Go Basics: Syntax and Structure
Welcome to part two of our Go Programming Tutorial Series, designed to build a solid Go (Golang) foundation. This article, focusing on Go Fundamentals: Syntax and Structure, covers everything from your first "Hello, World!" program to variables, constants, data types, and more. Whether you're a novice or seeking to refine your skills, this guide provides the knowledge for writing efficient, clean Go code.
Upon completion, you will be able to:
- Create your first Go program: Hello, World!
- Understand the
main
package andmain
function. - Work with variables, constants, and data types.
- Grasp the concept of zero values in Go.
- Utilize type inference and type conversion.
Let's begin!
Core Concepts
1. Your First Go Program: Hello, World!
Every programming journey starts with "Hello, World!". In Go:
package main import "fmt" func main() { fmt.Println("Hello, World!") }
Explanation:
package main
: Every Go program begins with a package declaration.main
signifies an executable program.import "fmt"
: Imports thefmt
package for console output (likePrintln
).func main()
: The program's entry point. Execution starts here.fmt.Println("Hello, World!")
: Prints "Hello, World!" to the console.
2. Understanding the main
Package and main
Function
- The
main
package is essential for creating executable Go programs. Without it, your code won't run independently. - The
main
function is mandatory within themain
package; it's the program's starting point.
3. Basic Syntax: Variables, Constants, and Data Types
Go is statically typed—you must specify a variable's data type. However, Go also supports type inference for concise code.
Variables
Variables are declared using var
:
var name string = "Go Programmer" var age int = 30
Shorthand (within functions):
name := "Go Programmer" age := 30
Constants
Constants are immutable values, declared with const
:
const pi float64 = 3.14159
Data Types
Go offers various built-in data types:
- Basic types:
int
,float64
,string
,bool
. Example:
var age int = 35 var price float64 = 29.99 var name string = "Bob" var isActive bool = true
- Composite types:
array
,slice
,struct
,map
. Example:
// Array var scores [3]int = [3]int{95, 80, 92} // Slice var grades []float64 = []float64{88.2, 91.5, 78.9} // Struct type Person struct { FirstName string LastName string Age int } var person = Person{"Jane", "Doe", 28} // Map var capitals map[string]string = map[string]string{ "France": "Paris", "Italy": "Rome", }
4. Zero Values in Go
Variables without explicit initialization receive their zero value:
- 0 for numeric types.
false
for booleans.""
(empty string) for strings.nil
for pointers, slices, maps, and channels.
Example:
package main import "fmt" func main() { fmt.Println("Hello, World!") }
5. Type Inference and Type Conversion
Go infers variable types from assigned values:
var name string = "Go Programmer" var age int = 30
Type conversion requires explicit casting:
name := "Go Programmer" age := 30
Practical Example
Let's create a program demonstrating variables, constants, data types, zero values, type inference, and type conversion:
const pi float64 = 3.14159
(The code would be identical to the example in the original text, with potentially slightly different variable names to maintain clarity and avoid repetition.)
Output (Similar to the original output)
(The output would be similar to the original example, reflecting the values and types of the variables.)
Explanation:
(The explanation would be the same as in the original, explaining each section of the code.)
Best Practices
- Descriptive Variable Names: Use clear, meaningful names.
var age int = 35 var price float64 = 29.99 var name string = "Bob" var isActive bool = true
- Type Inference: Use
:=
when the type is obvious.
// Array var scores [3]int = [3]int{95, 80, 92} // Slice var grades []float64 = []float64{88.2, 91.5, 78.9} // Struct type Person struct { FirstName string LastName string Age int } var person = Person{"Jane", "Doe", 28} // Map var capitals map[string]string = map[string]string{ "France": "Paris", "Italy": "Rome", }
- Avoid Unnecessary Type Conversions: Convert only when needed.
- Explicit Variable Initialization: Initialize variables clearly.
-
Clean
main
Function: Keepmain
concise; delegate logic to other functions.
Conclusion
This article covered Go's basic syntax and structure, including the "Hello, World!" program, the main
package and function, variables, constants, data types, zero values, type inference, and type conversion. Mastering these fundamentals is crucial for your Go programming journey.
Experiment with the example program or create your own to solidify your understanding.
Call to Action
This article is part of our ongoing Go Tutorial Series. Look for the next tutorial on Control Structures in Go!
Happy coding! ?
The above is the detailed content of Go Basics: Syntax and Structure. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


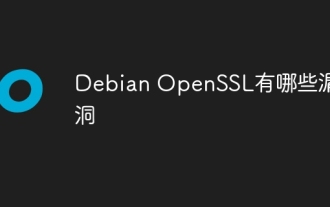
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
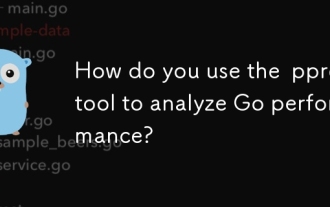
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
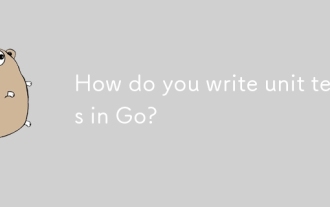
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
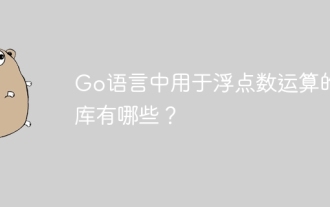
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
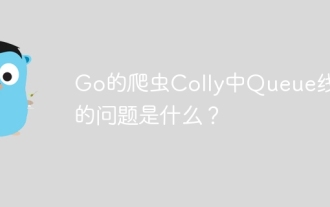
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
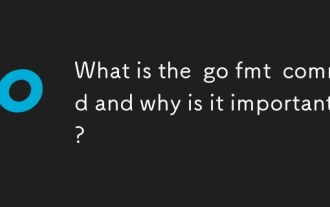
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
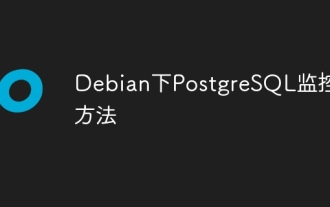
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
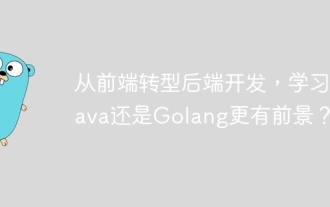
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
