How to Load Remote Components in Vue 3
Background
I recently received a requirement in a Vue 3 low-code project to load remote components. These remote components have unpredictable names and are stored in a database. I need to fetch all component data through an API to determine what components are available. After research, I found two viable solutions to meet this requirement.
HTML File UMD Components
This is the simplest solution to implement. We just need to package the component in UMD format and use it directly in the HTML file.
<div> <p>However, this solution is not suitable for large projects due to its low efficiency.</p> <h2> Vue 3 Project + ESM/UMD Components </h2> <p>This is the solution I implemented in my low-code project. During my research, I encountered and solved two main problems. Here's how it works:</p> <h3> Problem 1: Relative References </h3> <p>Since our project doesn't need to be compatible with IE, we can package the source code in ESM format. For example:<br> </p> <pre class="brush:php;toolbar:false">import { reactive } from 'vue' // other code...
then use in project:
const { default: TestInput } = await import('http://localhost/component/input/0.1.0/bundle.mjs')
When loading the remote TestInput component as shown above, it causes a "Relative references must start with either '/', './', or '../'" error. This is because browsers don't support directly using import { reactive } from 'vue' - we need to change 'vue' to https://..../vue.js or './vue.js'. Usually, we don't need to worry about this as our build tools handle it automatically.
Problem 2: Different Vue 3 Contexts
My first attempt to solve the first problem was to package the component with all its dependencies. While this removed all import statements, it unfortunately didn't work. This is because the Vue 3 context in our project and the Vue 3 context from node_modules are incompatible - they need to share the same context to work properly.
Even though all Vue 3 method names are the same across different contexts, their variables are not. This prevents remote components from loading normally.
Solution
To solve these problems:
- We can replace import { reactive } from 'vue' with const { reactive } = Vue to avoid the relative references error.
- We can import the entire Vue 3 instance in main.js instead of packaging it with the source code. This ensures our project and remote components use the same Vue context.
To handle the code transformation, I created a rollup plugin called rollup-plugin-import-to-const (supporting both vite and rollup). It automatically transforms code from import { reactive } from 'vue' to const { reactive } = Vue. With these solutions in place, we can load remote components in our project:
const { default: TestInput } = await import('http://localhost/component/input/0.1.0/bundle.mjs')
Actually, we can load components in any format (ESM/UMD/CJS, etc.) as long as we solve these two problems.
Summary
Loading remote components isn't limited to these two solutions. For example, we can also use vue3-sfc-loader or webpack5 Module Federation. The choice depends on your project's specific requirements.
Generally, loading remote components is most commonly used in low-code platforms.
The above is the detailed content of How to Load Remote Components in Vue 3. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










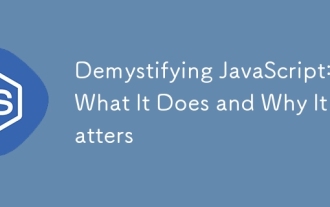
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
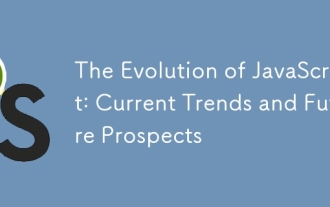
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
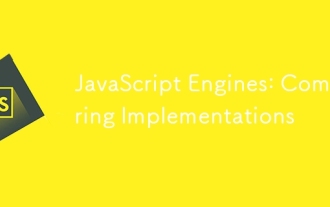
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
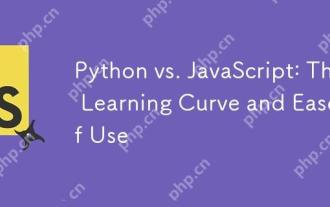
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
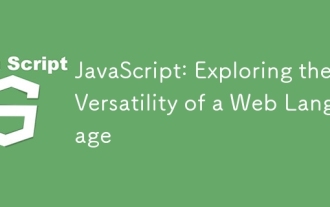
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
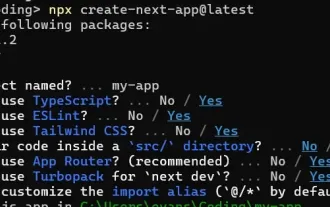
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
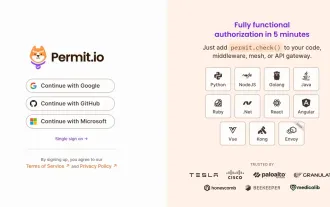
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
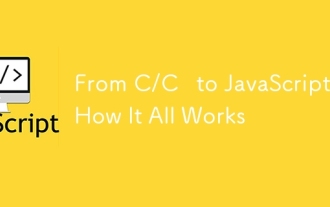
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
