Why NestJS Is The New Gold Standard For Node Backend Development
As a Node.js developer who has tried every Node framework under the sun, here’s why I believe NestJS must be the new gold standard for Node.js backend development. At first, the framework seemed like too much—the learning curve was steep, and I found it hard to understand coming from Express. Why all this complexity? But with time, I came to admire every aspect of this framework.
1. Why OOP is the Best for Backend Development
Object-Oriented Programming (OOP) is not just a paradigm; it's a methodology that addresses the core challenges of backend development. At its heart, OOP allows you to model real-world problems in a way that makes systems easier to design, implement, and maintain. NestJS utilizes OOP through its class-based approach, enabling you to organize logic into cohesive, reusable components.
For instance, consider the concept of services in NestJS. A service class encapsulates business logic, promoting separation of concerns. This encapsulation not only makes your codebase easier to navigate but also simplifies testing. Using decorators like @Injectable(), NestJS takes dependency injection a step further, allowing seamless integration of service dependencies without manual wiring.
Code Example
import { Injectable } from '@nestjs/common'; @Injectable() export class UserService { private users = []; createUser(name: string) { const user = { id: Date.now(), name }; this.users.push(user); return user; } getUsers() { return this.users; } }
Moreover, inheritance in OOP allows developers to extend and reuse functionality without duplicating code. Polymorphism, another cornerstone of OOP, lets you define common interfaces for varying implementations. In a NestJS context, this could be seen in abstract classes for database repositories, where specific implementations handle details for MongoDB, PostgreSQL, or other data stores.
So as your codebase grows larger, you can maintain a well-structured codebase within every module.
2. NestJS is Future-Proof
NestJS positions itself as a framework built to withstand the test of time. Its adoption of TypeScript ensures that your code is reliable and maintainable.
NestJS also uses decorators extensively, aligning with modern JavaScript standards such as ES6 and ECMAScript proposals. These decorators, like @Controller(), @Get(), and @Post(), provide declarative syntax that makes code intuitive and reduces boilerplate.
Code Example
import { Injectable } from '@nestjs/common'; @Injectable() export class UserService { private users = []; createUser(name: string) { const user = { id: Date.now(), name }; this.users.push(user); return user; } getUsers() { return this.users; } }
3. Ideal for Microservices and Enterprise Applications
NestJS shines in environments where modularity and scalability are non-negotiable. For microservices, NestJS provides native support for distributed systems using patterns like message brokers and event-driven communication. The framework includes modules for integration with RabbitMQ, Kafka, and Redis, making it easy to design resilient and decoupled systems.
Code Example
import { Controller, Get } from '@nestjs/common'; @Controller('users') export class UserController { @Get() findAll() { return 'This action returns all users'; } }
For enterprise-grade applications, NestJS's modular architecture allows teams to work on isolated modules without stepping on each other’s toes. Features like DynamicModule allow you to configure modules dynamically, simplifying the management of multi-tenant systems or applications with environment-specific configurations.
4. Built-in Support for Everything
NestJS eliminates the need for cobbling together third-party libraries by offering built-in support for a wide array of features. Need WebSocket support for real-time applications? NestJS provides an out-of-the-box module for that. Building a GraphQL API? NestJS's GraphQL module integrates seamlessly with decorators for schema-first or code-first approaches.
Code Example
Integrating JWT authentication using Passport.js:
import { Controller } from '@nestjs/common'; import { MessagePattern } from '@nestjs/microservices'; @Controller() export class AppController { @MessagePattern('notifications') handleNotification(data: any) { console.log('Received notification:', data); } }
For authentication, the @nestjs/passport library integrates Passport.js directly into your project, providing strategies for OAuth, JWT, and local authentication. NestJS also simplifies database interaction with its @nestjs/typeorm and @nestjs/mongoose modules, providing tight integration with popular ORMs like TypeORM and Mongoose.
This all-inclusive approach reduces decision fatigue and ensures consistency across your application, allowing you to focus on solving business problems rather than configuring your stack.
5. Modular Architecture That Scales
NestJS’s modular system is a game-changer for large-scale applications. By encapsulating features into dedicated modules, it allows developers to maintain clean boundaries between different parts of the application. Each module in NestJS acts as a self-contained unit, bundling together controllers, services, and other components.
Code Example
Creating a user module:
import { Injectable } from '@nestjs/common'; import { PassportStrategy } from '@nestjs/passport'; import { Strategy, ExtractJwt } from 'passport-jwt'; @Injectable() export class JwtStrategy extends PassportStrategy(Strategy) { constructor() { super({ jwtFromRequest: ExtractJwt.fromAuthHeaderAsBearerToken(), secretOrKey: 'secretKey', }); } validate(payload: any) { return { userId: payload.sub, username: payload.username }; } }
The modular design allows you to separate concerns and easily scale your application by adding new modules.
NestJS also supports lazy loading of modules, a crucial feature for microservices or applications with large, complex dependencies. This ensures that your application starts faster and consumes fewer resources.
6. Very Hard to Mess Up in NestJS
NestJS offers guardrails that make it difficult to write bad code. Its opinionated structure enforces best practices, such as the separation of concerns, dependency injection, and modular design. The framework's CLI (@nestjs/cli) helps scaffold components with a consistent folder structure, eliminating the guesswork in organizing your codebase.
Code Example
Using guards for role-based access control:
import { Injectable } from '@nestjs/common'; @Injectable() export class UserService { private users = []; createUser(name: string) { const user = { id: Date.now(), name }; this.users.push(user); return user; } getUsers() { return this.users; } }
Additionally, NestJS makes full use of TypeScript to catch errors during development, reducing runtime bugs. Features like guards (@CanActivate), interceptors (@Interceptor()), and pipes (@PipeTransform) provide clear patterns for handling concerns like validation, transformation, and access control. This layered approach ensures that even junior developers can contribute effectively while adhering to established conventions.
Even error handling is simplified. By extending the built-in HttpException, you can create custom exceptions with ease, ensuring your APIs remain consistent and predictable. NestJS takes care of the rest, including sending the appropriate HTTP response codes and error messages.
Final Thoughts
NestJS isn’t just another Node.js framework; it’s a paradigm shift in backend development. It’s an ideal choice, offering everything you need to succeed. From my experience, I believe this framework strikes the perfect balance between rapid delivery and long-term maintainability. It represents the ultimate evolution of Node.js backend development, setting a new standard for scalability and efficiency.
For more in-depth insights, check out the book Scalable Application Development with NestJS, available here.
The above is the detailed content of Why NestJS Is The New Gold Standard For Node Backend Development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










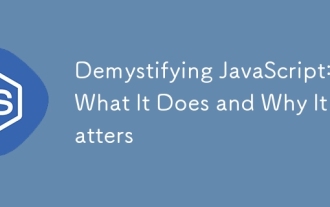
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
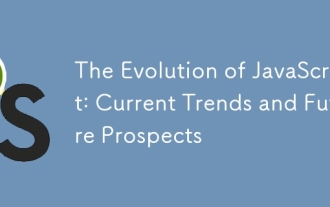
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
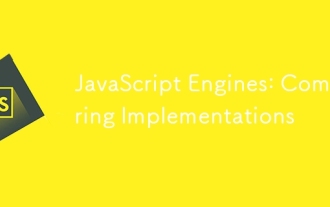
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
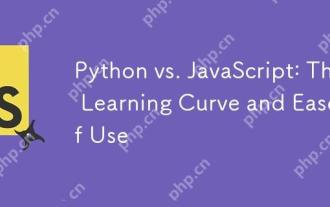
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
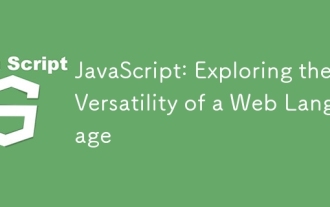
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
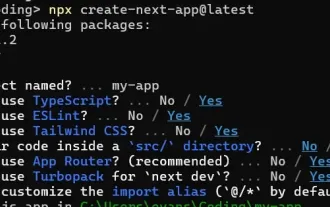
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
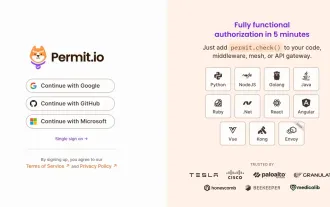
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
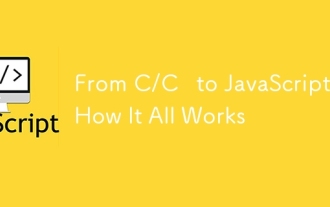
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
