


How Can C# and WMI Automate Application Startup and Shutdown Based on USB Drive Insertion and Removal?
Automating Application Behavior with USB Drive Insertion/Removal: A C# and WMI Solution
Need to automatically start and stop an application when a USB drive is inserted or removed? This guide demonstrates how to achieve this using C# and Windows Management Instrumentation (WMI).
Detecting USB Drive Changes
Several methods exist for detecting USB drive events in C#, including using WndProc
to intercept Windows messages. However, WMI offers a simpler and more robust approach, especially within a service context.
WMI Implementation: A Practical Example
The following code snippet illustrates how to use WMI to monitor USB drive insertion events:
using System.Management; // Create a WMI event watcher ManagementEventWatcher watcher = new ManagementEventWatcher(); // Define the WQL query to monitor volume change events (EventType 2 indicates insertion) WqlEventQuery query = new WqlEventQuery("SELECT * FROM Win32_VolumeChangeEvent WHERE EventType = 2"); // Assign the event handler watcher.EventArrived += watcher_EventArrived; // Set the query for the watcher watcher.Query = query; // Start the watcher watcher.Start(); // Wait for the next event (this can be adapted for continuous monitoring) watcher.WaitForNextEvent(); private void watcher_EventArrived(object sender, EventArrivedEventArgs e) { // Handle USB drive insertion event here }
The watcher_EventArrived
method will be triggered when a USB drive is inserted. Similar logic, modifying the EventType
in the query (EventType 3 represents removal), can be used to detect USB drive removal.
Summary
This combination of C# and WMI provides a reliable and efficient method for creating a Windows service that automatically responds to USB drive insertion and removal. This capability is highly valuable for various application scenarios requiring dynamic behavior based on USB drive presence.
The above is the detailed content of How Can C# and WMI Automate Application Startup and Shutdown Based on USB Drive Insertion and Removal?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


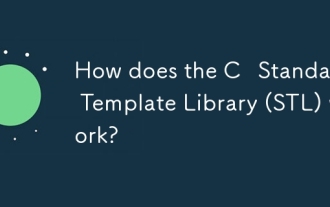
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
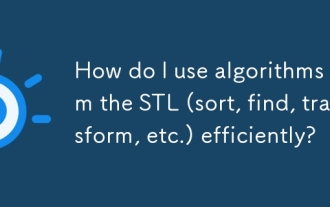
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
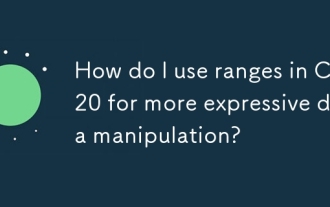
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
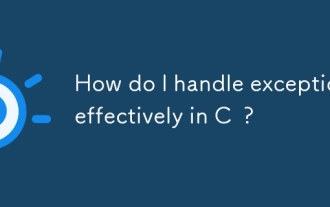
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
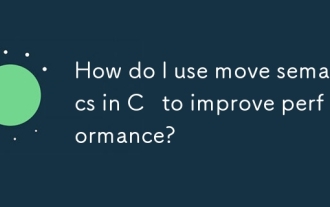
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
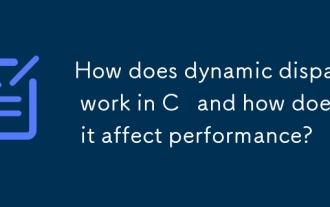
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
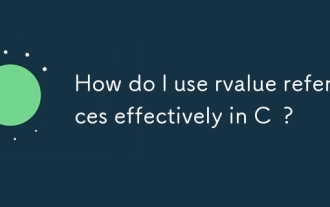
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
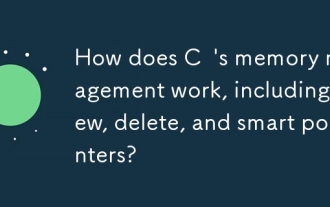
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
