How Can I Securely Parameterize SQL Queries with Dynamic Table Names?
Parameterized SQL queries using dynamic table names
Introduction
In SQL programming, passing variable table names into stored procedures is a common challenge. This article explores the limitations of parameterized queries and provides a reliable solution that is both safe and flexible.
Question
Traditionally, SQL statements are constructed on the client side and passed to the database as strings. This approach is vulnerable to SQL injection attacks as user input can be manipulated to execute malicious commands.
Parameterized query
To mitigate the risk of SQL injection, parameterized queries are introduced. These queries use placeholders in place of user input and then bind the actual values when executed. This prevents malicious code from being injected into the query.
However, there are challenges with parameterized queries when table names are variables. Dynamic SQL (generating query text at runtime) is often used to solve this problem. However, this approach can result in complex and error-prone code.
Secure and flexible solution
A safer and more elegant solution is to use stored procedures with dynamic SQL. The stored procedure takes user input as a parameter and uses it to look up the actual table name from a secure source (such as a database table or XML file).
The following example illustrates this approach:
CREATE PROC spCountAnyTableRows( @PassedTableName AS NVarchar(255) ) AS -- 安全地计算任何非系统表的行数 BEGIN DECLARE @ActualTableName AS NVarchar(255) SELECT @ActualTableName = QUOTENAME( TABLE_NAME ) FROM INFORMATION_SCHEMA.TABLES WHERE TABLE_NAME = @PassedTableName DECLARE @sql AS NVARCHAR(MAX) SELECT @sql = 'SELECT COUNT(*) FROM ' + @ActualTableName + ';' EXEC(@SQL) END
This stored procedure takes the passed table name, looks up the actual table name from the metadata table INFORMATION_SCHEMA.TABLES, and then executes a dynamic SQL query to count the number of rows in the actual table.
Safety Precautions
Using this approach provides several security advantages:
- SQL injection protection: The table name passed is only used for lookups and is not used directly in the executed query.
- Principle of Least Privilege: Limit lookup queries to specific metadata tables or XML files, limiting the potential damage of malicious attacks.
Other notes
- A similar method (INFORMATION_SCHEMA.COLUMNS) can be used to handle dynamic column names.
- Parameterized SQL queries can also be used with dynamic table names, but stored procedures provide a more manageable and secure solution.
- Refactoring table names into a single table with a "name" column is not possible in all cases.
The above is the detailed content of How Can I Securely Parameterize SQL Queries with Dynamic Table Names?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










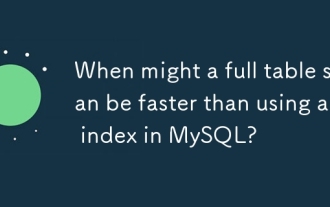
Full table scanning may be faster in MySQL than using indexes. Specific cases include: 1) the data volume is small; 2) when the query returns a large amount of data; 3) when the index column is not highly selective; 4) when the complex query. By analyzing query plans, optimizing indexes, avoiding over-index and regularly maintaining tables, you can make the best choices in practical applications.
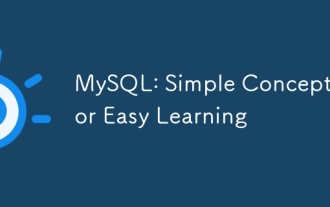
MySQL is an open source relational database management system. 1) Create database and tables: Use the CREATEDATABASE and CREATETABLE commands. 2) Basic operations: INSERT, UPDATE, DELETE and SELECT. 3) Advanced operations: JOIN, subquery and transaction processing. 4) Debugging skills: Check syntax, data type and permissions. 5) Optimization suggestions: Use indexes, avoid SELECT* and use transactions.
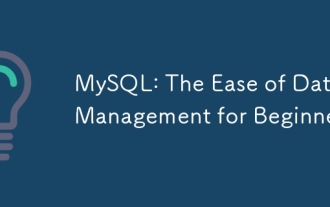
MySQL is suitable for beginners because it is simple to install, powerful and easy to manage data. 1. Simple installation and configuration, suitable for a variety of operating systems. 2. Support basic operations such as creating databases and tables, inserting, querying, updating and deleting data. 3. Provide advanced functions such as JOIN operations and subqueries. 4. Performance can be improved through indexing, query optimization and table partitioning. 5. Support backup, recovery and security measures to ensure data security and consistency.
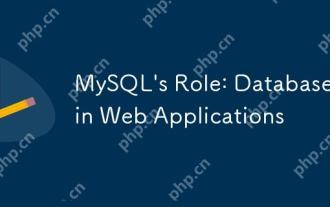
The main role of MySQL in web applications is to store and manage data. 1.MySQL efficiently processes user information, product catalogs, transaction records and other data. 2. Through SQL query, developers can extract information from the database to generate dynamic content. 3.MySQL works based on the client-server model to ensure acceptable query speed.
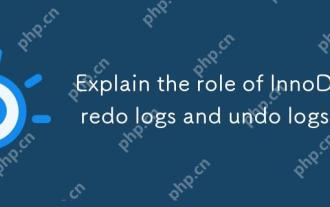
InnoDB uses redologs and undologs to ensure data consistency and reliability. 1.redologs record data page modification to ensure crash recovery and transaction persistence. 2.undologs records the original data value and supports transaction rollback and MVCC.
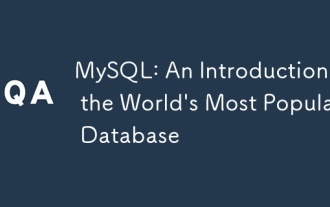
MySQL is an open source relational database management system, mainly used to store and retrieve data quickly and reliably. Its working principle includes client requests, query resolution, execution of queries and return results. Examples of usage include creating tables, inserting and querying data, and advanced features such as JOIN operations. Common errors involve SQL syntax, data types, and permissions, and optimization suggestions include the use of indexes, optimized queries, and partitioning of tables.
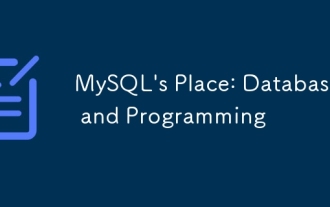
MySQL's position in databases and programming is very important. It is an open source relational database management system that is widely used in various application scenarios. 1) MySQL provides efficient data storage, organization and retrieval functions, supporting Web, mobile and enterprise-level systems. 2) It uses a client-server architecture, supports multiple storage engines and index optimization. 3) Basic usages include creating tables and inserting data, and advanced usages involve multi-table JOINs and complex queries. 4) Frequently asked questions such as SQL syntax errors and performance issues can be debugged through the EXPLAIN command and slow query log. 5) Performance optimization methods include rational use of indexes, optimized query and use of caches. Best practices include using transactions and PreparedStatemen
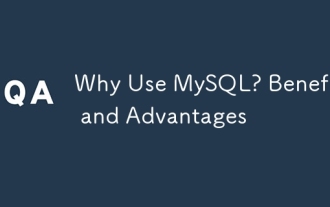
MySQL is chosen for its performance, reliability, ease of use, and community support. 1.MySQL provides efficient data storage and retrieval functions, supporting multiple data types and advanced query operations. 2. Adopt client-server architecture and multiple storage engines to support transaction and query optimization. 3. Easy to use, supports a variety of operating systems and programming languages. 4. Have strong community support and provide rich resources and solutions.
