Understanding the Styles of APIs in Vue.js
Vue is a JavaScript framework for building user interfaces. It builds on top of standard HTML, CSS, and JavaScript and provides a declarative, component-based programming model that helps you efficiently develop user interfaces of any complexity.
Vue components can be authored in two API styles: Options API and Composition API(Introduced above version 2.6), Both approaches have their unique benefits and drawbacks, and choosing the right one for your project can be a difficult decision.
Let’s dive deep into both styles of understanding
Options API:
The Options API is the traditional way of building Vue components. It defines a component's behavior and state using a set of options like data, methods, and computed properties.
data(): This function returns an object containing the component's reactive data properties. These properties will update the component's rendered output when their values change.
methods(): This function returns an object containing methods (functions) that can be used inside the component's template or other methods. These methods can manipulate the data or perform actions.
computed properties: These functions return a value based on the component's data. They are recalculated whenever any of their dependencies (reactive data properties) change.
One of the main benefits of the Options API is that it is simple and easy to understand. It follows a clear, declarative pattern that is familiar to many developers, and it is well-documented in the Vue documentation. This makes it a good choice for beginners who are just starting with Vue.
However, the Options API has some limitations that can make it difficult to use for more complex projects.
Another limitation of the Options API is that it can be inflexible when it comes to sharing logic between components.
Unit testing components built with the Options API can be more challenging. The spread of logic across different options makes it harder to isolate specific functionalities for testing.
Let's see the Example of Options API styling :
<template> <div> <h4>{{ name }}'s To Do List</h4> <div> <input v-model="newItemText" v-on:keyup.enter="addNewTodo" /> <button v-on:click="addNewTodo">Add</button> <button v-on:click="removeTodo">Remove</button> </div> <ul> <li v-for="task in tasks" v-bind:key="task">{{ task }}</li> </ul> </div> </template> <script> export default { data() { return { name: "John", tasks: ["Buy groceries", "Clean the house"], newItemText: "", }; }, methods: { addNewTodo() { if (this.newItemText !== "") { this.tasks.push(this.newItemText); this.newItemText = ""; } }, removeTodo(index) { this.tasks.splice(index, 1); }, }, }; </script>
- Composition API :
The Composition API is a set of tools introduced in Vue 3 (and available for Vue 2 through a plugin) that provides an alternative way to write component logic compared to the traditional Options API. It focuses on composing reusable functions to manage a component’s state and behavior.
With Composition API, we define a component’s logic using imported API functions. It also allows developers to use the full power of JavaScript to define component behavior.
Composition API is typically used with . The setup attribute is a hint that makes Vue perform compile-time transforms that allow us to use Composition API with less boilerplate.
The foundation of Composition API is Vue’s built-in reactivity system. Functions like ref and reactive create reactive data that automatically updates the component when it changes. This simplifies state management compared to manually setting up getters and setters in the Options API.
The Composition API supports dependency injection through functions like provide and inject.
The Composition API is particularly beneficial for:
Building complex and reusable components
Projects that prioritize code organization and maintainability
Applications that leverage TypeScript for type safety
The Composition API may seem like the best option to use. However, the Composition API is not without its drawbacks. One issue is that it can be more difficult to learn for developers who are not familiar with functional, reactive programming.
Another issue is that the Composition API is not backward compatible with Vue 2.6 and under by default. This means you will need to either upgrade to Vue 3.0 or import the Composition API via a plugin.
Let’s see the Example of Composition API styling :
The above is the detailed content of Understanding the Styles of APIs in Vue.js. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










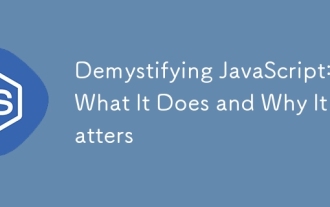
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
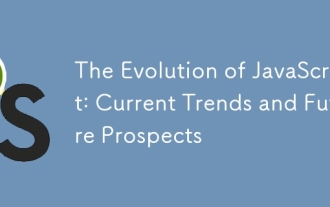
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
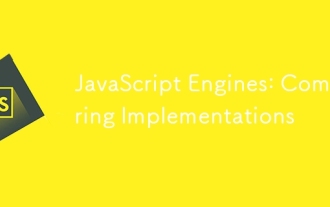
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
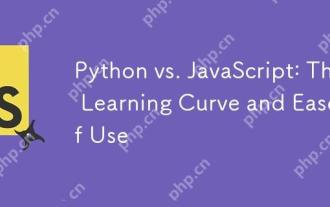
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
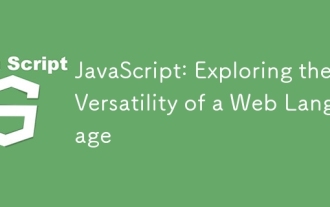
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
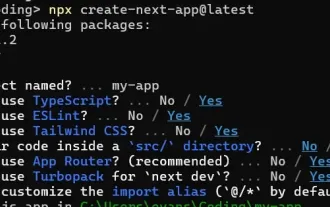
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
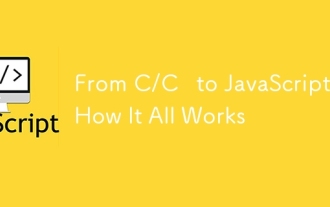
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
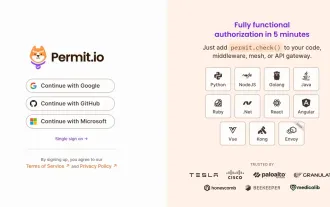
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
