Understanding Scopes in JavaScript
The concepts of scopes and closures in Javascript are foundational blocks necessary for mastery of the language. They are the unsung heroes behind Constructors, Factory functions, and IIFEs, to name a few.
This article aims to explain scope in JavaScript using practical examples. In a subsequent article, we will discuss JavaScript closures.
Scope and lexical scoping
Scope simply determines where a variable will be available for use in a JavaScript program. Fundamentally, there are two types of scope:
- Global Scope
- Local Scope
Global Scope
With global scope, a variable is made available everywhere and can be used anywhere within the program. Technically, when a variable is not declared within any function or { curly braces }, they are said to be in the global scope.
Local Scope
On the other hand, with local scope, a variable is only made available in a particular context and can only be used in that context. Technically, when a variable is declared within functions or { curly braces }, they are said to be locally scoped.
let x = 3; // x (global scoped) function addXY () { let y = 5; // y (locally scoped) return x + y; // returns 8 since x is available anywhere in this program }
More Scoopful of Scope
ECMAScript is the standardized specification that defines the core features of scripting languages like JavaScript, ensuring consistency and interoperability across platforms. Just as the International System of Units (SI) provides a standardized framework for measurements like meters and kilograms to ensure consistency worldwide, ECMAScript sets the standards for scripting languages like JavaScript, ensuring they work uniformly across different platforms and environments.
This standardized specification for JavaScript has evolved through editions, with new features and improvements added in each version. Among these editions is ES6 (ECMAScript 2015) which provided a major update that introduced let/const.
Before ES6, variables were defined in JavaScript with the var keyword. With var, variables can both be redefined and updated. However, var variables are only locally scoped within functions. The extension of local scope to include snippets within { curly braces } was introduced by ES6 through let/const. Simply put, var variables are locally scoped within functions but globally scoped everywhere else.
let and const introduce block scoping which makes a variable to only be available within the closest set of { curly braces } in which it was defined. These braces can be those of a for loop, if-else condition, or any other similar JavaScript construct. Such braces are also referred to as a code block.
Examples:
function addXYZ() { var x = 3; let y = 4; const z = 5; return x + y + z; // 12 } // These log undefined because var, let, and const variables are locally scoped within functions. console.log(x); console.log(y); console.log(z);
let age = 10; // global variable if (age < 18) { let letCandy = 2; // block-scoped variable console.log(`You are entitled to ${letCandy} candies`); } else { var varCandy = 4; // function-scoped variable console.log(`You are entitled to ${varCandy} candies`); } console.log(age); // Logs 10, as age is globally scoped console.log(letCandy); // Throws ReferenceError, as letCandy is block-scoped console.log(varCandy); // Logs 4, as varCandy is globally scoped outside of functions
In a subsequent article, we will be discussing lexical scoping and closures. Thank you for reading.
The above is the detailed content of Understanding Scopes in JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










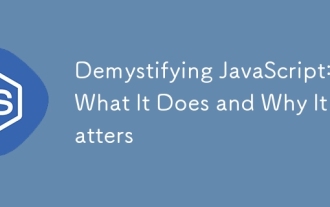
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
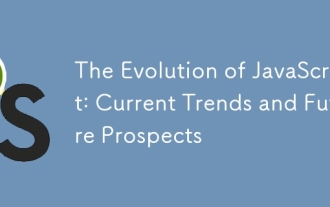
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
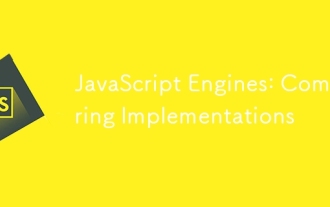
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
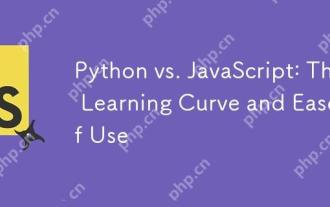
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
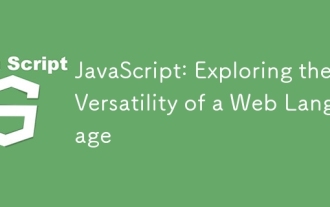
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
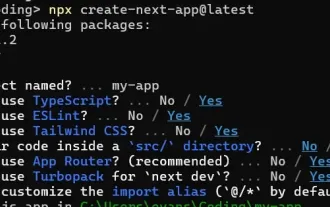
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
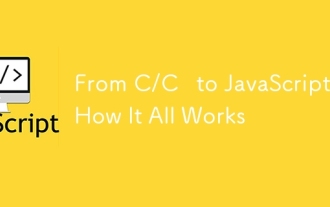
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
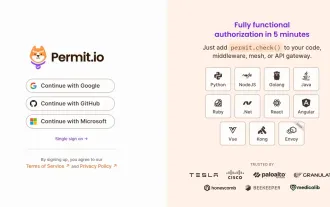
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
