How to Transpose a SQL Table with Multiple Columns?
Transpose a SQL table with multiple columns
Question:
You need to transpose a SQL table with multiple columns, for example:
<code>Day A B --------- Mon 1 2 Tue 3 4 Wed 5 6 Thu 7 8 Fri 9 0</code>
Transpose to the following format:
<code>Value Mon Tue Wed Thu Fri -------------------------- A 1 3 5 7 9 B 2 4 6 8 0</code>
Solution:
To transpose a table with multiple columns, you can use the UNPIVOT and PIVOT functions together.
- UNPIVOT: Convert multiple columns (A, B) into rows and add a column for column names:
select day, col, value from yourtable unpivot ( value for col in (A, B) ) unpiv
- PIVOT: Convert "day" value to column and aggregate "value" column:
select * from ( select day, col, value from yourtable unpivot ( value for col in (A, B) ) unpiv ) src pivot ( max(value) for day in (Mon, Tue, Wed, Thu, Fri) ) piv
This will generate the required transposed table.
Additional notes:
- If your SQL Server version is 2008 or higher, you can use CROSS APPLY and VALUES instead of the UNPIVOT function to transpose the data:
select * from ( select day, col, value from yourtable cross apply ( values ('A', ACalls), ('B', BCalls) ) c (col, value) ) src pivot ( max(value) for day in (Mon, Tue, Wed, Thu, Fri) ) piv
- To perform the transpose operation on your specific query, you can use code similar to the following structure:
select * from ( select LEFT(datename(dw,datetime),3) as DateWeek, col, value from DataTable cross apply ( values ('A', ACalls), ('B', BCalls) ) c (col, value) ) src pivot ( sum(value) for dateweek in (Mon, Tue, Wed, Thu, Fri) ) piv
The above is the detailed content of How to Transpose a SQL Table with Multiple Columns?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










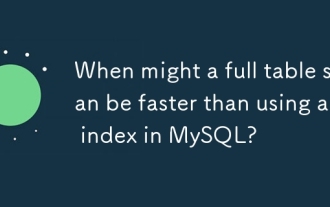
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
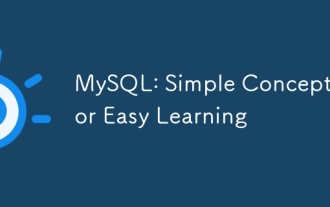
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
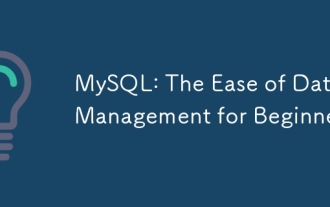
MySQL is suitable for beginners because it is simple to install, powerful and easy to manage data. 1. Simple installation and configuration, suitable for a variety of operating systems. 2. Support basic operations such as creating databases and tables, inserting, querying, updating and deleting data. 3. Provide advanced functions such as JOIN operations and subqueries. 4. Performance can be improved through indexing, query optimization and table partitioning. 5. Support backup, recovery and security measures to ensure data security and consistency.
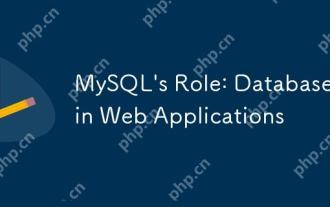
The main role of MySQL in web applications is to store and manage data. 1.MySQL efficiently processes user information, product catalogs, transaction records and other data. 2. Through SQL query, developers can extract information from the database to generate dynamic content. 3.MySQL works based on the client-server model to ensure acceptable query speed.
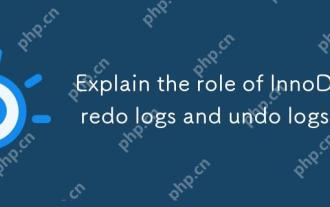
InnoDB uses redologs and undologs to ensure data consistency and reliability. 1.redologs record data page modification to ensure crash recovery and transaction persistence. 2.undologs records the original data value and supports transaction rollback and MVCC.
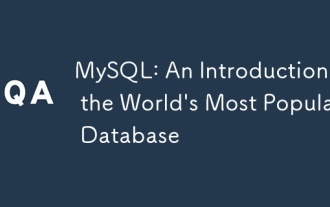
MySQL is an open source relational database management system, mainly used to store and retrieve data quickly and reliably. Its working principle includes client requests, query resolution, execution of queries and return results. Examples of usage include creating tables, inserting and querying data, and advanced features such as JOIN operations. Common errors involve SQL syntax, data types, and permissions, and optimization suggestions include the use of indexes, optimized queries, and partitioning of tables.
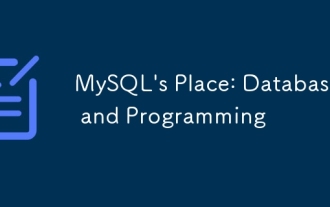
MySQL's position in databases and programming is very important. It is an open source relational database management system that is widely used in various application scenarios. 1) MySQL provides efficient data storage, organization and retrieval functions, supporting Web, mobile and enterprise-level systems. 2) It uses a client-server architecture, supports multiple storage engines and index optimization. 3) Basic usages include creating tables and inserting data, and advanced usages involve multi-table JOINs and complex queries. 4) Frequently asked questions such as SQL syntax errors and performance issues can be debugged through the EXPLAIN command and slow query log. 5) Performance optimization methods include rational use of indexes, optimized query and use of caches. Best practices include using transactions and PreparedStatemen
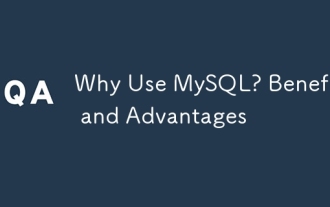
MySQL is chosen for its performance, reliability, ease of use, and community support. 1.MySQL provides efficient data storage and retrieval functions, supporting multiple data types and advanced query operations. 2. Adopt client-server architecture and multiple storage engines to support transaction and query optimization. 3. Easy to use, supports a variety of operating systems and programming languages. 4. Have strong community support and provide rich resources and solutions.
