How to Implement Authentication in React Using JWT (JSON Web Tokens)
Authentication is a critical part of many web applications, and securing routes in React requires an understanding of how tokens, such as JSON Web Tokens (JWT), work. JWT allows you to securely transmit information between the client and the server, which is particularly useful for implementing user authentication in modern web applications. In this article, we’ll learn how to implement authentication in React using JWT.
What is JWT?
JSON Web Tokens (JWT) are compact, URL-safe tokens used to represent claims between two parties. JWT is a popular choice for authentication in single-page applications (SPAs) because it is lightweight, stateless, and easy to implement.
A typical JWT consists of three parts:
1. Header: Contains metadata about the token, including the signing algorithm.
2. Payload: Contains the claims (data) that you want to transmit, such as user information.
3. Signature: Used to verify that the sender is who it says it is and to ensure that the message wasn’t changed along the way.
Steps to Implement JWT Authentication in React
1. Backend Setup (Node.js Express JWT)
- Install required dependencies: express, jsonwebtoken, bcryptjs.
- Set up a simple Express server and create routes for login and protected data access.
- Generate a JWT token upon successful login. Example backend code (Node.js Express JWT):
const express = require('express'); const jwt = require('jsonwebtoken'); const bcrypt = require('bcryptjs'); const app = express(); const users = [{ username: 'test', password: 'a$...' }]; // Example user data app.use(express.json()); app.post('/login', (req, res) => { const { username, password } = req.body; // Verify user credentials const user = users.find(u => u.username === username); if (user && bcrypt.compareSync(password, user.password)) { const token = jwt.sign({ username: user.username }, 'secretKey', { expiresIn: '1h' }); return res.json({ token }); } else { return res.status(401).send('Invalid credentials'); } }); app.listen(5000, () => console.log('Server running on port 5000'));
2. Frontend Setup (React JWT):
- Use React’s useState and useEffect hooks to manage authentication state.
- Store the JWT token in local storage or cookies after successful login.
- Use the JWT token to make authenticated requests to the backend API. Example frontend code (React JWT):
import React, { useState } from 'react'; import axios from 'axios'; function Login() { const [username, setUsername] = useState(''); const [password, setPassword] = useState(''); const handleLogin = async (e) => { e.preventDefault(); try { const response = await axios.post('http://localhost:5000/login', { username, password }); localStorage.setItem('token', response.data.token); // Store the JWT alert('Login successful'); } catch (error) { alert('Login failed'); } }; return ( <form onSubmit={handleLogin}> <input type="text" value={username} onChange={(e) => setUsername(e.target.value)} /> <input type="password" value={password} onChange={(e) => setPassword(e.target.value)} /> <button type="submit">Login</button> </form> ); } function Dashboard() { const [data, setData] = useState([]); useEffect(() => { const token = localStorage.getItem('token'); if (token) { axios.get('http://localhost:5000/protected', { headers: { Authorization: `Bearer ${token}` } }) .then(response => setData(response.data)) .catch(error => console.error(error)); } }, []); return ( <div> <h1>Protected Dashboard</h1> <pre class="brush:php;toolbar:false">{JSON.stringify(data, null, 2)}
3. Protecting Routes:
- Use React Router to create private routes that require authentication.
- Check if the JWT token exists before allowing access to protected routes. Example:
const PrivateRoute = ({ component: Component, ...rest }) => { const token = localStorage.getItem('token'); return ( <Route {...rest} render={props => token ? <Component {...props} /> : <Redirect to="/login" />} /> ); };
Conclusion
Implementing JWT authentication in React is a straightforward process that involves creating a secure backend, generating a token on successful login, and managing the authentication state in React. JWT provides a scalable and stateless way of managing authentication in modern web applications.
The above is the detailed content of How to Implement Authentication in React Using JWT (JSON Web Tokens). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










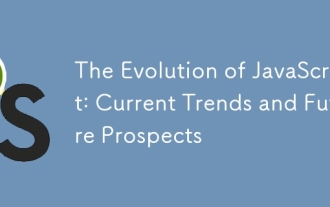
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
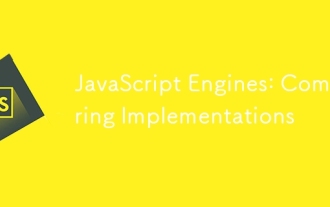
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
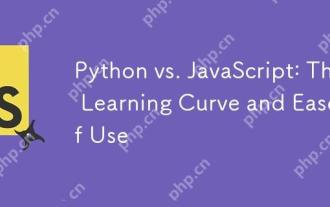
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
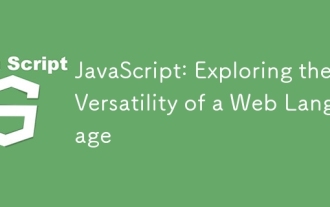
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
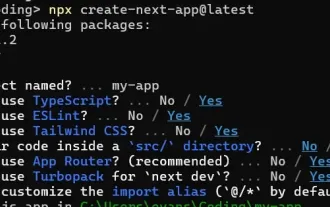
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
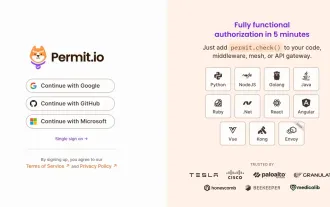
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
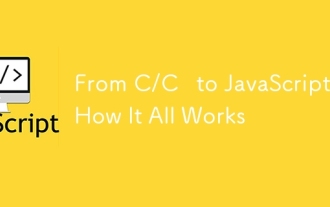
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
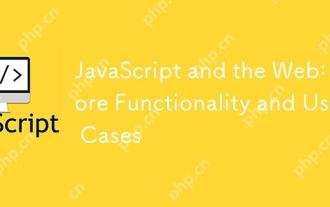
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
