How Can I Easily Generate Ordinal Numbers (1st, 2nd, 3rd, etc.) in C#?
Easily create ordinal numbers in C#
Creating ordinal numbers directly in C# (e.g., 1st, 2nd, 3rd) may not be directly supported by String.Format() or existing functions. However, there are some simple ways to achieve this.
One way is to use a custom function like this:
public static string AddOrdinal(int num) { if (num <= 0) return num.ToString(); // 处理0和负数 string number = num.ToString(); if (num % 100 == 11 || num % 100 == 12 || num % 100 == 13) { return number + "th"; } else { switch (num % 10) { case 1: return number + "st"; case 2: return number + "nd"; case 3: return number + "rd"; default: return number + "th"; } } }
This function receives an integer and checks its modulus to determine the appropriate ordinal suffix ("st", "nd", "rd", or "th"). For example:
Console.WriteLine(AddOrdinal(1)); // 输出:1st Console.WriteLine(AddOrdinal(2)); // 输出:2nd Console.WriteLine(AddOrdinal(3)); // 输出:3rd Console.WriteLine(AddOrdinal(11)); // 输出:11th Console.WriteLine(AddOrdinal(12)); // 输出:12th Console.WriteLine(AddOrdinal(13)); // 输出:13th Console.WriteLine(AddOrdinal(24)); // 输出:24th Console.WriteLine(AddOrdinal(0)); // 输出:0 Console.WriteLine(AddOrdinal(-5)); // 输出:-5
It should be noted that this code has not been internationalized and may need to be adjusted for other languages where the ordinal format may be different. The improved code handles 0 and negative numbers, and handles numbers ending in 11, 12, 13 more accurately.
The above is the detailed content of How Can I Easily Generate Ordinal Numbers (1st, 2nd, 3rd, etc.) in C#?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
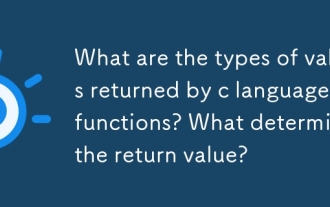
What are the types of values returned by c language functions? What determines the return value?
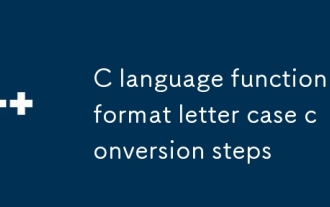
C language function format letter case conversion steps

What are the definitions and calling rules of c language functions and what are the

Where is the return value of the c language function stored in memory?
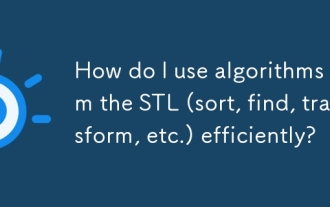
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
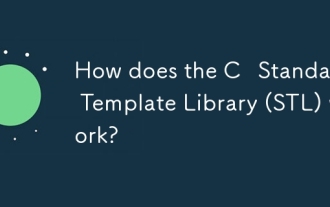
How does the C Standard Template Library (STL) work?
