What's the Most Elegant Way to Generate a List of Prime Numbers?
Elegant way to generate prime numbers
This article explores how to generate a list of prime numbers in the most elegant way. An elegant algorithm should be clear, concise and efficient.
Improved Sieve of Eratosthenes
One method is to improve the sieve of Eratosthenes. The following is an elegant Java implementation:
public static ArrayList<Integer> generatePrimes(int n) { ArrayList<Integer> primes = new ArrayList<>(); boolean[] isPrime = new boolean[n + 1]; Arrays.fill(isPrime, true); isPrime[0] = isPrime[1] = false; for (int i = 2; i * i <= n; i++) { if (isPrime[i]) { for (int j = i * i; j <= n; j += i) { isPrime[j] = false; } } } for (int i = 2; i <= n; i++) { if (isPrime[i]) { primes.add(i); } } return primes; }
This algorithm efficiently identifies prime numbers less than or equal to n by iteratively removing multiples of the found prime numbers, ensuring accuracy and efficiency.
Other elegant solutions
In addition to the improved sieving method, the following methods can also be considered:
- LINQ-based generation: Use the lazy loading feature of LINQ to elegantly generate prime number sequences. (This part requires specific code examples to be clearer)
- BigInteger method: Using Java's BigInteger class and nextProbablePrime method can achieve concise and efficient code. (This part requires specific code examples to be clearer)
- Prime number data source: Read directly from pre-generated prime number files or databases, fast and reliable.
Choose the most appropriate approach to building elegant prime number generation algorithms based on your specific needs and preferences for efficiency, simplicity, and readability.
The above is the detailed content of What's the Most Elegant Way to Generate a List of Prime Numbers?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


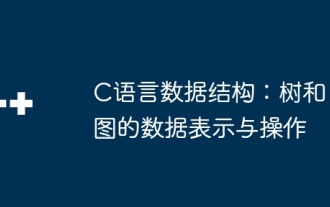
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
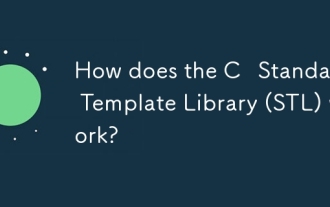
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
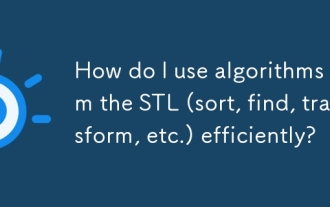
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
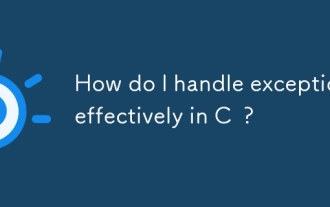
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
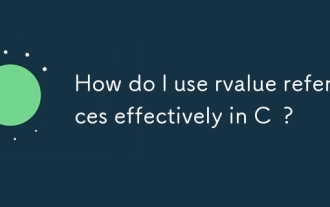
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
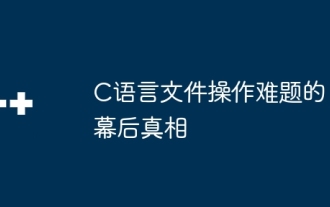
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
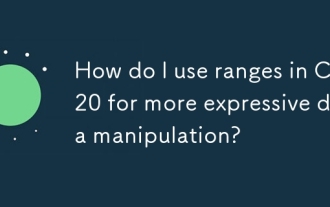
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
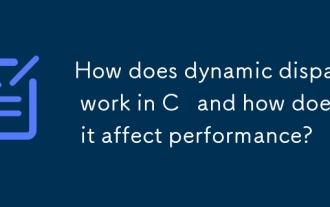
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
