function, the Go language performs escape analysis to determine whether the variable should reside on the heap or the stack. This process incurs overhead, and variables allocated on the heap can further exacerbate latency during garbage collection cycles. In contrast, variables restricted to the stack bypass the garbage collector entirely, benefiting from simple and efficient push/pop operations associated with stack memory management.
Memory management on the stack is inherently faster because it has a simple access pattern where memory allocation and deallocation is done simply by incrementing or decrementing a pointer or integer. In contrast, heap memory management involves more complex bookkeeping for allocation and deallocation.
When to use pointers in Go
-
Copy large structures
Although pointers may appear to be less performant due to the overhead of garbage collection, they have advantages in large structures. In this case, the efficiency gained from avoiding copying large data sets may outweigh the overhead introduced by garbage collection. -
Variability
To change a variable passed to a function, a pointer must be passed. The default pass-by-value approach means that any modifications are made on the copy and therefore do not affect the original variable in the calling function. -
API consistency
Using pointer receivers consistently throughout the API keeps it consistent, which is especially useful if at least one method requires a pointer receiver to mutate a struct.
Why do I prefer value?
I prefer passing values rather than pointers, based on a few key arguments:
-
Fixed size type
We consider here types such as integers, floating point numbers, small structures, and arrays. These types maintain a consistent memory footprint that is typically the same as or smaller than the size of a pointer on many systems. Using values for these smaller, fixed-size data types is both memory efficient and consistent with best practices for minimizing overhead. -
Immutability
Passing by value ensures that the receiving function gets an independent copy of the data. This feature is crucial to avoid unintended side effects; any modifications made within a function remain local, preserving the original data outside the scope of the function. Therefore, the call-by-value mechanism acts as a protective barrier, ensuring data integrity. -
Performance advantages of passing values
Despite the potential concerns, passing a value is often fast in many cases and can outperform using pointers in many cases:- Data copy efficiency: For small data, the copy behavior may be more efficient than handling pointer indirection. Direct access to data reduces the latency of extra memory dereferencing that typically occurs when using pointers.
- Reduced load on the garbage collector: Passing values directly reduces the load on the garbage collector. With fewer pointers to keep track of, the garbage collection process becomes more streamlined, improving overall performance.
- Memory locality: Data passed by value is usually stored contiguously in memory. This arrangement benefits the processor's caching mechanism, allowing faster access to data due to an increased cache hit rate. The spatial locality of value-based direct data access facilitates significant performance improvements, especially in computationally intensive operations.
Conclusion
In summary, pointers in Go language provide direct memory address access, which not only improves efficiency but also increases the flexibility of programming patterns, thereby facilitating data manipulation and optimization. Unlike pointer arithmetic in C, Go's approach to pointers is designed to enhance safety and maintainability, which is crucially supported by its built-in garbage collection system. Although the understanding and use of pointers and values in Go language will profoundly affect the performance and security of applications, the design of Go language fundamentally guides developers to make wise and effective choices. Through mechanisms such as escape analysis, the Go language ensures optimal memory management, balancing the power of pointers with the safety and simplicity of value semantics. This careful balance allows developers to create robust, efficient Go applications and clearly understand when and how to take advantage of pointers.
The above is the detailed content of Mastering Pointers in Go: Enhancing Safety, Performance, and Code Maintainability. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










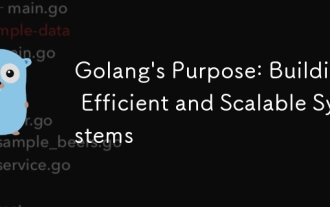
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
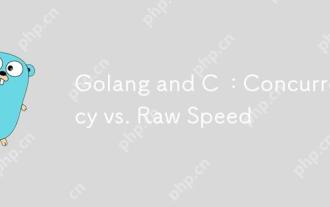
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
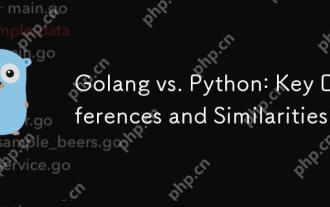
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
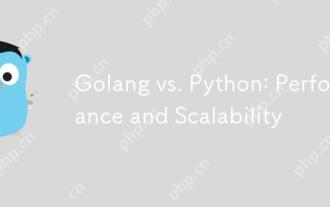
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
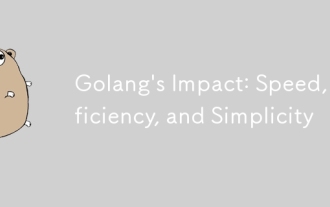
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
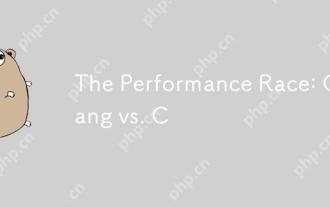
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
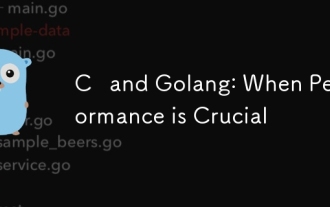
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
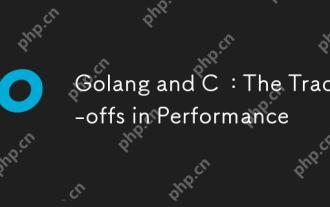
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
