Builder references
Referencing a constructor uses the syntax: classname::new.
Can be assigned to a functional interface that has a constructor-compatible method.
Example with Parameterized Constructor
- If the functional interface has a method with one parameter, the reference will be associated with the constructor with that parameter.
MyFunc myClassCons = MyClass::new;
MyClass mc = myClassCons.func("Testing");
- Here, MyClass(String s) is the referenced constructor.
Example with Default Constructor
- To reference the parameterless constructor, you must use a functional interface whose method also has no parameters.
MyFunc2 myClassCons = MyClass::new;
MyClass mc = myClassCons.func();
Use with Generic Classes
- For generic classes, you can specify the type when creating the reference.
MyGenClass
- Thanks to type inference, specification is not always mandatory.
Type Inference
- The reference to the constructor automatically selects the one that best suits the functional interface method.
// Demonstrates a constructor reference.
// MyFunc is a functional interface whose method returns
// a MyClass reference.
MyFunc interface {
MyClass func(String s);
}
class MyClass {
private String str;
// This constructor takes one argument.
MyClass(String s) { str = s; }
// This is the default constructor.
MyClass() { str = ""; }
// ...
String getStr() { return str; }
}
class ConstructorRefDemo {
public static void main(String args[])
{
// Creates a reference to the constructor of MyClass.
// Since MyFunc's func() method takes one argument,
// new references the parameterized constructor of MyClass
// and not the default constructor.
MyFunc myClassCons = MyClass::new; A constructor reference
// Creates an instance of MyClass using this constructor reference.
MyClass mc = myClassCons.func("Testing");
// Use the newly created MyClass instance.
System.out.println("str in mc is " mc.getStr());
}
}
The above is the detailed content of Builder references. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










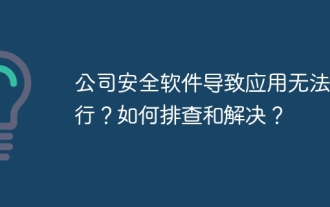
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
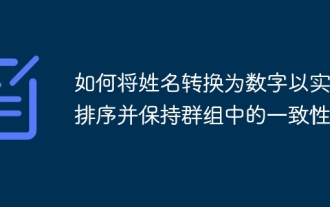
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
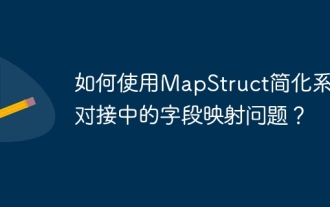
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
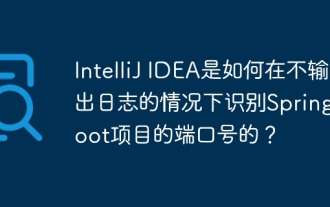
Start Spring using IntelliJIDEAUltimate version...
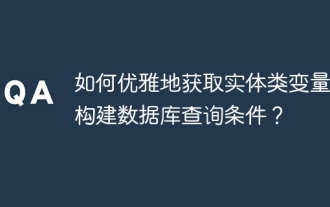
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
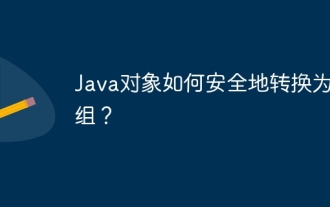
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
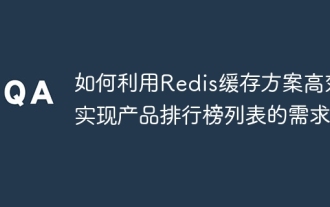
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
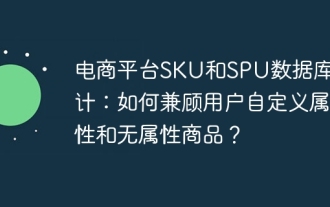
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
