JWT Tokens in Golang: A Developer's Guide to Secure APIs
Introduction
In modern web development, secure and scalable authentication is crucial. JSON Web Tokens (JWT) have become the standard way to achieve this goal. In this blog post, we will explore what JWT is, how it works, and how to implement it in Golang.
What is JWT?
JSON Web Token (JWT) is a compact, URL-safe way of representing claims for securely transmitting claims between two parties. It is commonly used to authenticate and authorize users in APIs and distributed systems.
Structure of JWT
A JWT consists of three parts separated by dots (.):
<code>Header.Payload.Signature</code>
Example:
<code>eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJzdWIiOiIxMjM0NTY3ODkwIiwibmFtZSI6IkpvaG4gRG9lIiwiYWRtaW4iOnRydWUsImlhdCI6MTUxNjIzOTAyMn0.reGQzG3OKdoIMWLDKOZ4TICJit3EW69cQE72E2CfzRE</code>
Each part is:
- Header: Specifies the token type (JWT) and signature algorithm (HS256).
<code>{ "alg": "HS256", "typ": "JWT" }</code>
- Payload: Contains claims (user data such as ID, role or name).
<code>{ "sub": "1234567890", "name": "John Doe", "admin": true, "iat": 1516239022 }</code>
- Signature: Uses a cryptographic signing process to ensure the integrity of the token. Let’s explore this in detail.
How to create a signature:
- Connect the encoded Header and Payload:
<code> base64UrlEncode(header) + "." + base64UrlEncode(payload)</code>Copy after login
- Sign the result:
- Use a cryptographic signature algorithm (e.g., HMACSHA256, RS256) using a secret key or private key.
- Additional signature: The final JWT becomes
<code> header.payload.signature</code>Copy after login
How JWT works
- The client sends login credentials to the server.
- If valid, the server will generate the JWT and return it to the client.
- Client stores JWT (e.g. in localStorage or cookie).
- For every request, the client includes the JWT in the Authorization header: Authorization: Bearer
- The server validates the JWT on every request.
- Recalculate the signature using the received Header and Payload.
- Compare the recalculated signature with the received signature.
Implementing JWT in Golang
Golang developers can leverage the excellent golang-jwt/jwt library to handle JWT. This library provides powerful functionality for creating, signing, and validating JWTs. You can find it here.
However, managing JWTs often requires repetitive tasks such as configuring signing methods, parsing tokens, and validating claims. To simplify this, I wrote a custom package to wrap the functionality of golang-jwt. You can view my package here. Here is an example of how to use my custom JWT package.
package main import ( "context" "fmt" "log" "time" "github.com/golang-jwt/jwt/v5" jwtutil "github.com/kittipat1413/go-common/util/jwt" ) type MyCustomClaims struct { jwt.RegisteredClaims UserID string `json:"uid"` } func main() { ctx := context.Background() signingKey := []byte("super-secret-key") manager, err := jwtutil.NewJWTManager(jwtutil.HS256, signingKey) if err != nil { log.Fatalf("Failed to create JWTManager: %v", err) } // Prepare custom claims claims := &MyCustomClaims{ RegisteredClaims: jwt.RegisteredClaims{ ExpiresAt: jwt.NewNumericDate(time.Now().Add(15 * time.Minute)), Issuer: "example-HS256", Subject: "example-subject", }, UserID: "abc123", } // Create the token tokenStringHS256, err := manager.CreateToken(ctx, claims) if err != nil { log.Fatalf("Failed to create token: %v", err) } fmt.Println("Generated Token:", tokenStringHS256) // Validate the token parsedClaims := &MyCustomClaims{} err = manager.ParseAndValidateToken(ctx, tokenStringHS256, parsedClaims) if err != nil { log.Fatalf("Failed to validate token: %v", err) } fmt.Printf("Token is valid! UserID: %s, Issuer: %s\n", parsedClaims.UserID, parsedClaims.Issuer) }
JWT Best Practices
- Use HTTPS: Always transfer tokens over a secure channel.
- Set expiration time: Include reasonable exp to limit token abuse.
- Protect your keys: Store your keys securely using environment variables or a key manager.
- Avoid using sensitive data in the payload: Include only non-critical information.
- Use refresh token: Pair a JWT with a refresh token to extend the session securely.
Conclusion ?
JWT is a powerful tool for secure, stateless authentication. The signature ensures the integrity and authenticity of the token, making JWT ideal for APIs and distributed systems. Using libraries like jwt-go you can easily implement JWT in your Golang project.
☕ Support my work ☕
If you like my work, please consider buying me a coffee! Your support helps me continue to create valuable content and share knowledge. ☕
The above is the detailed content of JWT Tokens in Golang: A Developer's Guide to Secure APIs. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
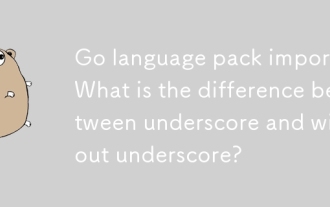
Go language pack import: What is the difference between underscore and without underscore?

How to implement short-term information transfer between pages in the Beego framework?
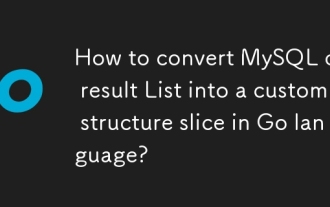
How to convert MySQL query result List into a custom structure slice in Go language?
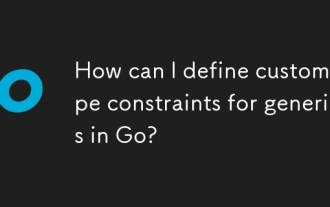
How can I define custom type constraints for generics in Go?
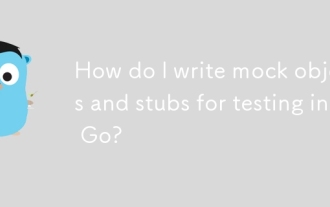
How do I write mock objects and stubs for testing in Go?
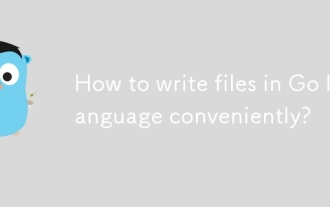
How to write files in Go language conveniently?
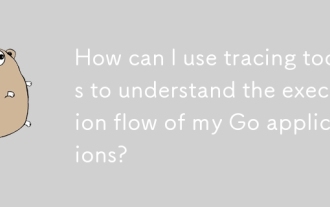
How can I use tracing tools to understand the execution flow of my Go applications?
