How to Implement Global Exception Handling in WinForms Applications?
Global exception handling in WinForms applications: intercepting unhandled errors
Effective handling of unhandled exceptions in WinForms applications is critical to prevent unexpected app crashes. By implementing a global exception handler, you can log exceptions to the database and ensure that critical functionality is not interrupted.
Question:
In debug mode, using a try/catch block in Program.cs surrounding Application.Run successfully handles the exception. However, when running the application in non-debug mode, the exception is not caught and the user sees an Unhandled Exception dialog box.
Solution:
To enable global exception handling, follow these steps:
-
Add UI thread exception handler:
Application.ThreadException += new ThreadExceptionEventHandler(ErrorHandlerForm.Form1_UIThreadException);
Copy after login -
Set unhandled exception mode:
Application.SetUnhandledExceptionMode(UnhandledExceptionMode.CatchException);
Copy after login -
Add non-UI thread exception handler:
AppDomain.CurrentDomain.UnhandledException += new UnhandledExceptionEventHandler(CurrentDomain_UnhandledException);
Copy after login
Selective exception handling for debugging:
You can choose to block exception handling during debugging to facilitate error analysis. Wrap the exception handling code with the following conditional:
if (!AppDomain.CurrentDomain.FriendlyName.EndsWith("vshost.exe")) { ... /* 异常处理代码 */ }
Alternatively, you can check if the debugger is attached:
if (!System.Diagnostics.Debugger.IsAttached) { ... /* 异常处理代码 */ }
This ensures that exceptions are only handled when the application is running in production mode.
The above is the detailed content of How to Implement Global Exception Handling in WinForms Applications?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
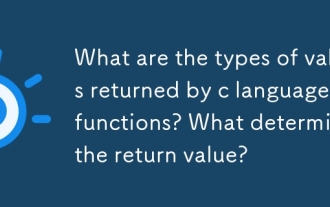
What are the types of values returned by c language functions? What determines the return value?
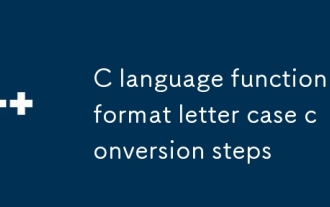
C language function format letter case conversion steps

What are the definitions and calling rules of c language functions and what are the

Where is the return value of the c language function stored in memory?
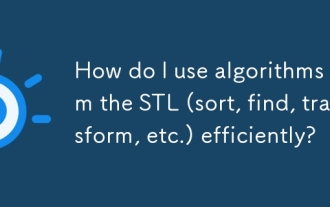
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
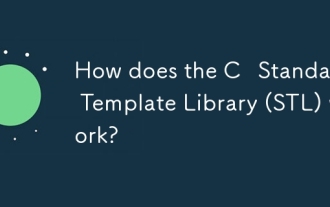
How does the C Standard Template Library (STL) work?
