How to Build a System Tray-Only .NET Windows Forms Application?
Developing a System Tray-Only .NET Windows Forms Application
Standard Windows Forms apps typically occupy space in the main window area. However, some applications only need to reside in the system tray. Here's how to create one:
1. Adjusting Application Startup:
In your Program.cs
file, replace Application.Run(new Form1());
with a call to a custom application context class inheriting from ApplicationContext
. For instance: MyCustomApplicationContext
.
public class MyCustomApplicationContext : ApplicationContext
2. Creating and Configuring the NotifyIcon:
Within your custom application context class, create a NotifyIcon
object. Set its icon, tooltip text, and context menu. Ensure the icon is set to visible.
trayIcon = new NotifyIcon() { // ...icon, tooltip, context menu settings... Visible = true };
3. Implementing Application Exit:
Attach an event handler to your "Exit" menu item. This handler should hide the tray icon and gracefully close the application.
void Exit(object sender, EventArgs e) { trayIcon.Visible = false; Application.Exit(); }
4. Complete Code Example:
Here's a skeletal example demonstrating the process in Program.cs
and MyCustomApplicationContext
:
Program.cs
:
Application.Run(new MyCustomApplicationContext());
MyCustomApplicationContext.cs
:
public class MyCustomApplicationContext : ApplicationContext { private NotifyIcon trayIcon; public MyCustomApplicationContext() { // ...NotifyIcon initialization... } void Exit(object sender, EventArgs e) { // ...Exit handling... } }
By following these steps, your .NET Windows Forms application will operate exclusively within the system tray, offering a subtle and user-friendly interface.
The above is the detailed content of How to Build a System Tray-Only .NET Windows Forms Application?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


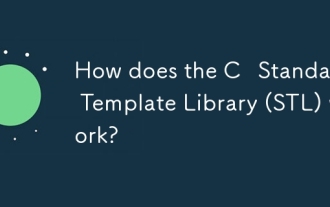
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
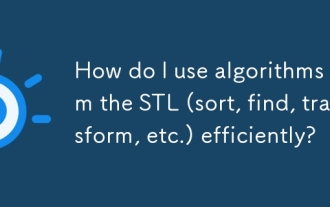
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
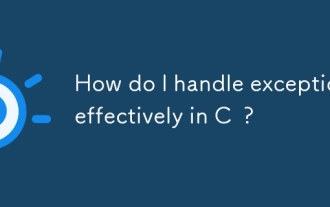
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
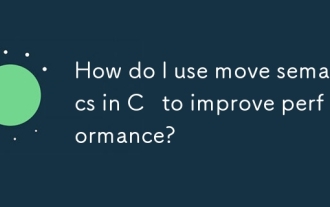
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
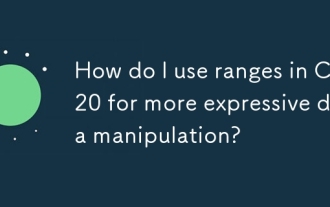
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
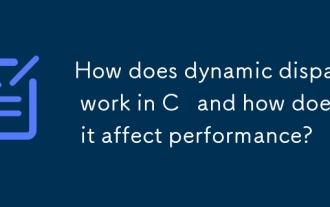
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
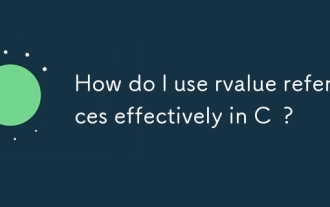
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
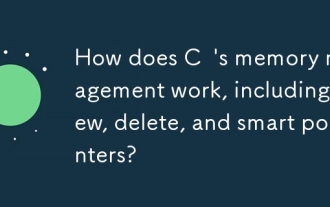
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
