


Creating Custom Inputs for Regex Validation in React and React Native
Validation is a key aspect of form handling, ensuring that user input adheres to specific rules. In this article, we'll explore how to create reusable custom input components with regex validation for React and React Native, using examples like phone numbers, credit cards, and CVCs.
Table of Contents
- Why Custom Inputs?
- Getting Started
- Custom Input Component
- Adding Regex Validation with zod
- Building the Form
- Conclusion
Links
- Source Code
- Live Demo
Why Custom Inputs?
Using custom inputs lets you:
- Standardize validation logic across forms.
- Enhance the user experience with input masks and clear error messages.
- Reuse components in both React and React Native.
Getting Started
Prerequisites
Make sure you have the following dependencies installed:
- react-hook-form for form handling.
- zod and @hookform/resolvers/zod for schema-based validation.
- react-native-mask-input for masked inputs in React Native.
- styled-components for styling.
npm install react-hook-form zod @hookform/resolvers zod react-native-mask-input styled-components
Custom Input Component
Here is the reusable StyledInput component:
Code for React Native
import React from "react"; import { Controller, FieldPath, FieldValues, useFormContext, } from "react-hook-form"; import { TextInputProps, View } from "react-native"; import styled from "styled-components/native"; const InputContainer = styled.View` width: 100%; `; const Label = styled.Text` font-size: 16px; color: ${({ theme }) => theme.colors.primary}; `; const InputBase = styled.TextInput` flex: 1; font-size: ${({ theme }) => `${theme.fontSizes.base}px`}; color: ${({ theme }) => theme.colors.primary}; height: ${({ theme }) => `${theme.inputSizes.base}px`}; border: 1px solid ${({ theme }) => theme.colors.border}; border-radius: 8px; padding: 8px; `; const ErrorMessage = styled.Text` font-size: 12px; color: ${({ theme }) => theme.colors.error}; `; interface StyledInputProps<TFieldValues extends FieldValues> extends TextInputProps { label: string; name: FieldPath<TFieldValues>; } export function StyledInput<TFieldValues extends FieldValues>({ label, name, ...inputProps }: StyledInputProps<TFieldValues>) { const { control, formState } = useFormContext<TFieldValues>(); const { errors } = formState; const errorMessage = errors[name]?.message as string | undefined; return ( <InputContainer> <Label>{label}</Label> <Controller control={control} name={name} render={({ field: { onChange, onBlur, value } }) => ( <InputBase onBlur={onBlur} onChangeText={onChange} value={value} {...inputProps} /> )} /> {errorMessage && <ErrorMessage>{errorMessage}</ErrorMessage>} </InputContainer> ); }
Code for React
The same logic applies, but replace TextInput with HTML's and style it accordingly.
Adding Regex Validation with zod
Define masks and validators for inputs like phone numbers and credit cards:
import * as zod from "zod"; import { Mask } from "react-native-mask-input"; const turkishPhone = { mask: [ "+", "(", "9", "0", ")", " ", /\d/, /\d/, /\d/, " ", /\d/, /\d/, /\d/, "-", /\d/, /\d/, "-", /\d/, /\d/, ], validator: /^\+\(90\) \d{3} \d{3}-\d{2}-\d{2}$/, placeholder: "+(90) 555 555-55-55", }; const creditCard = { mask: [ /\d/, /\d/, /\d/, /\d/, "-", /\d/, /\d/, /\d/, /\d/, "-", /\d/, /\d/, /\d/, /\d/, "-", /\d/, /\d/, /\d/, /\d/, ], validator: /^\d{4}-\d{4}-\d{4}-\d{4}$/, placeholder: "4242-4242-4242-4242", }; const cvc = { mask: [/\d/, /\d/, /\d/], validator: /^\d{3}$/, placeholder: "123", }; const schema = zod.object({ phone: zod.string().regex(turkishPhone.validator, "Invalid phone number"), creditCard: zod .string() .regex(creditCard.validator, "Invalid credit card number"), cvc: zod.string().regex(cvc.validator, "Invalid CVC"), });
Building the Form
Use react-hook-form with zod for validation:
import { FormProvider, useForm } from "react-hook-form"; import { zodResolver } from "@hookform/resolvers/zod"; export default function FormScreen() { const form = useForm({ resolver: zodResolver(schema), mode: "onBlur", }); return ( <FormProvider {...form}> <StyledInput name="phone" label="Phone" placeholder={turkishPhone.placeholder} /> <StyledInput name="creditCard" label="Credit Card" placeholder={creditCard.placeholder} /> <StyledInput name="cvc" label="CVC" placeholder={cvc.placeholder} /> <button type="submit">Submit</button> </FormProvider> ); }
Conclusion
By creating reusable custom inputs with regex validation, you can simplify form handling and maintain consistency across your projects. These components work seamlessly in both React and React Native, providing a great user experience.
Feel free to customize the masks, validators, and styles to suit your application's requirements. Happy coding!
The above is the detailed content of Creating Custom Inputs for Regex Validation in React and React Native. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










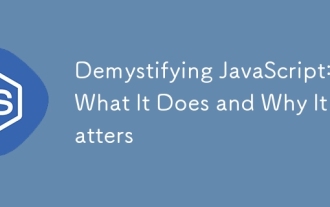
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
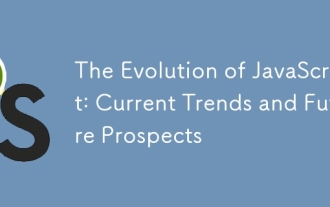
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
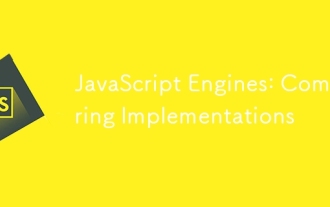
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
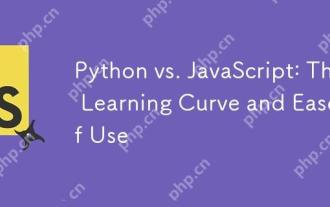
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
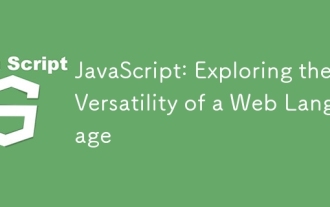
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
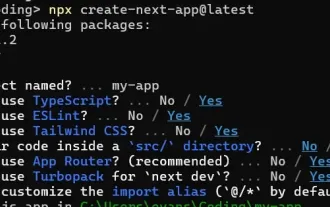
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
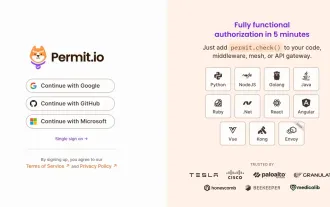
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
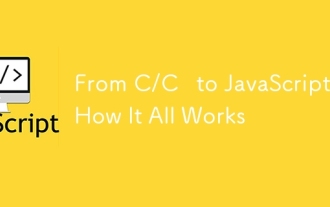
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
