


Step-by-Step Email Verification JavaScript Tutorial: Best Practices & Code Examples
Email verification in JavaScript involves two essential components: client-side format validation and server-side verification through confirmation links. This comprehensive guide provides production-ready code examples and security best practices for implementing a robust email verification system in your applications.
Proper email verification is crucial for maintaining email deliverability and protecting your application from invalid or malicious email submissions. While client-side validation offers immediate user feedback, server-side verification ensures the email actually exists and belongs to the user.
Before diving into the implementation, ensure you have a basic understanding of:
- JavaScript (ES6 )
- Regular expressions
- Node.js (for server-side implementation)
- Basic email protocol concepts
Understanding how email verification works at a fundamental level helps you implement more secure and efficient solutions. Modern email verification typically employs multiple validation layers:
While implementing email validation best practices, it's essential to balance security with user experience. Our implementation will focus on creating a robust system that protects against invalid emails while maintaining a smooth user experience.
Throughout this tutorial, we'll build a complete email verification system that includes:
- Client-side validation using modern JavaScript patterns
- Server-side verification with secure token generation
- Protection against common security vulnerabilities
- Testing strategies for ensuring reliability
The code examples provided are production-ready and follow current security best practices, allowing you to implement them directly in your applications while maintaining flexibility for customization based on your specific needs.
While implementing email validation best practices, it's essential to balance security with user experience. A robust email verification system protects against various threats while maintaining user engagement:
First, client-side validation provides immediate feedback, preventing obvious formatting errors before server submission. This approach reduces server load and improves user experience by catching mistakes early in the process. However, client-side validation alone isn't sufficient for securing your application.
Server-side verification adds crucial security layers by performing deeper validation checks. This includes domain verification and implementing secure confirmation workflows. The combination of both client and server-side validation creates a comprehensive security framework.
Common security challenges you'll need to address include:
- Protection against automated form submissions
- Prevention of email confirmation link exploitation
- Secure token generation and management
- Rate limiting to prevent abuse
When implementing email verification, consider these critical factors that impact your application's security and user experience:
Modern JavaScript frameworks and libraries can significantly streamline the implementation process. However, understanding the underlying principles ensures you can adapt the solution to your specific requirements and improve your marketing campaigns through better email validation.
The implementation approaches we'll explore are designed to scale with your application's growth. Whether you're building a small web application or a large-scale system, these patterns provide a solid foundation for reliable email verification.
By following this tutorial, you'll create a verification system that:
- Validates email format using modern JavaScript techniques
- Implements secure server-side verification
- Handles edge cases and potential security threats
- Provides a smooth user experience
- Scales effectively as your application grows
Let's begin with implementing client-side validation, where we'll explore modern JavaScript patterns for effective email format verification.
Client-Side Email Validation
Client-side email validation provides immediate feedback to users before form submission, enhancing user experience and reducing server load. Let's implement a robust validation system using modern JavaScript practices and proven regex patterns.
RegEx Pattern Validation
The foundation of email validation starts with a reliable regular expression pattern. While no regex pattern can guarantee 100% accuracy, we'll use a pattern that balances validation thoroughness with practical usage:
const emailRegex = /^[a-zA-Z0-9.!#$%&'* /=?^_{|}~-] @[a-zA-Z0-9-] (?:.[a-zA-Z0-9-] )*$/;`
This pattern validates email addresses according to RFC 5322 standards, checking for:
- Valid characters in the local part (before @)
- Presence of a single @ symbol
- Valid domain name structure
- Proper use of dots and special characters
Building the Validation Function
Let's create a comprehensive validation function that not only checks the format but also provides meaningful feedback. This approach aligns with email format best practices:
`function validateEmail(email) {
// Remove leading/trailing whitespace
const trimmedEmail = email.trim();
// Basic structure check if (!trimmedEmail) { return { isValid: false, error: 'Email address is required' }; } // Length validation if (trimmedEmail.length > 254) { return { isValid: false, error: 'Email address is too long' }; } // RegEx validation if (!emailRegex.test(trimmedEmail)) { return { isValid: false, error: 'Please enter a valid email address' }; } // Additional checks for common mistakes if (trimmedEmail.includes('..')) { return { isValid: false, error: 'Invalid email format: consecutive dots not allowed' }; } return { isValid: true, error: null };
}`
Form Integration and Error Handling
Integrate the validation function with your HTML form to provide real-time feedback. This implementation follows current validation best practices:
`document.addEventListener('DOMContentLoaded', () => {
const emailInput = document.getElementById('email');
const errorDisplay = document.getElementById('error-message');
emailInput.addEventListener('input', debounce(function(e) { const result = validateEmail(e.target.value); if (!result.isValid) { errorDisplay.textContent = result.error; emailInput.classList.add('invalid'); emailInput.classList.remove('valid'); } else { errorDisplay.textContent = ''; emailInput.classList.add('valid'); emailInput.classList.remove('invalid'); } }, 300));
});
// Debounce function to prevent excessive validation calls
function debounce(func, wait) {
let timeout;
return function executedFunction(...args) {
const later = () => {
clearTimeout(timeout);
func(...args);
};
clearTimeout(timeout);
timeout = setTimeout(later, wait);
};
}`
Here's the corresponding HTML structure:

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


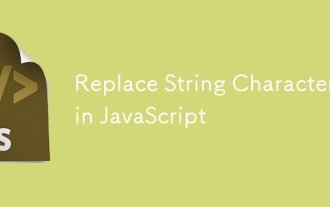
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
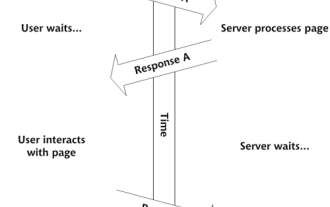
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
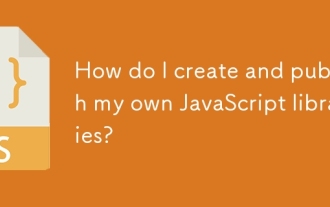
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
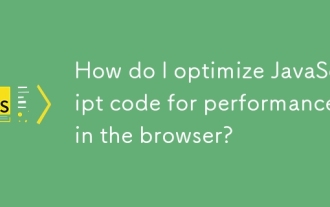
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
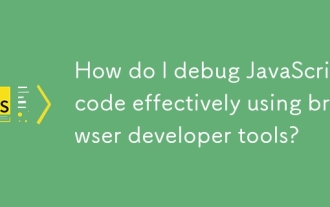
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
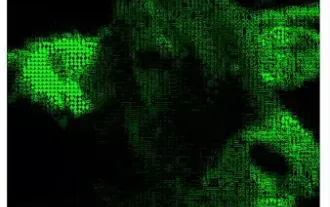
Bring matrix movie effects to your page! This is a cool jQuery plugin based on the famous movie "The Matrix". The plugin simulates the classic green character effects in the movie, and just select a picture and the plugin will convert it into a matrix-style picture filled with numeric characters. Come and try it, it's very interesting! How it works The plugin loads the image onto the canvas and reads the pixel and color values: data = ctx.getImageData(x, y, settings.grainSize, settings.grainSize).data The plugin cleverly reads the rectangular area of the picture and uses jQuery to calculate the average color of each area. Then, use
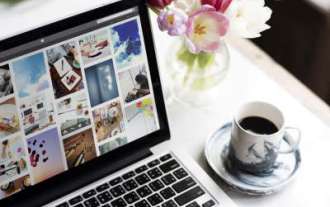
This article will guide you to create a simple picture carousel using the jQuery library. We will use the bxSlider library, which is built on jQuery and provides many configuration options to set up the carousel. Nowadays, picture carousel has become a must-have feature on the website - one picture is better than a thousand words! After deciding to use the picture carousel, the next question is how to create it. First, you need to collect high-quality, high-resolution pictures. Next, you need to create a picture carousel using HTML and some JavaScript code. There are many libraries on the web that can help you create carousels in different ways. We will use the open source bxSlider library. The bxSlider library supports responsive design, so the carousel built with this library can be adapted to any
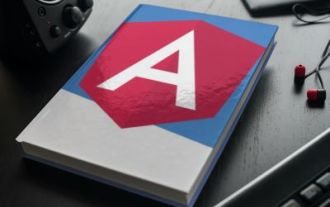
Data sets are extremely essential in building API models and various business processes. This is why importing and exporting CSV is an often-needed functionality.In this tutorial, you will learn how to download and import a CSV file within an Angular
