Secrets of Javas String Pool
1. Introduction to Java's String Pool
In Java, strings are a fundamental part of programming. The String Pool, also known as the String Intern Pool, is a special memory area where Java stores string literals to optimize memory usage. This concept is key to understanding how Java manages string data efficiently.
1.1 What is the String Pool?
The String Pool is a collection of unique string literals stored in the Java heap memory. When a string literal is created, Java checks if an identical string already exists in the pool. If it does, Java reuses the existing string reference instead of creating a new one. This approach helps in saving memory and improving performance by reducing the number of string objects created.
1.2 How Does the String Pool Work?
String Literal Pool : This is a special area in memory where string literal objects are stored. When you use the syntax "string", that string is stored in the String Pool. String literals like "Hello" and "World" are stored here.
Heap Memory : Strings created using the new String("string") syntax are not stored in the String Pool but rather in heap memory. However, if you call the .intern() method on a string object, Java will check the String Pool and return the string object from the pool if it exists.
2. Benefits of the String Pool
Understanding the advantages of the String Pool can help you write more efficient and optimized Java code. Here’s how it benefits your application:
2.1 Memory Efficiency
By reusing string literals, the String Pool reduces the number of objects created in memory. This efficiency is particularly noticeable when dealing with a large number of identical string values, such as in text-heavy applications or when processing data from external sources.
Example Code:
public class StringPoolExample { public static void main(String[] args) { String str1 = "Java"; String str2 = "Java"; // Check if both references point to the same object System.out.println(str1 == str2); // Output: true } }
In this example, str1 and str2 refer to the same object in the String Pool, demonstrating memory efficiency.
2.2 Performance Improvement
Reusing string literals from the pool can lead to performance improvements. Since strings are immutable, the JVM can optimize string operations and comparisons when using pooled strings. This can reduce the time spent on memory allocation and garbage collection.
Example Code:
public class StringPerformanceExample { public static void main(String[] args) { String str1 = "Performance"; String str2 = new String("Performance").intern(); // Check if both references point to the same object System.out.println(str1 == str2); // Output: true } }
Here, str1 is a literal string that gets pooled, while str2 is explicitly interned to ensure it points to the same reference.
3. Practical Implications
Understanding and leveraging the String Pool can help in various scenarios, especially in applications where memory and performance are critical. Here’s how you can make the most of it:
Avoiding Unnecessary String Creation
Using string literals and the intern() method helps avoid creating redundant string objects. This practice is beneficial in scenarios like configuration management or when handling large datasets.
Optimizing String Comparisons
When comparing strings, especially in performance-critical code, using pooled strings can lead to faster comparisons since they are guaranteed to be unique in the pool.
Example Code:
public class StringPoolExample { public static void main(String[] args) { String str1 = "Java"; String str2 = "Java"; // Check if both references point to the same object System.out.println(str1 == str2); // Output: true } }
In this example, str1 and str2 are compared using the equals() method, ensuring that they are considered equal when referring to the same pooled string.
4. Conclusion
The String Pool is a powerful feature in Java that enhances memory efficiency and performance. By understanding how it works and applying it effectively, you can write more optimized and efficient Java applications. If you have any questions or need further clarification, feel free to leave a comment below!
Read posts more at : Secrets of Java's String Pool
The above is the detailed content of Secrets of Javas String Pool. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










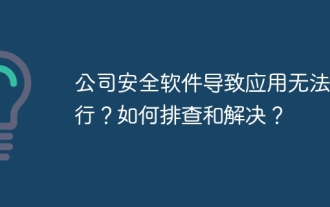
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
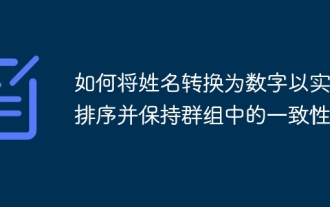
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
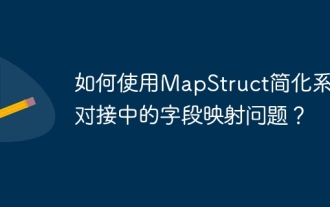
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
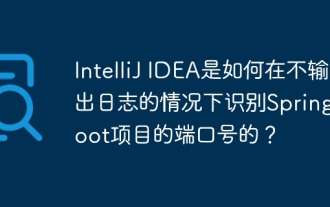
Start Spring using IntelliJIDEAUltimate version...
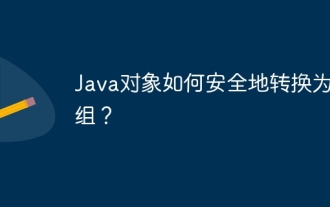
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
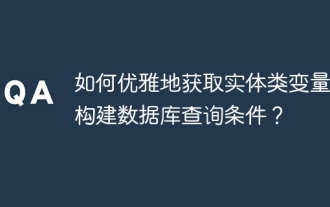
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
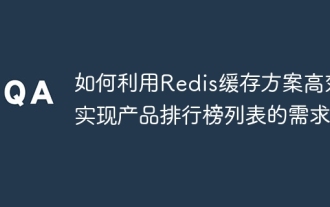
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
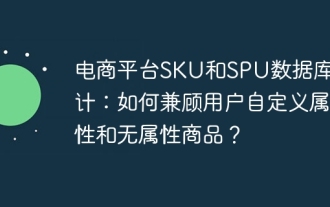
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
