React New API use(promise)
In this post, I’ll demo how to read a value from a promise using use.
Links
Demo
Codebase
Snippet
Let’s check out the following code:
import { Suspense } from "react"; export default function Page() { const messagePromise = fetchMessages(); return ( <Suspense fallback={<p>⌛ waiting for messages...</p>}> <Message messagePromise={messagePromise} /> </Suspense> ); }
A couple of things to note:
is being used, which basically displays the fallback, in this case: ⌛ waiting for messages..., until the promise is resolved. messagePromise is a promise passed as a prop to
.
Now, let’s take a look at fetchMessages:
async function fetchMessages() { return [ { id: 1, text: "message 1", }, { id: 2, text: "message 2", }, ]; }
As you can see, it’s a very simple function. In a real world example, this could be a fetch request, but for simplicity, the function just returns an array. By using async, JavaScript automatically knows that the function returns a promise.
Finally, let’s check out the
function Message({ messagePromise }) { const comments = use(messagePromise); return comments.map((comment) => <p key={comment.id}>{comment.text}</p>); }
This is where it gets interesting. The
Usually, you’d use await with a promise, but in React 19, you can now use use to achieve basically the same result.
One key difference between await and use is that await blocks rendering until the promise is resolved, whereas use doesn’t block rendering.
Here’s how the component looks before the promise is resolved:
And once the promise is resolved:
Conclusion
use works similarly to await, and if the application is running on a server using SSR, the result is exactly the same: the server will return the same HTML response in both cases.
However, if the code runs on the client, rendering behaves a bit differently. await blocks rendering until the promise is resolved, while use allows the component to re-render once the promise is resolved.
The above is the detailed content of React New API use(promise). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










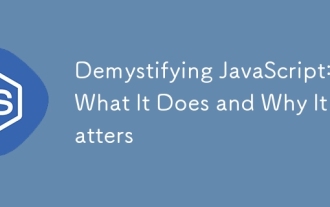
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
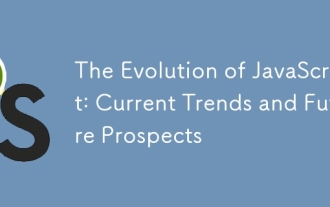
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
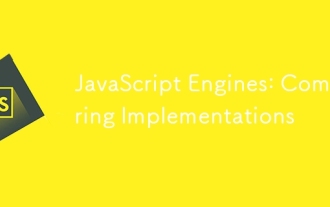
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
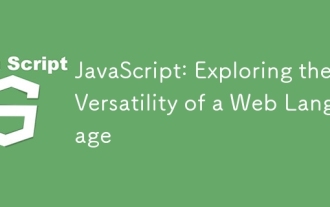
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
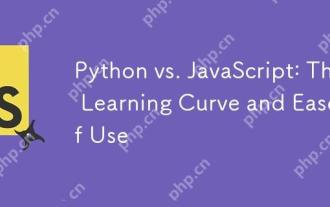
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
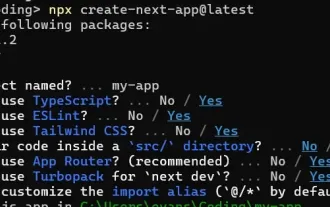
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
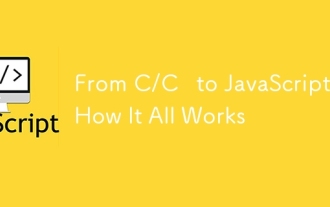
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
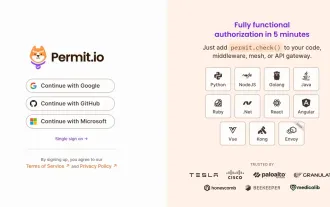
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
