


How Can Named Pipes Facilitate Inter-Process Communication in a Simple C# Console Application?
A Simple C# Console Application Demonstrating Named Pipes
Named pipes offer a robust method for inter-process communication (IPC) within a single system. This example showcases a basic C# console application where two processes exchange messages using named pipes.
Application Structure
The application comprises two programs: one initiates communication by sending a message to the second, which then responds. Both run concurrently for continuous message exchange.
Code Implementation
The following C# code demonstrates the implementation:
using System; using System.IO; using System.IO.Pipes; using System.Linq; using System.Text; using System.Threading.Tasks; namespace ConsoleAppIPC { class Program { static void Main(string[] args) { // Initiate the server process StartServer(); Task.Delay(1000).Wait(); // Brief delay to ensure server is ready // Client-side connection and communication using (var client = new NamedPipeClientStream("PipesOfPiece")) { client.Connect(); using (var reader = new StreamReader(client)) using (var writer = new StreamWriter(client)) { while (true) { string input = Console.ReadLine(); if (string.IsNullOrEmpty(input)) break; writer.WriteLine(input); writer.Flush(); Console.WriteLine(reader.ReadLine()); } } } } static void StartServer() { Task.Factory.StartNew(() => { using (var server = new NamedPipeServerStream("PipesOfPiece")) { server.WaitForConnection(); using (var reader = new StreamReader(server)) using (var writer = new StreamWriter(server)) { while (true) { string line = reader.ReadLine(); writer.WriteLine(string.Join("", line.Reverse())); writer.Flush(); } } } }); } } }
Operational Flow
The client connects to the named pipe "PipesOfPiece," establishing a connection with the server. Messages are sent via a StreamWriter
and received using a StreamReader
. In this example, the server reverses the received message before sending it back to the client, illustrating a simple communication pattern. The client then displays the reversed message.
The above is the detailed content of How Can Named Pipes Facilitate Inter-Process Communication in a Simple C# Console Application?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










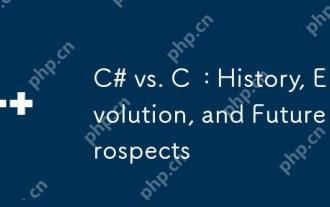
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
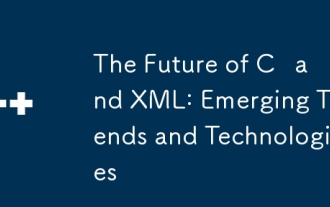
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
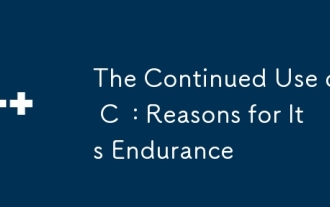
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
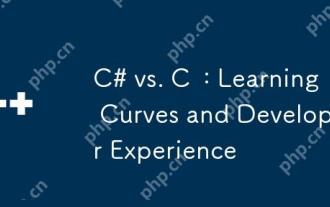
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
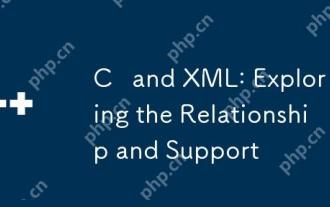
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
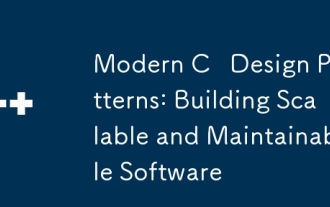
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
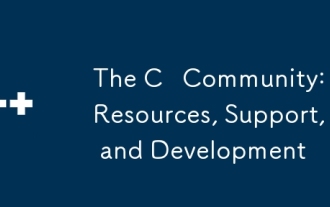
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
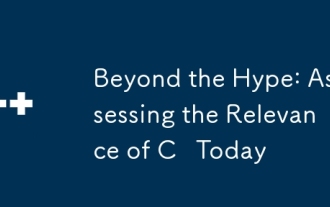
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
